How does jQuery get and calculate the length of an object?
In our daily development, Objects are used very frequently. It is very convenient for us to calculate the length of the array, but how to calculate the length of the object? Suppose we have a library project with a set of books and authors, like the following:
var bookAuthors = { "Farmer Giles of Ham": "J.R.R. Tolkien", "Out of the Silent Planet": "C.S. Lewis", "The Place of the Lion": "Charles Williams", "Poetic Diction": "Owen Barfield" };
We analyze the current needs and send data to an API, but the length of the book cannot exceed 100, So we need to calculate the total number of books in an object before sending the data. So what do we always do? We might do this:
function countProperties (obj) { var count = 0; for (var property in obj) { if (Object.prototype.hasOwnProperty.call(obj, property)) { count++; } } return count; } var bookCount = countProperties(bookAuthors); // Outputs: 4 console.log(bookCount);
This is achievable, fortunately Javascript provides a changed method to calculate the length of the object:
var bookAuthors = { "Farmer Giles of Ham": "J.R.R. Tolkien", "Out of the Silent Planet": "C.S. Lewis", "The Place of the Lion": "Charles Williams", "Poetic Diction": "Owen Barfield" }; var arr = Object.keys(bookAuthors); //Outputs: Array [ "Farmer Giles of Ham", "Out of the Silent Planet", "The Place of the Lion", "Poetic Diction" ] console.log(arr); //Outputs: 4 console.log(arr.length);
Below we To use the keys method on the array:
var arr = ["zuojj", "benjamin", "www.zuojj.com"]; //Outputs: ["0", "1", "2"] console.log(Object.keys(arr)); //Outputs: 3 console.log(arr.length);
Object.keys() method will return a property name consisting of all the enumerable own properties of the given object The array, the order of the attribute names in the array is consistent with the order returned when using a for-in loop to traverse the object (the main difference between the two is that for-in will also traverse an object from its prototype chainInherit to the enumerable properties).
Get the length of an object in JavaScript:
/** * jQuery 扩展方法 * * $.Object.count( p ) * 获取一个对象的长度,需要指定上下文,通过 call/apply 调用 * 示例: $.Object.count.call( obj, true ); * @param {p} 是否跳过 null / undefined / 空值 * */ $.extend({ // 获取对象的长度,需要指定上下文 this Object: { count: function( p ) { p = p || false; return $.map( this, function(o) { if( !p ) return o; return true; } ).length; } } }); // 示例 // --------------------------------------------------------------------------- var obj = { a: null, b: undefined, c: 1, d: 2, e: 'test' }; // 不过滤空值 console.log( $.Object.count.call( obj ) ); // 过滤空值 console.log( $.Object.count.call( obj, true ) );
The above is the detailed content of How does jQuery get and calculate the length of an object?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
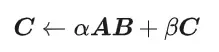
General Matrix Multiplication (GEMM) is a vital part of many applications and algorithms, and is also one of the important indicators for evaluating computer hardware performance. In-depth research and optimization of the implementation of GEMM can help us better understand high-performance computing and the relationship between software and hardware systems. In computer science, effective optimization of GEMM can increase computing speed and save resources, which is crucial to improving the overall performance of a computer system. An in-depth understanding of the working principle and optimization method of GEMM will help us better utilize the potential of modern computing hardware and provide more efficient solutions for various complex computing tasks. By optimizing the performance of GEMM
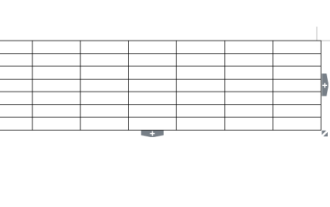
WORD is a powerful word processor. We can use word to edit various texts. In Excel tables, we have mastered the calculation methods of addition, subtraction and multipliers. So if we need to calculate the addition of numerical values in Word tables, How to subtract the multiplier? Can I only use a calculator to calculate it? The answer is of course no, WORD can also do it. Today I will teach you how to use formulas to calculate basic operations such as addition, subtraction, multiplication and division in tables in Word documents. Let's learn together. So, today let me demonstrate in detail how to calculate addition, subtraction, multiplication and division in a WORD document? Step 1: Open a WORD, click [Table] under [Insert] on the toolbar, and insert a table in the drop-down menu.
