


Detailed explanation of JavaScript string manipulation methods and browser compatibility examples
slice()
Definition: Accepts one or two parameters, the first parameter specifies the starting position of the substring. The second parameter represents the end position of the substring (excluding the character at the end position). If the second parameter is not passed, the length of the string is used as the end position.
1. When the passed parameter is a positive value:
var str ="helloWorld"; // 一个参数,则将字符串长度作为结束位置 alert(str.slice(3)); // "loWorld" // 两个参数,7位置上的字符为"r",但不包括结束位置的字符 alert(str.slice(3,7)); // "loWo"
2. When the passed parameter is a negative value:
slice()方法会将传入的负值与字符串长度相加。 var str ="helloWorld"; // 一个参数,与字符串长度相加即为slice(7) alert(str.slice(-3)); // "rld" // 两个参数,与字符串长度相加即为slice(3,6) alert(str.slice(3,-4)); // "loW"
3. When the second parameter is smaller than the first parameter:
The second parameter passed in by the slice() method is smaller than the first parameter. If the first parameter is small, an empty string is returned.
var str ="helloWorld"; alert(str.slice(5,3)); // ""
4. IE compatibility
Under the IE8 browser test, there is no problem and the behavior is consistent with modern browsers .
substring()
Definition: Accepts one or two parameters, the first parameter specifies the starting position of the substring. The second parameter represents the end position of the substring (excluding the character at the end position). If the second parameter is not passed, the length of the string is used as the end position.
1. The passed parameter is a positive value: the same behavior as the slice() method
var str ="helloWorld"; // 一个参数,则将字符串长度作为结束位置 alert(str.substring(3)); // "loWorld" // 两个参数,7位置上的字符为"r",但不包括结束位置的字符 alert(str.substring(3,7)); // "loWo"
2. The passed parameter is In the case of negative values: The
substring() method will convert all negative parameters to 0. Let’s look at an example:
var str ="helloWorld"; // 两个参数,-4会转换为0,相当于substring(3,0) -->即为 substring(0,3) alert(str.substring(3,-4)); // "hel"
The substring() method will use the smaller number as the starting position and the larger number as the ending position. As in the above example, substring(3,0) and substring(0,3) have the same effect.
4. IE compatibility
Under the IE8 browser test, there is no problem and the behavior is consistent with modern browsers.
substr()
Definition: Accepts one or two parameters, the first parameter specifies the starting position of the substring. The second parameter is somewhat different from the previous method, indicating the number of characters returned. If no second argument is passed, the length of the string is used as the ending position. Let’s look at an example:
1. When the passed parameter is a positive value:
var str ="helloWorld"; // 一个参数,则将字符串长度作为结束位置 alert(str.substr(3)); // "loWorld" // 两个参数,从位置3开始截取后面7个字符 alert(str.substr(3,7)); // "loWorld"
2. When the passed parameter is a negative value :
The substr() method will add the negative first parameter to the length of the string, and convert the negative second parameter to 0.
var str ="helloWorld"; // 将第一个负的参数加上字符串的长度---> //即为:substr(7,5) ,从位置7开始向后截取5个字符 alert(str.substr(-3,5)); // "rld" // 将第二个参数转换为0 // 即为:substr(3,0),即从位置3截取0个字符串,则返回空 alert(str.substr(3,-2)); // ""
3. IE compatibility
There will be a problem when the substr() method passes a negative value and the original value will be returned. String. IE9 fixes this issue.
Let me introduce to you the difference between slice, substr and substring
First of all, they both receive two parameters. Slice and substring receive the starting position and the ending position. (excluding the end position), while substr receives the starting position and the length of the string to be returned. Look directly at the following example:
var test = 'hello world'; alert(test.slice(,)); //o w alert(test.substring(,)); //o w alert(test.substr(,)); //o world
Something to note here is: substring uses the smaller of the two parameters as the starting position, the larger parameter as the end position.
For example:
alert(test.substring(7,4)); //o w
Then, when the received parameter is a negative number, slice will compare the length of its string with the corresponding negative number. Add, and the result is used as a parameter; substr just adds the first parameter to the length of the string as the first parameter; substring simply converts all negative parameters directly to 0. The test code is as follows:
var test = 'hello world'; alert(test.slice(-)); //rld alert(test.substring(-)); //hello world alert(test.substr(-)); //rld alert(test.slice(,-)); //lo w alert(test.substring(,-)); //hel alert(test.substr(,-)); //空字符串
Note: IE has an error in handling substr's negative value, and it will return the original string.
The above is the detailed content of Detailed explanation of JavaScript string manipulation methods and browser compatibility examples. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
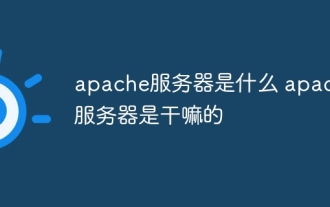
Apache server is a powerful web server software that acts as a bridge between browsers and website servers. 1. It handles HTTP requests and returns web page content based on requests; 2. Modular design allows extended functions, such as support for SSL encryption and dynamic web pages; 3. Configuration files (such as virtual host configurations) need to be carefully set to avoid security vulnerabilities, and optimize performance parameters, such as thread count and timeout time, in order to build high-performance and secure web applications.
