


Detailed explanation of C++ function optimization: How to optimize code readability and maintainability?
Function optimization techniques include: clear naming, passing parameters by value or reference, using default parameters, inline functions, constant expressions and exception handling. Optimized functions improve readability, maintainability, and robustness, such as functions that compute matrix determinants: error validation, default parameters are provided, constant expressions are used, and exception handling is used.
Detailed explanation of C function optimization: improving readability and maintainability
Preface
In the development of large-scale C projects, functions Readability and maintainability are crucial. Optimizing functions improves code clarity and reduces complexity, thereby reducing maintenance and debugging costs. This article will explore C function optimization techniques and illustrate them through practical cases.
Function naming
Function naming should be clear and reflect the purpose of the function. Avoid using vague or generic names, such as process()
or handle()
. Use names that specifically describe what the function does, such as calculate_average()
or validate_input()
.
Parameter passing
Passing by value: For primitive types and small objects, passing by value can reduce function call overhead. However, for large objects, passing by value creates copies, resulting in performance degradation.
Pass by reference: For large objects or variables that need to be modified, passing by reference can avoid copy overhead. When using reference parameters, you need to ensure that the function does not modify the value of the reference variable intentionally or unintentionally.
Default parameters
Default parameters allow a function to be called without specifying all parameters. This simplifies function calls and provides useful default behavior. For example:
int sum(int a, int b = 0) { return a + b; }
Inline function
Inline function embeds the function calling code directly into the call point. This reduces function call overhead but may increase code size. Generally speaking, only small, frequently called functions are suitable for inlining.
To make a function inline, you can use inline
Keywords:
inline double calculate_area(double radius) { return 3.14159 * radius * radius; }
Constant expression
Constant expression is an expression that is evaluated at compile time. Representing constants as выражения в функции can improve code readability and ensure the correctness of expressions. For example:
const double PI = 3.14159; double calculate_area(double radius) { return PI * radius * radius; }
Exception handling
The exception handling mechanism allows functions to report errors without terminating the program. Using exceptions can make your code more robust and simplify error handling.
To throw an exception, you can use throw
Keyword:
void validate_input(int value) { if (value < 0) { throw std::invalid_argument("Value must be non-negative"); } }
Practical case
Consider a function that calculates the determinant of a matrix:
double calculate_determinant(std::vector<std::vector<double>> matrix) { double result = 0; // ... 复杂的逻辑 ... return result; }
To optimize this function, we can apply the above tips:
- Function naming: Explicitly name the function
calculate_matrix_determinant()
to reflect its use. - Default parameters: Add a default parameter that takes the identity matrix as an input parameter to simplify the calculation of the determinant of the identity matrix.
- Constant expression: Use floating point constant expression to represent π.
- Exception handling: If the matrix is not a square matrix or is not invertible, throw an exception to report an error.
The optimized function looks like this:
double calculate_matrix_determinant(std::vector<std::vector<double>> matrix, bool is_identity = false) { if (!is_identity) { // 验证矩阵是否为方阵 for (int i = 0; i < matrix.size(); i++) { if (matrix[i].size() != matrix.size()) { throw std::invalid_argument("Matrix must be square"); } } } const double PI = 3.14159; double result = 0; // ... 复杂的逻辑 ... return result; }
By applying these optimization techniques, we improve the readability, maintainability and robustness of the function.
The above is the detailed content of Detailed explanation of C++ function optimization: How to optimize code readability and maintainability?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










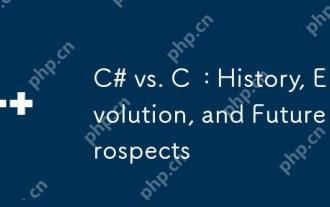
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
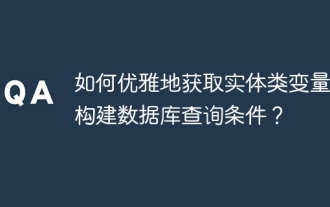
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
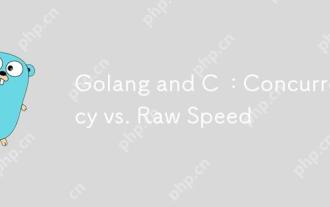
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
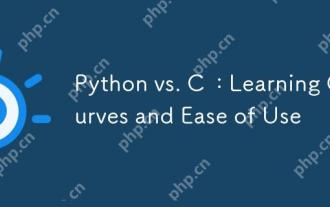
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
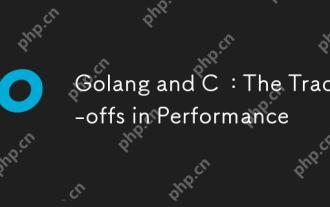
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
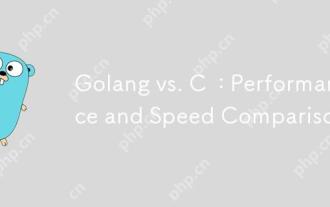
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
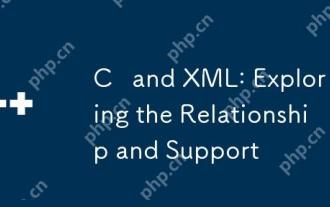
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
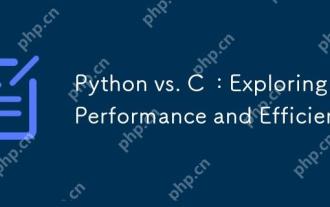
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
