What are the common problems and solutions for Java security mechanisms?
Common problems with Java security mechanisms include credential leakage, SQL injection, cross-site scripting attacks, client-side code injection, and unauthorized access. Solutions include: 1. Using a secure credential management system and RBAC; 2. Using prepared statements and RBAC; 3. Output encoding of user input, implementing CSP and validating HTML input; 4. Using security frameworks, input validation and access Limitations; 5. Implement RBAC, SSO and CAPTCHA or two-factor authentication. Practical case: Use PreparedStatement to prevent SQL injection.
Common problems and solutions for Java security mechanisms
Java security mechanisms are designed to protect applications and systems from security attacks . However, in actual development and deployment, you may encounter some common problems. This article describes these issues and provides practical solutions.
Problem 1: Credential leakage
Solution:
- Use a secure credential management system (such as HashiCorp Vault or AWS Secrets Manager) .
- Implement role-based access control (RBAC) to restrict access to sensitive information and systems.
Problem 2: SQL injection
Solution:
- Use prepared statements or parameterized queries to prevent SQL injection .
- Restrict access to the database and only grant necessary permissions.
Issue 3: Cross-site scripting (XSS) attack
Solution:
- Encode user input for output ( HTML, JavaScript, etc.).
- Implement Content Security Policy (CSP) to restrict script execution from external sources.
- Validate and sanitize HTML input, removing malicious code.
Problem 4: Client Code Injection
Solution:
- Use a security framework such as Spring Security or Apache Shiro ) to restrict access to sensitive APIs.
- Limit the impact of client-side code on server-side logic through input validation and access restrictions.
Issue 5: Unauthorized Access
Solution:
- Implement Role-Based Access Control (RBAC), Restrictions Access to sensitive resources.
- Implement single sign-on (SSO) to reduce the risk of credential theft.
- Use verification code or two-factor authentication to prevent brute force attacks.
Practical case: Preventing SQL injection
import java.sql.*; public class PreventSQLInjection { public static void main(String[] args) { // PreparedStatement 使用占位符来防止 SQL 注入 String sql = "SELECT * FROM users WHERE username = ? AND password = ?"; try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/database", "user", "password"); PreparedStatement statement = conn.prepareStatement(sql)) { statement.setString(1, "username"); statement.setString(2, "password"); ResultSet rs = statement.executeQuery(); // 处理结果集... } catch (SQLException e) { e.printStackTrace(); } } }
The above is the detailed content of What are the common problems and solutions for Java security mechanisms?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
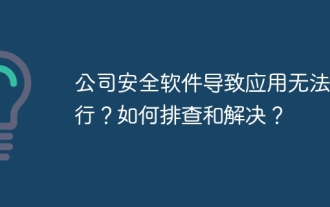
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
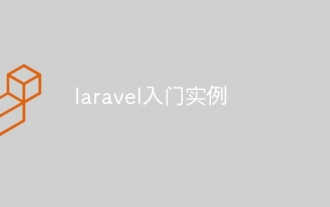
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
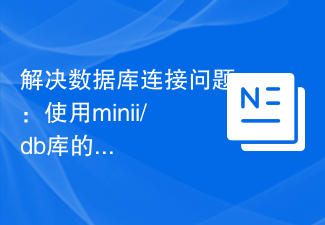
I encountered a tricky problem when developing a small application: the need to quickly integrate a lightweight database operation library. After trying multiple libraries, I found that they either have too much functionality or are not very compatible. Eventually, I found minii/db, a simplified version based on Yii2 that solved my problem perfectly.
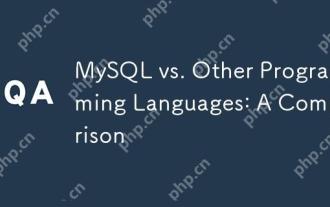
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
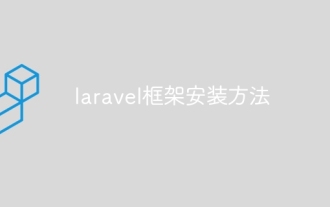
Article summary: This article provides detailed step-by-step instructions to guide readers on how to easily install the Laravel framework. Laravel is a powerful PHP framework that speeds up the development process of web applications. This tutorial covers the installation process from system requirements to configuring databases and setting up routing. By following these steps, readers can quickly and efficiently lay a solid foundation for their Laravel project.
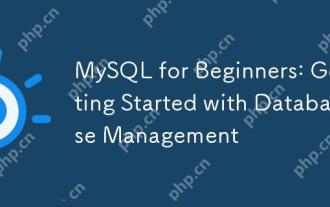
The basic operations of MySQL include creating databases, tables, and using SQL to perform CRUD operations on data. 1. Create a database: CREATEDATABASEmy_first_db; 2. Create a table: CREATETABLEbooks(idINTAUTO_INCREMENTPRIMARYKEY, titleVARCHAR(100)NOTNULL, authorVARCHAR(100)NOTNULL, published_yearINT); 3. Insert data: INSERTINTObooks(title, author, published_year)VA

MySQL and phpMyAdmin are powerful database management tools. 1) MySQL is used to create databases and tables, and to execute DML and SQL queries. 2) phpMyAdmin provides an intuitive interface for database management, table structure management, data operations and user permission management.
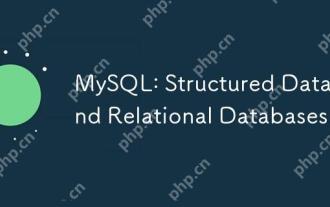
MySQL efficiently manages structured data through table structure and SQL query, and implements inter-table relationships through foreign keys. 1. Define the data format and type when creating a table. 2. Use foreign keys to establish relationships between tables. 3. Improve performance through indexing and query optimization. 4. Regularly backup and monitor databases to ensure data security and performance optimization.