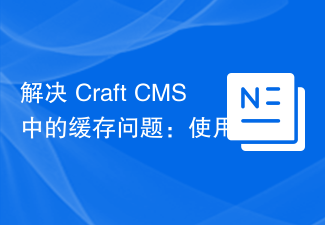
When developing websites using CraftCMS, you often encounter resource file caching problems, especially when you frequently update CSS and JavaScript files, old versions of files may still be cached by the browser, causing users to not see the latest changes in time. This problem not only affects the user experience, but also increases the difficulty of development and debugging. Recently, I encountered similar troubles in my project, and after some exploration, I found the plugin wiejeben/craft-laravel-mix, which perfectly solved my caching problem.
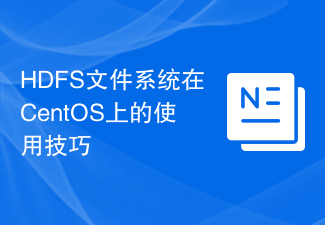
The Installation, Configuration and Optimization Guide for HDFS File System under CentOS System This article will guide you how to install, configure and optimize Hadoop Distributed File System (HDFS) on CentOS System. HDFS installation and configuration Java environment installation: First, make sure that the appropriate Java environment is installed. Edit /etc/profile file, add the following, and replace /usr/lib/java-1.8.0/jdk1.8.0_144 with your actual Java installation path: exportJAVA_HOME=/usr/lib/java-1.8.0/jdk1.8.0_144exportPATH=$J
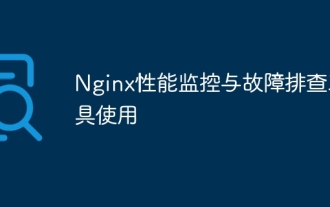
Nginx performance monitoring and troubleshooting are mainly carried out through the following steps: 1. Use nginx-V to view version information, and enable the stub_status module to monitor the number of active connections, requests and cache hit rate; 2. Use top command to monitor system resource occupation, iostat and vmstat monitor disk I/O and memory usage respectively; 3. Use tcpdump to capture packets to analyze network traffic and troubleshoot network connection problems; 4. Properly configure the number of worker processes to avoid insufficient concurrent processing capabilities or excessive process context switching overhead; 5. Correctly configure Nginx cache to avoid improper cache size settings; 6. By analyzing Nginx logs, such as using awk and grep commands or ELK
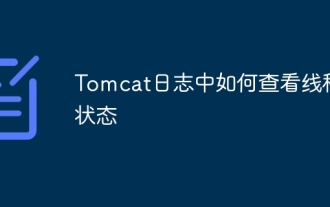
To view the thread status in the Tomcat log, you can use the following methods: TomcatManagerWeb interface: Enter the management address of Tomcat (usually http://localhost:8080/manager) in the browser, and you can view the status of the thread pool after logging in. JMX Monitoring: Use JMX monitoring tools (such as JConsole) to connect to Tomcat's MBean server to view the status of Tomcat's thread pool. Select in JConsole
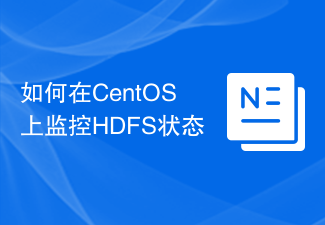
There are many ways to monitor the status of HDFS (Hadoop Distributed File System) on CentOS systems. This article will introduce several commonly used methods to help you choose the most suitable solution. 1. Use Hadoop’s own WebUI, Hadoop’s own Web interface to provide cluster status monitoring function. Steps: Make sure the Hadoop cluster is up and running. Access the WebUI: Enter http://:50070 (Hadoop2.x) or http://:9870 (Hadoop3.x) in your browser. The default username and password are usually hdfs/hdfs. 2. Command line tool monitoring Hadoop provides a series of command line tools to facilitate monitoring
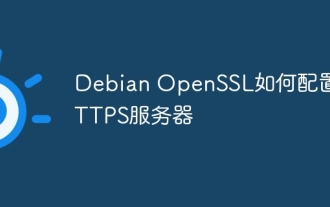
Configuring an HTTPS server on a Debian system involves several steps, including installing the necessary software, generating an SSL certificate, and configuring a web server (such as Apache or Nginx) to use an SSL certificate. Here is a basic guide, assuming you are using an ApacheWeb server. 1. Install the necessary software First, make sure your system is up to date and install Apache and OpenSSL: sudoaptupdatesudoaptupgradesudoaptinsta
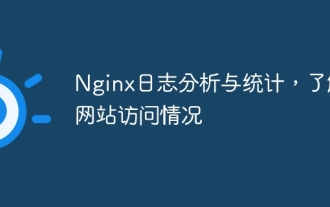
This article describes how to analyze Nginx logs to improve website performance and user experience. 1. Understand the Nginx log format, such as timestamps, IP addresses, status codes, etc.; 2. Use tools such as awk to parse logs and count indicators such as visits, error rates, etc.; 3. Write more complex scripts according to needs or use more advanced tools, such as goaccess, to analyze data from different dimensions; 4. For massive logs, consider using distributed frameworks such as Hadoop or Spark. By analyzing logs, you can identify website access patterns, improve content strategies, and ultimately optimize website performance and user experience.
