Compare three implementations of Ajax and JSON parsing
This article will introduce you to the three implementations of ajax and related information on json parsing. Friends who are interested in this article can refer to it
This article mainly compares three ways to implement Ajax, and provides a starting point for future learning. .
Preparation:
1、 prototype.js
2、 jquery1.3.2.min.js
3、 json2.js
Background handler (Servlet), access path servlet/testAjax:
Java code
##
package ajax.servlet; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * Ajax例子后台处理程序 * @author bing * @version 2011-07-07 * */ public class TestAjaxServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html;charset=utf-8"); PrintWriter out = response.getWriter(); String name = request.getParameter("name"); String age = request.getParameter("age"); System.out.println("{\"name\":\"" + name + "\",\"age\":\"" + age + "\"}"); out.print("{\"name\":\"" + name + "\",\"age\":" + age + "}"); out.flush(); out.close(); } public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request,response); } }
Html code
<p id="show">显示区域</p> <p id="parameters"> name:<input id="name" name="name" type="text" /><br /> age:<input id="age" name="age" type="text" /><br /> </p>
1. Prototype implementation
##Html code<script type="text/javascript" src="prototype.js"></script> <script type="text/javascript"> function prototypeAjax() { var url = "servlet/testAjax";//请求URL var params = Form.serialize("parameters");//提交的表单 var myAjax = new Ajax.Request( url,{ method:"post",// 请求方式 parameters:params, // 参数 onComplete:pressResponse, // 响应函数 asynchronous:true }); $("show").innerHTML = "正在处理中..."; } function pressResponse(request) { var obj = request.responseText; // 以文本方式接收 $("show").innerHTML = obj; var objJson = request.responseText.evalJSON(); // 将接收的文本用解析成Json格式 $("show").innerHTML += "name=" + objJson['name'] + " age=" + objJson['age']; } </script> <input id="submit" type="button" value="提交" onclick="prototypeAjax()" /><br />
In prototype In Ajax implementation, the evalJSON method is used to convert strings into JSON objects.
Html code
<script type="text/javascript" src="jquery-1.3.2.min.js"></script> <script type="text/javascript" src="json2.js"></script> <input id="submit" type="button" value="提交" /><br /> <script type="text/javascript"> function jqueryAjax() { var user={"name":"","age":""}; user.name= $("#name").val(); user.age=$("#age").val(); var time = new Date(); $.ajax({ url: "servlet/testAjax?time="+time, data: "name="+user.name+"&age="+user.age, datatype: "json",//请求页面返回的数据类型 type: "GET", contentType: "application/json",//注意请求页面的contentType 要于此处保持一致 success:function(data) {//这里的data是由请求页面返回的数据 var dataJson = JSON.parse(data); // 使用json2.js中的parse方法将data转换成json格式 $("#show").html("data=" + data + " name="+dataJson.name+" age=" + dataJson.age); }, error: function(XMLHttpRequest, textStatus, errorThrown) { $("#show").html("error"); } }); } $("#submit").bind("click",jqueryAjax); // 绑定提交按钮 </script>
##3. XMLHttpRequest implementation
Html code
<script type="text/javascript">
var xmlhttp;
function XMLHttpRequestAjax()
{
// 获取数据
var name = document.getElementById("name").value;
var age = document.getElementById("age").value;
// 获取XMLHttpRequest对象
if(window.XMLHttpRequest){
xmlhttp = new XMLHttpRequest();
}else if(window.ActiveXObject){
var activxName = ["MSXML2.XMLHTTP","Microsoft.XMLHTTP"];
for(var i = 0 ; i < activexName.length; i++){
try{
xmlhttp = new ActiveXObject(activexName[i]);
break;
}catch(e){}
}
}
xmlhttp.onreadystatechange = callback; //回调函数
var time = new Date();// 在url后加上时间,使得每次请求不一样
var url = "servlet/testAjax?name="+name+"&age="+age+"&time="+time;
xmlhttp.open("GET",url,true); // 以get方式发送请求
xmlhttp.send(null); // 参数已在url中,所以此处不需要参送
}
function callback(){
if(xmlhttp.readyState == 4){
if(xmlhttp.status == 200){ // 响应成功
var responseText = xmlhttp.responseText; // 以文本方式接收响应信息
var userp = document.getElementById("show");
var responseTextJson = JSON.parse(responseText); // 使用json2.js中的parse方法将data转换成json格式
userp.innerHTML=responseText + " name=" + responseTextJson.name + " age=" + responseTextJson.age;
}
}
}
</script>
<input id="submit" type="button" value="提交" onclick="XMLHttpRequestAjax()" /><br />
ps: Three ways to convert strings into JSON
During project development using Ajax, it is often necessary to convert JSON format into The string is returned to the front end, and the front end parses it into a JS object (JSON). ECMA-262(E3) did not write the JSON concept into the standard, but in ECMA-262(E5) the concept of JSON was officially introduced, including the global JSON object and the Date toJSON method.
1, eval method analysis, I am afraid this is the earliest analysis method.
function strToJson(str){
var json = eval('(' + str + ')');
return json;
}
Remember the parentheses on both sides of str.
2, the new Function form is quite weird.
function strToJson(str){
var json = (new Function("return " + str))();
return json;
}
In IE6/7, when the string contains a newline (\n), new Function cannot parse it, but eval can.
3, use the global JSON object.
function strToJson(str){
return JSON.parse(str);
}
Currently IE8(S)/Firefox3.5/Chrome4/Safari4/Opera10 has implemented this method.
