Best practices and anti-patterns in PHP function calls
Best practices: 1. Use namespaces and aliases to reduce redundancy. 2. Use optional parameters to increase flexibility. 3. Perform parameter type checking to enhance robustness. Anti-patterns: 1. Abuse of aliases and duplicate namespaces. 2. Lack of type checking reduces reliability.
Best practices and anti-patterns in PHP function calls
Best practices
-
Use namespaces : Use the
use
statement to reduce the complete namespace of function calls and improve code readability and maintainability.
use App\Classes\MyClass; MyClass::myMethod();
- Use aliases: Use the
as
keyword to create function aliases to simplify long function names and reduce code redundancy.
function fullFunctionName() { // ... } $fn = 'fullFunctionName' as; $fn();
- Use optional parameters: Define optional function parameters by specifying default values to make the call more flexible.
function myFunction($param1, $param2 = 'default') { // ... } myFunction('value1');
- Parameter type checking: Use type hints to check the data type of incoming parameters to enhance code robustness.
function myFunction(int $param1, string $param2) { // ... }
Anti-pattern
- Duplicate fully qualified name: Do not write out the full namespace repeatedly in a function call because it will give the code Adds redundancy and reduces readability.
\Namespace\Subnamespace\Class\method(); // AVOID
- Abuse of aliases: Avoid overuse of aliases as it may confuse the code and reduce maintainability.
// AVOID: Creates ambiguous function calls function f1() { // ... } function f2() { // ... } $f = f1' as; $f(); // Which function is called?
- Lack of parameter type checking: Failure to perform parameter type checking will lead to potential data type errors and reduce the reliability and maintainability of the code.
function myFunction($param) { // ... } myFunction([]); // May throw an error if $param is not an array
Practical Case
Consider the following code snippet:
namespace App\Controllers; use App\Models\User; class UserController { public function index() { $users = User::all(); return view('users.index', compact('users')); } }
Best Practice:
- Use
namespace
statement imports theUserController
namespace. - Use the
use
statement to import theUser
model.
Anti-pattern:
- Repeatedly writing the
App\Models\User
namespace. - The
use
statement was not used to import theUser
model.
The above is the detailed content of Best practices and anti-patterns in PHP function calls. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
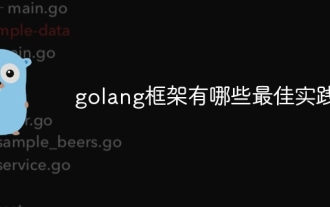
When using Go frameworks, best practices include: Choose a lightweight framework such as Gin or Echo. Follow RESTful principles and use standard HTTP verbs and formats. Leverage middleware to simplify tasks such as authentication and logging. Handle errors correctly, using error types and meaningful messages. Write unit and integration tests to ensure the application is functioning properly.
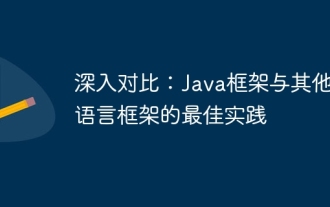
Java frameworks are suitable for projects where cross-platform, stability and scalability are crucial. For Java projects, Spring Framework is used for dependency injection and aspect-oriented programming, and best practices include using SpringBean and SpringBeanFactory. Hibernate is used for object-relational mapping, and best practice is to use HQL for complex queries. JakartaEE is used for enterprise application development, and the best practice is to use EJB for distributed business logic.
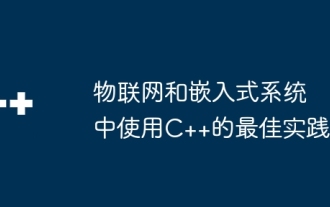
Introduction to Best Practices for Using C++ in IoT and Embedded Systems C++ is a powerful language that is widely used in IoT and embedded systems. However, using C++ in these restricted environments requires following specific best practices to ensure performance and reliability. Memory management uses smart pointers: Smart pointers automatically manage memory to avoid memory leaks and dangling pointers. Consider using memory pools: Memory pools provide a more efficient way to allocate and free memory than standard malloc()/free(). Minimize memory allocation: In embedded systems, memory resources are limited. Reducing memory allocation can improve performance. Threads and multitasking use the RAII principle: RAII (resource acquisition is initialization) ensures that the object is released at the end of its life cycle.
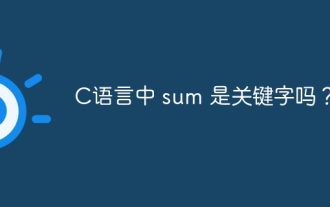
The sum keyword does not exist in C language, it is a normal identifier and can be used as a variable or function name. But to avoid misunderstandings, it is recommended to avoid using it for identifiers of mathematical-related codes. More descriptive names such as array_sum or calculate_sum can be used to improve code readability.
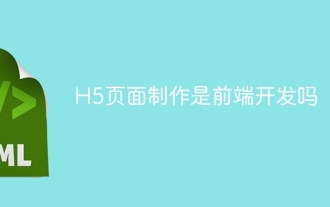
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
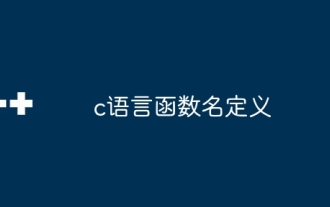
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
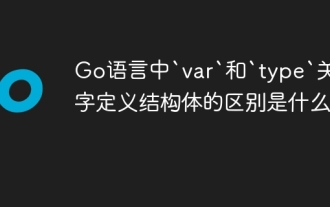
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
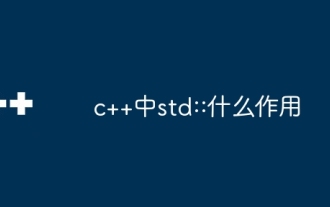
std:: is a namespace in C++ that contains standard library functions, classes, and objects, simplifying software development. Its specific functions include: providing data structure containers, such as vectors and sets; providing iterators for traversing containers; including various algorithms for operating data; providing input/output stream objects for processing I/O operations; providing other practical tools, Such as exception handling and memory management.
