How do C++ functions handle errors gracefully?
Techniques for handling errors in C functions include: exception handling, noexcept specification, return error code, standard return value and custom exception. Exception handling provides a reliable means of catching and handling errors, and the noexcept specification indicates that functions will not throw exceptions. By returning an error code or enumeration value, the caller can check the error status. In some cases, standard return values such as nullptr are used to indicate errors. For custom errors, exception classes can be defined to provide more specific information.
Tips for gracefully handling errors in C functions
When writing robust code in C, handle errors gracefully to It's important. By employing the following techniques, you can ensure that your functions continue to work properly when errors are encountered.
1. Exception handling
Using try-catch
blocks to catch and handle errors is a common method. You can specify the types of errors to catch and provide an error handler to handle them.
try { // 代码可能抛出异常的地方 } catch (const std::exception& e) { // 处理错误 }
2. noexcept
Specification
If you are sure that the function will not throw any exceptions, you can use the noexcept
specification . This will tell the compiler that the function will not throw an exception and allow certain optimizations to be done.
int safe_function() noexcept { // 函数保证不会抛出异常 }
3. Return error code
Another way to handle errors is to return an error code or enumeration value. Callers can check this value to determine whether the function executed successfully and any errors that occurred.
enum class ErrorCodes { Success, InvalidInput, IOError }; ErrorCodes do_something() { // 函数执行并返回错误码 }
4. Standard return values
For some situations, it may be appropriate to use standard return values to indicate errors. For example, in a find operation, a nullptr
can be returned to indicate that the item was not found.
Item* find_item(const std::string& name) { // 函数返回一个指针,指向找到的项目,或 nullptr 如果未找到 }
5. Custom exceptions
For complex or custom errors, you can define a custom exception class. This allows you to provide more specific information about the error and simplify error handling.
class MyException : public std::exception { public: MyException(const std::string& message) : message_(message) {} const char* what() const noexcept override { return message_.c_str(); } private: std::string message_; };
Practical case:
Consider a function that reads numbers from a file. This function may throw an exception if the file does not exist or the data format is invalid. We can also use error codes to indicate other errors, such as file corruption or access denied.
#include <fstream> #include <stdexcept> using namespace std; enum class ErrorCodes { Success, FileNotFound, InvalidDataFormat, FileAccessDenied }; int read_number_from_file(const string& filename) { ifstream file(filename); if (!file.is_open()) { return ErrorCodes::FileNotFound; } int number; file >> number; if (file.fail()) { return ErrorCodes::InvalidDataFormat; } return number; }
When calling this function, the caller can check the error code returned to determine whether the number was successfully read, and any errors that occurred. This allows the caller to take appropriate action based on the error.
The above is the detailed content of How do C++ functions handle errors gracefully?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










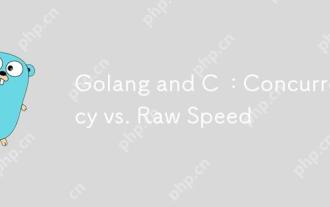
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
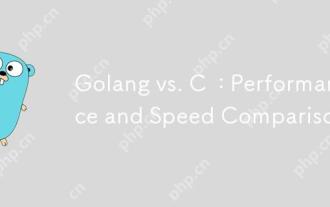
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
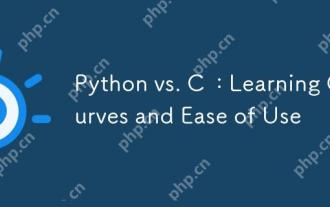
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
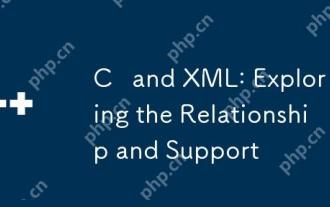
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
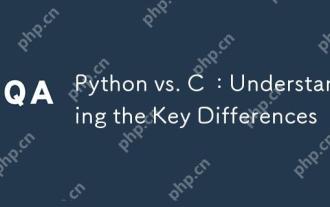
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
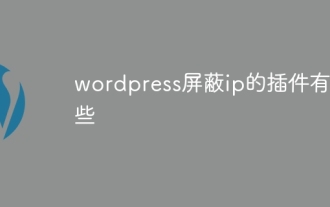
WordPress IP blocking plugin selection is crucial. The following types can be considered: based on .htaccess: efficient, but complex operation; database operation: flexible, but low efficiency; firewall: high security performance, but complex configuration; self-written: highest control, but requires more technical level.
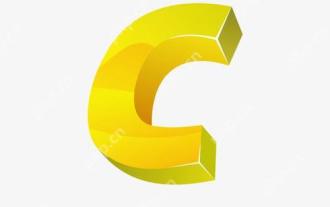
The application of static analysis in C mainly includes discovering memory management problems, checking code logic errors, and improving code security. 1) Static analysis can identify problems such as memory leaks, double releases, and uninitialized pointers. 2) It can detect unused variables, dead code and logical contradictions. 3) Static analysis tools such as Coverity can detect buffer overflow, integer overflow and unsafe API calls to improve code security.
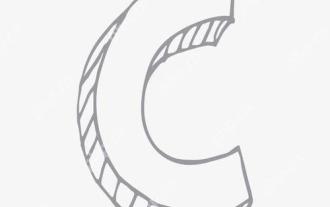
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
