在Python的web框架中编写创建日志的程序的教程
在Web开发中,后端代码写起来其实是相当容易的。
例如,我们编写一个REST API,用于创建一个Blog:
@api @post('/api/blogs') def api_create_blog(): i = ctx.request.input(name='', summary='', content='') name = i.name.strip() summary = i.summary.strip() content = i.content.strip() if not name: raise APIValueError('name', 'name cannot be empty.') if not summary: raise APIValueError('summary', 'summary cannot be empty.') if not content: raise APIValueError('content', 'content cannot be empty.') user = ctx.request.user blog = Blog(user_id=user.id, user_name=user.name, name=name, summary=summary, content=content) blog.insert() return blog
编写后端Python代码不但很简单,而且非常容易测试,上面的API:api_create_blog()本身只是一个普通函数。
Web开发真正困难的地方在于编写前端页面。前端页面需要混合HTML、CSS和JavaScript,如果对这三者没有深入地掌握,编写的前端页面将很快难以维护。
更大的问题在于,前端页面通常是动态页面,也就是说,前端页面往往是由后端代码生成的。
生成前端页面最早的方式是拼接字符串:
s = '<html><head><title>' + title + '</title></head><body>' + body + '</body></html>'
显然这种方式完全不具备可维护性。所以有第二种模板方式:
<html> <head> <title>{{ title }}</title> </head> <body> {{ body }} </body> </html>
ASP、JSP、PHP等都是用这种模板方式生成前端页面。
如果在页面上大量使用JavaScript(事实上大部分页面都会),模板方式仍然会导致JavaScript代码与后端代码绑得非常紧密,以至于难以维护。其根本原因在于负责显示的HTML DOM模型与负责数据和交互的JavaScript代码没有分割清楚。
要编写可维护的前端代码绝非易事。和后端结合的MVC模式已经无法满足复杂页面逻辑的需要了,所以,新的MVVM:Model View ViewModel模式应运而生。
MVVM最早由微软提出来,它借鉴了桌面应用程序的MVC思想,在前端页面中,把Model用纯JavaScript对象表示:
<script> var blog = { name: 'hello', summary: 'this is summary', content: 'this is content...' }; </script>
View是纯HTML:
<form action="/api/blogs" method="post"> <input name="name"> <input name="summary"> <textarea name="content"></textarea> <button type="submit">OK</button> </form>
由于Model表示数据,View负责显示,两者做到了最大限度的分离。
把Model和View关联起来的就是ViewModel。ViewModel负责把Model的数据同步到View显示出来,还负责把View的修改同步回Model。
ViewModel如何编写?需要用JavaScript编写一个通用的ViewModel,这样,就可以复用整个MVVM模型了。
好消息是已有许多成熟的MVVM框架,例如AngularJS,KnockoutJS等。我们选择Vue这个简单易用的MVVM框架来实现创建Blog的页面templates/manage_blog_edit.html:
{% extends '__base__.html' %} {% block title %}编辑日志{% endblock %} {% block beforehead %} <script> var action = '{{ action }}', redirect = '{{ redirect }}'; var vm; $(function () { vm = new Vue({ el: '#form-blog', data: { name: '', summary: '', content: '' }, methods: { submit: function (event) { event.preventDefault(); postApi(action, this.$data, function (err, r) { if (err) { alert(err); } else { alert('保存成功!'); return location.assign(redirect); } }); } } }); }); </script> {% endblock %} {% block content %} <div class="uk-width-1-1"> <form id="form-blog" v-on="submit: submit" class="uk-form uk-form-stacked"> <div class="uk-form-row"> <div class="uk-form-controls"> <input v-model="name" class="uk-width-1-1"> </div> </div> <div class="uk-form-row"> <div class="uk-form-controls"> <textarea v-model="summary" rows="4" class="uk-width-1-1"></textarea> </div> </div> <div class="uk-form-row"> <div class="uk-form-controls"> <textarea v-model="content" rows="8" class="uk-width-1-1"></textarea> </div> </div> <div class="uk-form-row"> <button type="submit" class="uk-button uk-button-primary">保存</button> </div> </form> </div> {% endblock %}
初始化Vue时,我们指定3个参数:
el:根据选择器查找绑定的View,这里是#form-blog,就是id为form-blog的DOM,对应的是一个

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










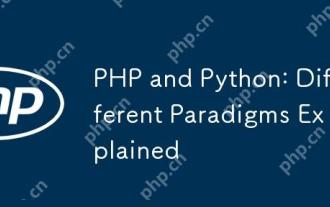
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
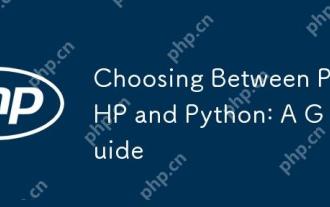
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
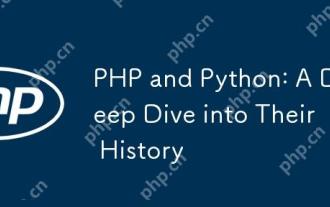
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
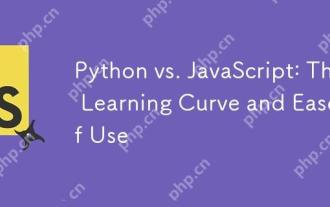
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
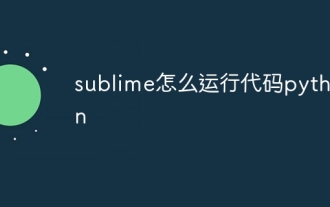
To run Python code in Sublime Text, you need to install the Python plug-in first, then create a .py file and write the code, and finally press Ctrl B to run the code, and the output will be displayed in the console.
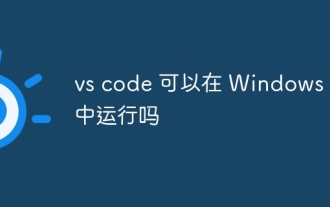
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
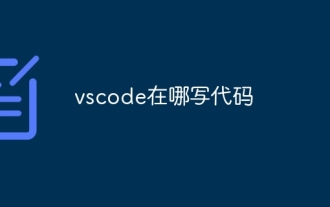
Writing code in Visual Studio Code (VSCode) is simple and easy to use. Just install VSCode, create a project, select a language, create a file, write code, save and run it. The advantages of VSCode include cross-platform, free and open source, powerful features, rich extensions, and lightweight and fast.
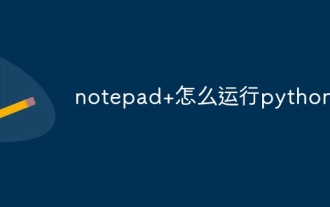
Running Python code in Notepad requires the Python executable and NppExec plug-in to be installed. After installing Python and adding PATH to it, configure the command "python" and the parameter "{CURRENT_DIRECTORY}{FILE_NAME}" in the NppExec plug-in to run Python code in Notepad through the shortcut key "F6".
