


How to learn the process and child_process modules in node (detailed tutorial)
This article mainly introduces the node process and child_process module study notes. Now I share them with you and give them a reference.
After struggling with the process for a week, I finally understood the obscure and difficult document, and am ready to share my understanding with everyone. I also hope that everyone can point out some opinions
Process The concept of
#In Node.js every application is an instance object of the process class.
Use the process object to represent the application. This is a global object through which you can obtain the properties of the Node.jsy application and the user and environment running the program. methods and events.
Several important attributes in the process
stdin standard input readable stream
stdout standard input writable stream
stderr standard error output stream
argv terminal input parameter array
env operating system environment information
pid application process id
stdin and stdout
process.stdin.on('data', (chunk) => { process.stdout.write('进程接收到数据' + chunk) })
Run result
argv
console.log(process.env)
console.log(process.env.NODE_ENV) //develop
- process.memoryUsage() View memory usage information
- process.nextTick() Current The callback function is executed after the eventloop is executed
- process.chdir() The chdir method is used to modify the current working directory used in the Node.js application
- process.cwd() The current working directory of the process
- process.kill() Kill the process
- process.uncaughtException() When the application The uncaughtException event of the process object is triggered when an uncaught exception is thrown
say() //方法不存在 process.on('uncaughtException',function(err){ console.log('捕获到一个未被处理的错误:',err); });
Copy after login
The child process is the focus of today, I also have some things that I don’t quite understand, and I hope I can communicate with you more
The background of child_processIn Node.js, there is only one thread to perform all operations. If a certain When an operation consumes a lot of CPU resources, subsequent operations need to wait.
In Node.js, a child_process module is provided, through which multiple child processes can be started, memory space can be shared between multiple child processes, and information exchange can be achieved through mutual communication of child processes.
The child_process module gives node the ability to create child processes at will. The official document of node gives four methods for the child_proces module. Mapping to the operating system actually creates child processes. But for developers only, the APIs of these methods are a little different
child_process.exec(command[, options][, callback]) starts the
child process to execute the shell command, which can be done through the callback parameter Get the script shell execution result
child_process.execfile(file[, args][, options][, callback])
child_process.spawn(command[, args][, options]) only executes a shell command and does not need to obtain the execution results
child_process.fork(modulePath[, args][, options] ]) You can use node
to execute the .js file, and there is no need to obtain the execution results. The child process coming out of fork must be the node processSyntax: child_process.spawn(command, [args], [options])
- command Parameters that must be specified, specify the command to be executed
- args array, which stores all the parameters required to run the command
- The options parameter is an object, used to specify the options used when starting the child process
const { spawn } = require('child_process') const path = require('path') let child1 = spawn('node', ['test1.js', 'yanyongchao'], { stdio: ['pipe', 'pipe', 'pipe'], // 三个元素数组 下面会详解 cwd: __dirname, 子进程工作目录 env: process.env, 环境变量 detached: true // 如果为true,当父进程不存在时也可以独立存在 })
Copy after loginIn fact, the above is easy to understand except for the sdtio array. Let’s analyze stdio
stdio is an array used to set standard input, standard output, and error output. Personal understanding
pipe: Establish a pipe between the parent process and the child process
Main process code
const path = require('path') const { spawn } = require('child_process') let p = spawn('node', ['childs_t.js'], { cwd: path.join(__dirname, 'childs'), stdio: ['pipe', 'pipe', process.stderr] }) p.stdout.on('data', (data) => { console.log(data.toString()) }) // 这里用stdout原因: 子进程的数据流与常规理解的数据流方向相反, // stdin:写入流,stdout、stderr:读取流。
Child process code
process.stdout.write('asd')
If you put it in stdio A stream, process.stdout, process.stdin
Main process code
const { spawn } = require('child_process') const path = require('path') // 如果放的是一个流,则意味着父进程和子进程共享一个流 const p = spawn('node', ['child_t.js'], { cwd: path.join(__dirname, 'childs'), stdio: [process.stdin, process.stdout, process.stderr] })
Sub process code
process.stdout.write('asd') //控制台会输出asd
Main Process code
const path = require('path') const { spawn } = require('child_process') let p = spawn('node', ['child_t.js'], { cwd: path.join(__dirname, 'childs'), stdio: ['ipc', 'pipe', 'pipe'] }) p.on('message', (msg) => { console.log(msg) }) p.send('hello chhild_process')
Child process code
process.on('message', (msg) => { process.send('子进程' + msg) }) // child.send(message,[sendHandle]);//在父进程中向子进程发送消息 // process.send(message,[sendHandle]);//在子进程中向主进程发送消息
const { spawn } = require('child_process')
const fs = require('fs')
const path = require('path')
