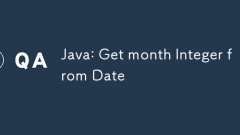
Java: Get month Integer from Date
Problem statement Extracting month integers from date objects is a basic skill for developers. This is because you may encounter tasks like generating monthly reports, filtering dates by month, and scheduling events. In this article, we will try to familiarize ourselves with Java's powerful date and time API. Prerequisites Let's dive right into the Java task at hand, making sure you consider the following points. Make sure you are familiar with the basic Java syntax, especially the date and time API. You will use syntaxes such as java.util.Date, java.util.Calendar, and modern java.time packages (recently introduced in Java 8).
Feb 07, 2025 am 11:41 AM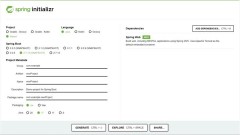
How to Run Your First Spring Boot Application in IntelliJ?
IntelliJ IDEA simplifies Spring Boot development, making it a favorite among Java developers. Its convention-over-configuration approach minimizes boilerplate code, allowing developers to focus on business logic. This tutorial demonstrates two metho
Feb 07, 2025 am 11:40 AM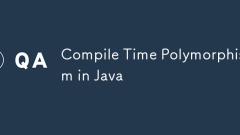
Compile Time Polymorphism in Java
Polymorphism in Java refers to a capability declaration of objects in the Java environment. It allows us to perform the same process in different ways. There are two types of polymorphisms in Java: Compilation-time polymorphism method Runtime polymorphism method Today, we will discuss compile-time polymorphisms using method overloading and operator overloading. Example of compile-time polymorphism Here is an example: void ARBRDD() { ... } void ARBRDD(int num1 ) { ... } void ARBRDD(float num1) { ... } void ARBRDD(int num1 , float num2 ) {
Feb 07, 2025 am 11:39 AM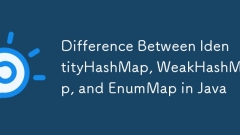
Difference Between IdentityHashMap, WeakHashMap, and EnumMap in Java
This article explores the nuances of IdentityHashMap, WeakHashMap, and EnumMap in Java, highlighting their key differences through various parameters. IdentityHashMap handles reference equality using the == operator, unlike standard HashMaps which r
Feb 07, 2025 am 11:38 AM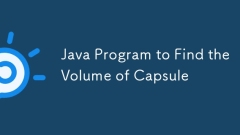
Java Program to Find the Volume of Capsule
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
Feb 07, 2025 am 11:37 AM
Java program to delete all even nodes from a singly linked list
This Java program efficiently removes all even-valued nodes from a singly linked list. Let's refine the explanation and presentation for clarity. This article demonstrates how to remove all even-numbered nodes from a singly linked list in Java. We'll
Feb 07, 2025 am 11:36 AM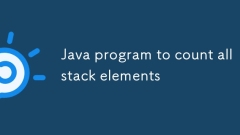
Java program to count all stack elements
This tutorial will introduce several methods to calculate the number of elements in the Java stack. In Java, the stack is a basic data structure that follows the last in first out (LIFO) principle, which means that the elements recently added to the stack will be accessed first. The practical applications of the stack include function call management, expression evaluation, etc. In these scenarios, we may need to calculate the number of elements in the stack. For example, when using the stack for function call management, you need to calculate the total number of function calls; when using the stack for evaluation, you need to calculate the total number of operations to be performed. We will explore three ways to calculate the number of elements in the stack: Use Stack.size() method Using a for loop (iteration method) Using recursive methods Use Stack.size() method count
Feb 07, 2025 am 11:35 AM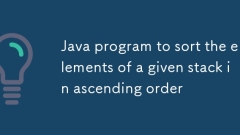
Java program to sort the elements of a given stack in ascending order
This tutorial will guide you how to sort stack elements in ascending order using Java. Stacks are the basic data structures in computer science, following the last-in-first-out (LIFO) principle. We will break down a simple and efficient method that uses an additional temporary stack, provides detailed step-by-step instructions, and includes a complete code example. This tutorial is ideal for those who want to enhance their understanding of stack operations and improve their Java programming skills. Sort the stack in ascending order using Java The stack is like a pile of books, you can only take the top one. That is, the stack is stored in first-out (LIFO) mode. The last item added is the first item removed. The following is the sorting of stack elements using the auxiliary stack
