What is the impact of templated programming on code performance?
The impact of templated programming on code performance: Optimized compilation: allows the compiler to inline code, reduce function overhead, and improve performance. Code bloat: Unwrapping templated code results in increased code size, which can be a problem in resource-constrained environments. Runtime overhead: Templated code generates metadata parsing when the compiler cannot inline, potentially increasing first-call latency.
The impact of template programming on code performance
Introduction
Template programming is a A powerful technology that allows programmers to create generic code that can be customized for specific types at compile time. However, templated programming can have a significant impact on code performance.
Optimizing Compilation
One of the major advantages of templated programming is that it allows the compiler to perform optimizations. The compiler can inline templated code where it is used, eliminating the overhead of function calls. This can improve performance by reducing the number of instructions and memory accesses.
Code bloat
However, templated programming may also lead to code bloat. When the compiler expands templated code, it generates multiple type-specific versions. This can result in a significant increase in code size, which can be a problem in resource-constrained environments such as embedded systems.
Runtime overhead
In some cases, templated programming may also introduce runtime overhead. When the compiler cannot inline templated code, it must generate metadata to resolve the template at runtime. This may increase the latency of the first call because the metadata must be loaded and interpreted.
Practical Case
To illustrate the impact of templated programming on performance, let us consider a function that calculates the average of a list of numbers:
// 非模板化函数 double average(const std::vector<double>& numbers) { double sum = 0; for (const double& number : numbers) { sum += number; } return sum / numbers.size(); } // 模板化函数 template <typename T> T average(const std::vector<T>& numbers) { T sum = 0; for (const T& number : numbers) { sum += number; } return sum / numbers.size(); }
For For a list of numbers containing doubles, the performance difference between templated and non-templated functions is as follows:
Function | Execution time (microseconds) |
---|---|
##average(non-templated)
| 1.23|
average(templated)
| 1.56
Conclusion
Templated programming is a powerful tool, but it can have a significant impact on code performance. Optimizing compilation and code bloat are key factors to consider. By carefully considering the intended use of templated code, programmers can decide whether it is suitable for a specific application.The above is the detailed content of What is the impact of templated programming on code performance?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
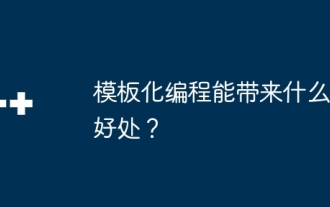
Templated programming improves code quality because it: Enhances readability: Encapsulates repetitive code, making it easier to understand. Improved maintainability: Just change the template to accommodate data type changes. Optimization efficiency: The compiler generates optimized code for specific data types. Promote code reuse: Create common algorithms and data structures that can be reused.
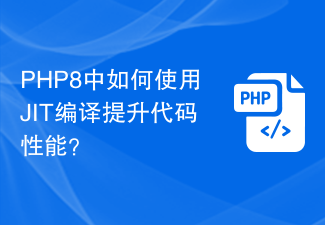
The PHP language has always been widely used to build web applications, but its performance is relatively low due to the characteristics of interpreted execution. In order to improve the performance of PHP, the JIT (Just-in-Time) compiler has been introduced since PHP7. In the new PHP8 version, the JIT compilation function has been further improved and developed to improve code performance to a greater extent. . This article will introduce how to use JIT compilation to improve code performance in PHP8, and give specific code examples. First, we need
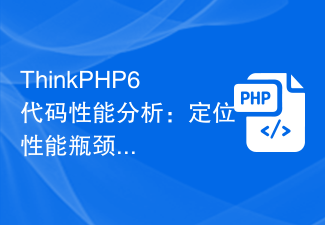
ThinkPHP6 code performance analysis: locating performance bottlenecks Introduction: With the rapid development of the Internet, more efficient code performance analysis has become increasingly important for developers. This article will introduce how to use ThinkPHP6 to perform code performance analysis in order to locate and solve performance bottlenecks. At the same time, we will also use code examples to help readers understand better. Importance of Performance Analysis Code performance analysis is an integral part of the development process. By analyzing the performance of the code, we can understand where a lot of resources are consumed
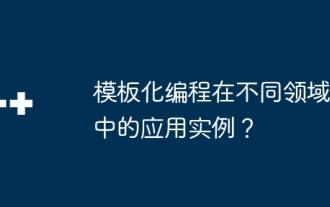
Templated programming is a paradigm for creating flexible, reusable code that is widely used in areas such as data structures, container libraries, metaprogramming, and graphics libraries. Specific examples include dynamic arrays, hash tables, priority queues, type erasure, and vertex shaders.
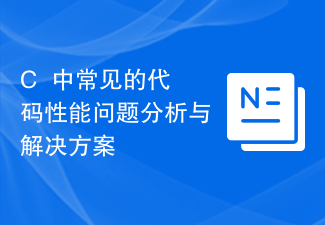
Analysis and solutions to common code performance problems in C++ Introduction: In the C++ development process, optimizing code performance is a very important task. Performance issues can cause programs to run slowly, waste resources, or even crash. This article will introduce in detail common code performance problems in C++ and provide corresponding solutions. At the same time, specific code examples will also be given so that readers can better understand and apply them. 1. Memory management issues Memory leaks Memory leaks are one of the most common performance problems in C++. When dynamically allocated memory is not allocated correctly
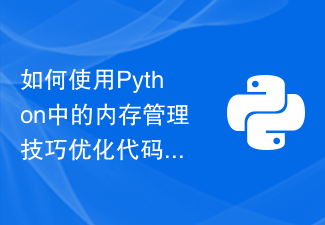
How to Optimize Code Performance Using Memory Management Tips in Python When writing Python code, optimizing performance is an important consideration. Although Python, as an interpreted language, may not be as efficient as a compiled language, we can still optimize the performance of Python code through reasonable use of memory management techniques. This article will introduce some ways to use memory management techniques in Python to optimize code performance and provide specific code examples. Avoid creating unnecessary objects: In Py
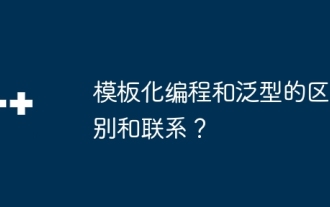
Generics and templated programming are both mechanisms in C++ to improve code reusability and type safety. Generics are type-checked at compile time, allowing different types of data to be used, whereas templated programming is compiled at instantiation time, requiring separate instantiation for each type. Despite their similarities, templated programming has a higher compile time overhead and generic functions or classes are easier to use with other types. Both mechanisms improve code reusability and type safety.
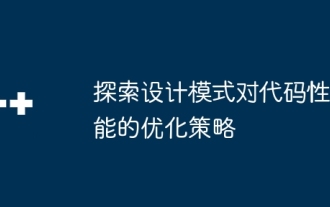
By applying design patterns, code performance can be optimized. Specific strategies include: Strategy pattern: allows dynamic exchange of algorithms, improving scalability and flexibility. Proxy pattern: Create a proxy object to control access to another object and optimize performance (such as delayed creation or caching). Factory pattern: Centralize control of object creation logic, simplify code and optimize performance.