When using JSON.parse, you must strictly abide by the JSON specification. For example, attributes need to be enclosed in quotation marks, as follows
var str = '{name:"jack"}'; var obj = JSON.parse(str); // --> parse error
name is not enclosed in quotation marks Now, when using JSON.parse, exceptions are thrown in all browsers and parsing fails. The first two methods are fine.
The above is what I compiled for everyone. I hope it will be helpful to everyone in the future.
Related articles:
Manual solution through Ajax WordPress WP-PostViews does not count the problem
The above is the detailed content of Compare three implementations of Ajax and JSON parsing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
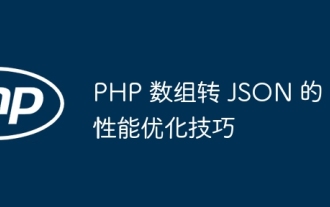
Performance optimization methods for converting PHP arrays to JSON include: using JSON extensions and the json_encode() function; adding the JSON_UNESCAPED_UNICODE option to avoid character escaping; using buffers to improve loop encoding performance; caching JSON encoding results; and considering using a third-party JSON encoding library.
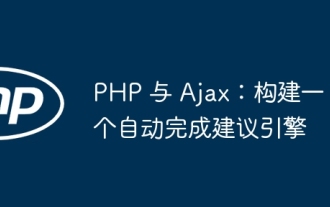
Build an autocomplete suggestion engine using PHP and Ajax: Server-side script: handles Ajax requests and returns suggestions (autocomplete.php). Client script: Send Ajax request and display suggestions (autocomplete.js). Practical case: Include script in HTML page and specify search-input element identifier.
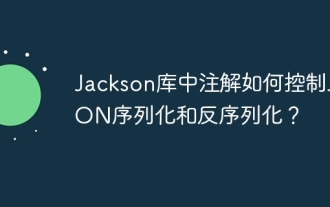
Annotations in the Jackson library control JSON serialization and deserialization: Serialization: @JsonIgnore: Ignore the property @JsonProperty: Specify the name @JsonGetter: Use the get method @JsonSetter: Use the set method Deserialization: @JsonIgnoreProperties: Ignore the property @ JsonProperty: Specify name @JsonCreator: Use constructor @JsonDeserialize: Custom logic
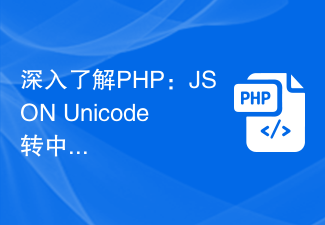
In-depth understanding of PHP: Implementation method of converting JSONUnicode to Chinese During development, we often encounter situations where we need to process JSON data, and Unicode encoding in JSON will cause us some problems in some scenarios, especially when Unicode needs to be converted When encoding is converted to Chinese characters. In PHP, there are some methods that can help us achieve this conversion process. A common method will be introduced below and specific code examples will be provided. First, let us first understand the Un in JSON
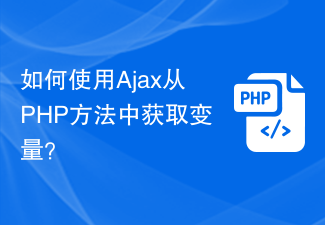
Using Ajax to obtain variables from PHP methods is a common scenario in web development. Through Ajax, the page can be dynamically obtained without refreshing the data. In this article, we will introduce how to use Ajax to get variables from PHP methods, and provide specific code examples. First, we need to write a PHP file to handle the Ajax request and return the required variables. Here is sample code for a simple PHP file getData.php:
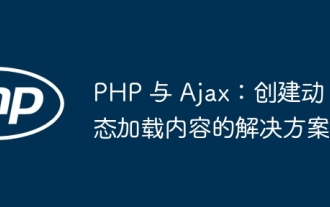
Ajax (Asynchronous JavaScript and XML) allows adding dynamic content without reloading the page. Using PHP and Ajax, you can dynamically load a product list: HTML creates a page with a container element, and the Ajax request adds the data to that element after loading it. JavaScript uses Ajax to send a request to the server through XMLHttpRequest to obtain product data in JSON format from the server. PHP uses MySQL to query product data from the database and encode it into JSON format. JavaScript parses the JSON data and displays it in the page container. Clicking the button triggers an Ajax request to load the product list.
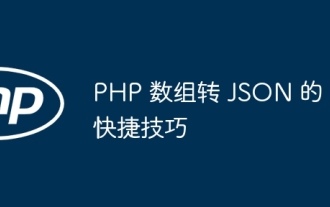
PHP arrays can be converted to JSON strings through the json_encode() function (for example: $json=json_encode($array);), and conversely, the json_decode() function can be used to convert from JSON to arrays ($array=json_decode($json);) . Other tips include avoiding deep conversions, specifying custom options, and using third-party libraries.
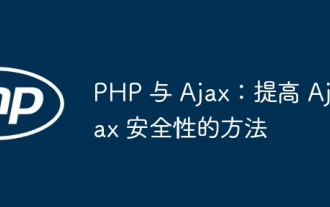
In order to improve Ajax security, there are several methods: CSRF protection: generate a token and send it to the client, add it to the server side in the request for verification. XSS protection: Use htmlspecialchars() to filter input to prevent malicious script injection. Content-Security-Policy header: Restrict the loading of malicious resources and specify the sources from which scripts and style sheets are allowed to be loaded. Validate server-side input: Validate input received from Ajax requests to prevent attackers from exploiting input vulnerabilities. Use secure Ajax libraries: Take advantage of automatic CSRF protection modules provided by libraries such as jQuery.