Feb 07, 2025 am 11:34 AM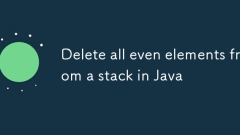
Delete all even elements from a stack in Java
This tutorial demonstrates two methods for eliminating even numbers from a Java stack. Stacks, adhering to the Last-In-First-Out (LIFO) principle, present a unique challenge for this type of filtering. The techniques shown here are adaptable to oth
Feb 07, 2025 am 11:32 AM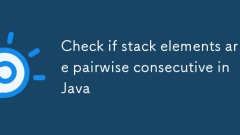
Check if stack elements are pairwise consecutive in Java
Stacks are a basic data structure in computer science and are often used for their latter-in-first-out (LIFO) attributes. When using the stack, you may encounter an interesting problem, which is to check if the elements of the stack are continuous in pairs. In this article, we will learn how to solve this problem using Java to ensure that the solution is efficient and clear. Problem statement Given an integer stack, the task is to determine whether the elements of the stack are continuous in pairs. If the difference between two elements is exactly 1, they are considered continuous. enter 4, 5, 2, 3, 10, 11 Output Are elements continuous in pairs? true Steps to check whether stack elements are paired and contiguous Here are the steps to check whether the stack elements are paired and contiguous: Inspection
Feb 07, 2025 am 11:31 AM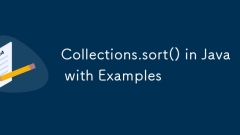
Collections.sort() in Java with Examples
This article explores the Collections.sort() method in Java, a powerful tool for sorting elements within collections like lists and arrays. While a TreeSet can also sort elements, Collections.sort() offers flexibility and efficiency, especially when
Feb 07, 2025 am 11:29 AM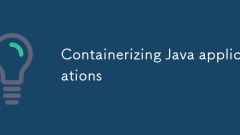
Containerizing Java applications
Containerization, a core concept in Java application deployment, involves packaging a Java service or application within a software container. This container bundles all necessary components for execution. This approach offers several key advantages
Feb 07, 2025 am 11:28 AM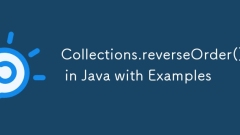
Collections.reverseOrder() in Java with Examples
The Collections.reverseOrder() method in Java provides a convenient way to reverse the natural ordering of elements within a collection. This method returns a Comparator that imposes the reverse ordering on a given collection. There are two variati
Feb 07, 2025 am 11:26 AM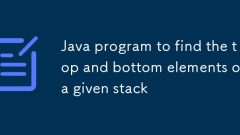
Java program to find the top and bottom elements of a given stack
This tutorial will explain how to use Java to find the top and bottom elements of a given stack. The stack represents a linear dataset that follows the last-in first-out (LIFO) principle, so elements are added and removed at the same location. We will further explore two ways to find the top and bottom elements of a given stack, i.e. through iterative and recursion. Problem statement We will get an array of stacks containing n elements, and the task is to find the 1st and nth elements of the stack without destroying it in any way. Therefore, we need to use iterative methods and recursive methods to perform peek() operations in our custom stack to ensure that the original stack remains unchanged. Enter 1 stack = [5, 10, 15, 20, 25, 30] Output
Feb 07, 2025 am 11:25 AM
Hot tools Tags

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

vc9-vc14 (32+64 bit) runtime library collection (link below)
Download the collection of runtime libraries required for phpStudy installation

VC9 32-bit
VC9 32-bit phpstudy integrated installation environment runtime library

PHP programmer toolbox full version
Programmer Toolbox v1.0 PHP Integrated Environment

VC11 32-bit
VC11 32-bit phpstudy integrated installation environment runtime library

SublimeText3 Chinese version
Chinese version, very easy to use
