Express builds query server
This article mainly introduces how to use express to build a simple query server. Now I share it with you and give it as a reference.
This article introduces the method of using express to build a simple query server and shares it with everyone. The details are as follows:
The technology stack used includes express and mysql.
projects Structure:
service --node_modules --app.js --query.js
app.js supports calling services and uses body-parser to process requests.
query.js implements the functions of linking to the database and querying the database.
app The .js code is as follows:
var express = require('express'); var query = require('./query') var bodyParser = require('body-parser'); var cookieParser = require('cookie-parser'); var app = express(); app.use(bodyParser.urlencoded({ extended: false }))//返回的对象是一个键值对,当extended为false的时候,键值对中的值就为'String'或'Array'形式,为true的时候,则可为任何数据类型。 app.use(bodyParser.json()) //跨域支持 app.all('*', function(req, res, next) { res.header("Access-Control-Allow-Origin", "*"); res.header('Access-Control-Allow-Methods', 'PUT, GET, POST, DELETE, OPTIONS'); res.header("Access-Control-Allow-Headers", "X-Requested-With"); res.header('Access-Control-Allow-Headers', 'Content-Type'); next(); }); //登录 app.post('/login',(req,res)=>{ var opts = req.body; query(" SELECT *FROM `v_users` WHERE userAcount = ?",opts.userName).then((result)=>{ var response = result[0]; if(opts.password !== response.u_password){ return res.send({ errorCode:'404', errorMsg:'登录密码错误' }) } //模拟生成loginToken var loginToken = response.userAcount + Math.random()*Math.pow(10,16) res.send({ loginToken:loginToken }) }) }) var server = app.listen(3000,()=>{ console.log('success') })
query.js code is as follows:
(function() { var mysql = require('mysql'); // var session = require('cookie-session'); var query = (sql,key) => { var connection = mysql.createConnection({ host: 'localhost', user: 'root', password: 'root123', database: 'm_users' }); connection.connect() var promise = new Promise((resolve,reject)=>{ connection.query(sql,[key], function(error, results, fields) { if(error){ reject(error) }else{ resolve(results); } }); connection.end(); }); return promise; } module.exports = query; })()
Practice summary:
1. Entry-level usage of express, as well as body-parser and mysql plug-ins usage.
2. Try to use Inspector to debug the node program and implement debugger, by the way. Personally, I am more accustomed to using gulp for debugging.
3. When the client uses post to call the interface, it must distinguish Content- The difference between Type:
Content-Type:application/json;charset=UTF-8 parameter is placed in requestPayload
Content-Type: does not set or application/x-www-form-urlencoded parameter I put it on Form Data
and compiled it for everyone. I hope it will be helpful to everyone in the future.
Related articles:
Use cheerio to make a simple web crawler in Node.js (detailed tutorial)
How to do it in vue Implement the parent component to pass multiple data to the child component
How to use Native in React to implement a custom pull-down refresh pull-up loaded list
The above is the detailed content of Express builds query server. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
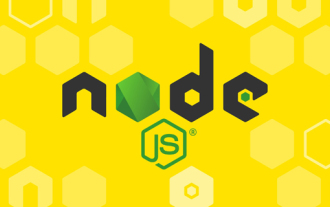
How to handle file upload? The following article will introduce to you how to use express to handle file uploads in the node project. I hope it will be helpful to you!
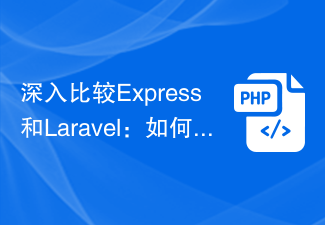
In-depth comparison of Express and Laravel: How to choose the best framework? When choosing a back-end framework suitable for your project, Express and Laravel are undoubtedly two popular choices among developers. Express is a lightweight framework based on Node.js, while Laravel is a popular framework based on PHP. This article will provide an in-depth comparison of the advantages and disadvantages of these two frameworks and provide specific code examples to help developers choose the framework that best suits their needs. Performance and scalabilityExpr
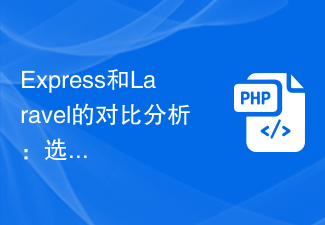
Express and Laravel are two very popular web frameworks, representing the excellent frameworks of the two major development languages of JavaScript and PHP respectively. This article will conduct a comparative analysis of these two frameworks to help developers choose a framework that is more suitable for their project needs. 1. Framework Introduction Express is a web application framework based on the Node.js platform. It provides a series of powerful functions and tools that enable developers to quickly build high-performance web applications. Express
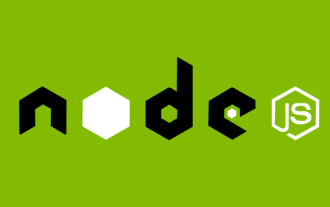
How does node+express operate cookies? The following article will introduce to you how to use node to operate cookies. I hope it will be helpful to you!
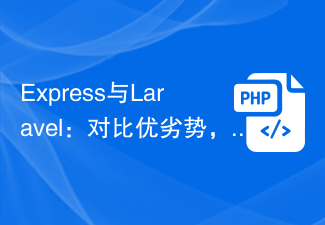
Express vs. Laravel: Comparing the advantages and disadvantages, which one will you choose? In the field of web development, Express and Laravel are two frameworks that have attracted much attention. Express is a flexible and lightweight web application framework based on Node.js, while Laravel is an elegant and feature-rich web development framework based on PHP. This article will compare the advantages and disadvantages of Express and Laravel in terms of functionality, ease of use, scalability, and community support, and combine
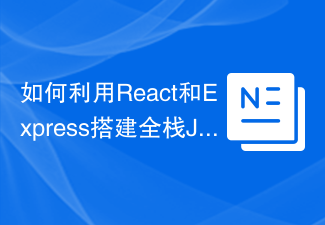
How to use React and Express to build a full-stack JavaScript application Introduction: React and Express are currently very popular JavaScript frameworks. They are used to build front-end and back-end applications respectively. This article will introduce how to use React and Express to build a full-stack JavaScript application. We will explain step by step how to build a simple TodoList application and provide specific code examples. 1. Preparation before starting
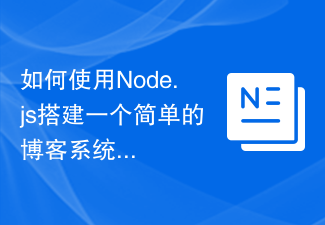
How to use Node.js to build a simple blog system Node.js is a JavaScript runtime environment based on the ChromeV8 engine, which can make JavaScript run more efficiently. With the help of Node.js, we can build powerful server-side applications using JavaScript, including blogging systems. This article will introduce you to how to use Node.js to build a simple blog system and provide you with specific code examples. Press
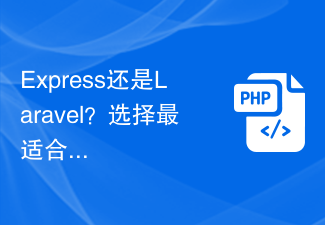
When it comes to choosing a backend framework, both Express and Laravel are very popular choices. Express is a web application development framework based on Node.js, while Laravel is a web application development framework based on PHP. Both have their own advantages, and choosing the framework that best suits you requires considering many factors. The strengths of the Express framework are its flexibility and easy learning curve. The core idea of Express is "small enough and flexible enough", and it provides a large number of middleware