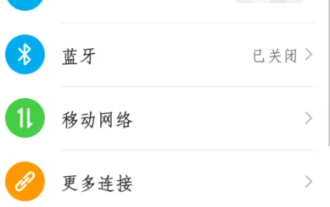
Google Authenticator is a tool used to protect the security of user accounts, and its key is important information used to generate dynamic verification codes. If you forget the key of Google Authenticator and can only verify it through the security code, then the editor of this website will bring you a detailed introduction on where to get the Google security code. I hope it can help you. If you want to know more Users please continue reading below! First open the phone settings and enter the settings page. Scroll down the page and find Google. Go to the Google page and click on Google Account. Enter the account page and click View under the verification code. Enter your password or use your fingerprint to verify your identity. Obtain a Google security code and use the security code to verify your Google identity.
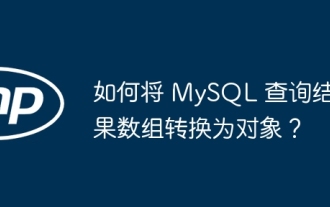
Here's how to convert a MySQL query result array into an object: Create an empty object array. Loop through the resulting array and create a new object for each row. Use a foreach loop to assign the key-value pairs of each row to the corresponding properties of the new object. Adds a new object to the object array. Close the database connection.
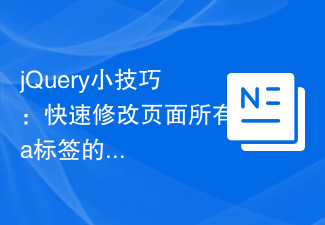
Title: jQuery Tips: Quickly modify the text of all a tags on the page In web development, we often need to modify and operate elements on the page. When using jQuery, sometimes you need to modify the text content of all a tags in the page at once, which can save time and energy. The following will introduce how to use jQuery to quickly modify the text of all a tags on the page, and give specific code examples. First, we need to introduce the jQuery library file and ensure that the following code is introduced into the page: <
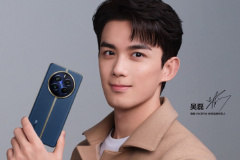
Although the general operations of domestic mobile phones are very similar, there are still some differences in some details. For example, different mobile phone models and manufacturers may have different dual-SIM installation methods. Erzhenwo 12Pro, a new mobile phone, also supports dual-SIM dual standby, but how should dual-SIM be installed on this phone? How to install dual SIM on Realme 12Pro? Remember to turn off your phone before installation. Step 1: Find the SIM card tray: Find the SIM card tray of the phone. Usually, in the Realme 12 Pro, the SIM card tray is located on the side or top of the phone. Step 2: Insert the first SIM card. Use a dedicated SIM card pin or a small object to insert it into the slot in the SIM card tray. Then, carefully insert the first SIM card.
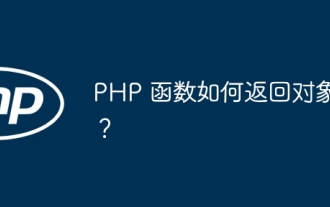
PHP functions can encapsulate data into a custom structure by returning an object using a return statement followed by an object instance. Syntax: functionget_object():object{}. This allows creating objects with custom properties and methods and processing data in the form of objects.
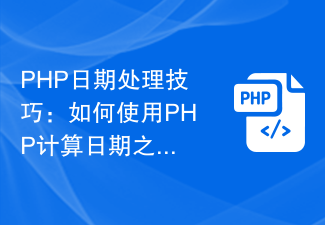
PHP date processing tips: How to calculate the month difference between dates using PHP? Date processing is a very common requirement in web development, especially in business logic that needs to be related to time. In PHP, calculating the month difference between dates can be achieved through some methods. This article will introduce how to use PHP to calculate the month difference between two dates and provide specific code examples. Method 1: Use the DateTime class. PHP's DateTime class provides a wealth of date processing methods, including calculating dates.