let out = fs.openSync(path.join(__dirname, 'childs/msg.txt'), 'w', 0o666)
let p = spawn('node', ['test4.js'], {
detached: true, //保证父进程结束,子进程仍然可以运行
stdio: 'ignore',
cwd: path.join(__dirname, 'childs')
})
p.unref()
p.on('close', function() {
console.log('子进程关闭')
})
p.on('exit', function() {
console.log('子进程退出')
})
p.on('error', function(err) {
console.log('子进程1开启失败' + err)
})
- Spawn a new Node.js process and call a specified module by establishing an IPC communication channel, which allows the parent process and the child process to send information to each other
- The fork method returns an implicitly created ChildProcess object representing the child process
- After the input/output operation of the child process is completed, the child process will not automatically exit and must Explicitly exit using the process.exit() method
- Child process code
const { fork } = require('child_process') const path = require('path') let child = fork(path.join(__dirname, 'childs/fork1.js')) child.on('message', (data) => { console.log('父进程接收到消息' + data) }) child.send('hello fork') child.on('error', (err) => { console.error(err) })
Child process code
process.on('message', (m, setHandle) => { console.log('子进程接收到消息' + m) process.send(m) //sendHandle是一个 net.Socket 或 net.Server 对象 })
// exec同步执行一个shell命令
let { exec } = require('child_process')
let path = require('path')
// 用于使用shell执行命令, 同步方法
let p1 = exec('node exec.js a b c', {cwd: path.join(__dirname, 'childs')}, function(err, stdout, stderr) {
console.log(stdout)
})
上面是我整理给大家的,希望今后会对大家有帮助。 相关文章: The above is the detailed content of How to learn the process and child_process modules in node (detailed tutorial). For more information, please follow other related articles on the PHP Chinese website!let { execFile } = require('child_process')
let path = require('path')
let p1 = execFile('node', ['exec.js', 'a', 'b', 'c'], {
cwd: path.join(__dirname, 'childs')
}, function(err, stdout, stderr) {
console.log(stdout)
})

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
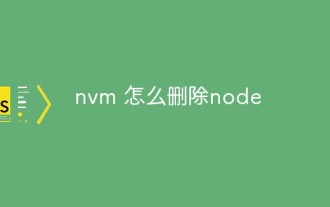
How to delete node with nvm: 1. Download "nvm-setup.zip" and install it on the C drive; 2. Configure environment variables and check the version number through the "nvm -v" command; 3. Use the "nvm install" command Install node; 4. Delete the installed node through the "nvm uninstall" command.
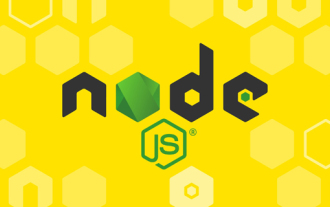
How to handle file upload? The following article will introduce to you how to use express to handle file uploads in the node project. I hope it will be helpful to you!
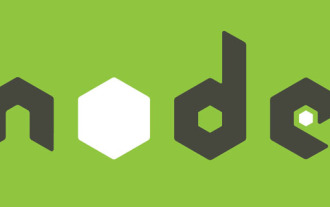
During this period, I was developing a HTML dynamic service that is common to all categories of Tencent documents. In order to facilitate the generation and deployment of access to various categories, and to follow the trend of cloud migration, I considered using Docker to fix service content and manage product versions in a unified manner. . This article will share the optimization experience I accumulated in the process of serving Docker for your reference.
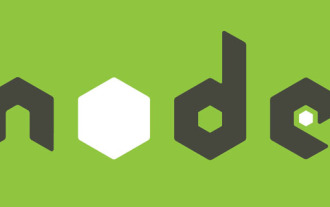
This article will share with you Node's process management tool "pm2", and talk about why pm2 is needed, how to install and use pm2, I hope it will be helpful to everyone!
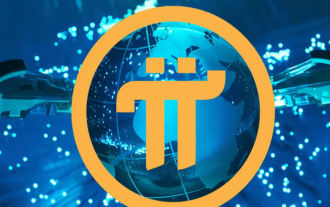
Detailed explanation and installation guide for PiNetwork nodes This article will introduce the PiNetwork ecosystem in detail - Pi nodes, a key role in the PiNetwork ecosystem, and provide complete steps for installation and configuration. After the launch of the PiNetwork blockchain test network, Pi nodes have become an important part of many pioneers actively participating in the testing, preparing for the upcoming main network release. If you don’t know PiNetwork yet, please refer to what is Picoin? What is the price for listing? Pi usage, mining and security analysis. What is PiNetwork? The PiNetwork project started in 2019 and owns its exclusive cryptocurrency Pi Coin. The project aims to create a one that everyone can participate
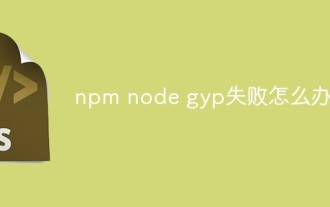
npm node gyp fails because "node-gyp.js" does not match the version of "Node.js". The solution is: 1. Clear the node cache through "npm cache clean -f"; 2. Through "npm install -g n" Install the n module; 3. Install the "node v12.21.0" version through the "n v12.21.0" command.
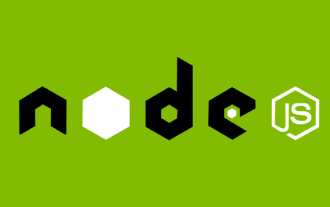
How to package nodejs executable file with pkg? The following article will introduce to you how to use pkg to package a Node project into an executable file. I hope it will be helpful to you!
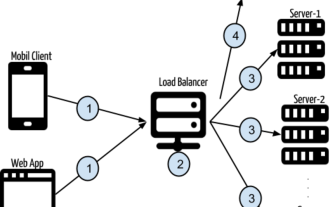
Authentication is one of the most important parts of any web application. This tutorial discusses token-based authentication systems and how they differ from traditional login systems. By the end of this tutorial, you will see a fully working demo written in Angular and Node.js. Traditional Authentication Systems Before moving on to token-based authentication systems, let’s take a look at traditional authentication systems. The user provides their username and password in the login form and clicks Login. After making the request, authenticate the user on the backend by querying the database. If the request is valid, a session is created using the user information obtained from the database, and the session information is returned in the response header so that the session ID is stored in the browser. Provides access to applications subject to
