


In-depth analysis of C++ virtual functions: type erasure and polymorphic implementation
C virtual functions implement polymorphism and separate object type information through type erasure so that the compiler only recognizes public interfaces. The virtual pointer table stores virtual function addresses. When the base class pointer points to a derived class object, the derived class pointer pointing to the derived class virtual pointer table will replace the virtual pointer table pointed to by the base class pointer, thus achieving polymorphism.
In-depth analysis of C virtual functions: type erasure and polymorphic implementation
In object-oriented programming (OOP), polymorphism State is a crucial idea, which allows us to use a set of public interfaces to operate objects of different classes. The C language implements polymorphism through virtual functions, which separate type information from objects and allow us to deal with different object types in a common way.
Type erasure
When the compiler encounters a virtual function, it type-erases it, which means it removes the object's type information . Therefore, when a base class pointer points to a derived class object, the compiler no longer knows the exact type of the object. Instead, it only knows about the object's public interface, which is the base class interface.
Polymorphic implementation
C virtual functions are implemented through a method table called a virtual pointer. Each class has a virtual pointer table (VTABLE), which lists the addresses of all virtual functions of the class. When a base class pointer points to a derived class object, the compiler replaces the original virtual pointer table pointed by the base class pointer with a derived class pointer pointing to a derived class VTABLE.
Practical Case
The following is an example of a C virtual function, which shows the application of type erasure and polymorphism in practice:
#include <iostream> class Shape { public: virtual void draw() = 0; // 纯虚函数 }; class Rectangle : public Shape { public: void draw() override { std::cout << "Drawing a rectangle" << std::endl; } }; class Circle : public Shape { public: void draw() override { std::cout << "Drawing a circle" << std::endl; } }; int main() { Shape* shapes[] = {new Rectangle(), new Circle()}; // 类型擦除: 数组中包含不同类型的 Shape 对象 for (Shape* shape : shapes) { shape->draw(); // 多态: 无论对象的实际类型如何,都会调用正确的 draw() 方法 } return 0; }
In this example, we define a Shape
base class and two derived classes Rectangle
and Circle
. Shape
The base class contains a pure virtual function draw()
, and the derived class implements this function. In the main()
function, we create an array of Shape
pointers pointing to the Rectangle
and Circle
objects. Since the draw()
function is a virtual function, when we call draw()
through the base class pointer, it will call the draw()
method of the derived class , thus achieving polymorphism.
By understanding the type erasure and polymorphic implementation principles of virtual functions, we can have a deeper understanding of the core mechanism of OOP. This allows us to design flexible and extensible programs that can handle a variety of different object types.
The above is the detailed content of In-depth analysis of C++ virtual functions: type erasure and polymorphic implementation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










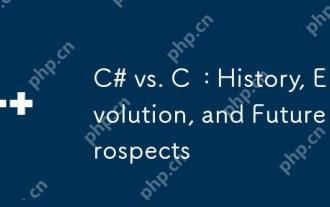
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
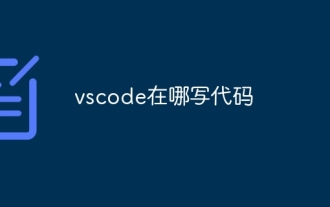
Writing code in Visual Studio Code (VSCode) is simple and easy to use. Just install VSCode, create a project, select a language, create a file, write code, save and run it. The advantages of VSCode include cross-platform, free and open source, powerful features, rich extensions, and lightweight and fast.
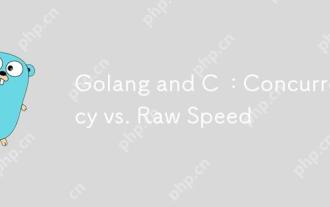
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
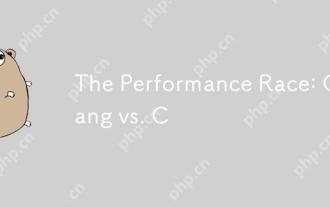
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
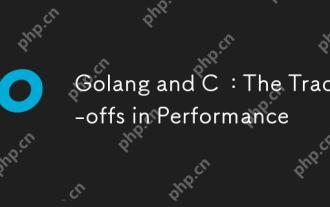
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
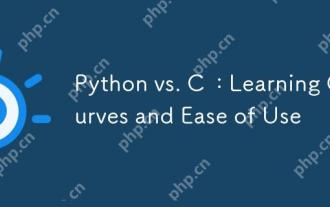
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
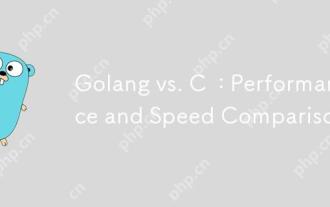
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
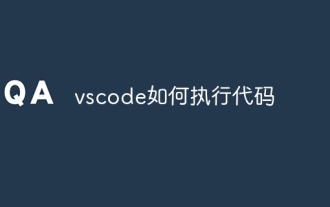
Executing code in VS Code only takes six steps: 1. Open the project; 2. Create and write the code file; 3. Open the terminal; 4. Navigate to the project directory; 5. Execute the code with the appropriate commands; 6. View the output.
