How does Java Servlet handle thread safety and parallel requests?
Java Servlet provides multiple mechanisms to handle thread safety and parallel requests, including: Thread safety: Use ThreadLocal to store synchronization methods Immutable objects Parallel request processing: Use thread pool asynchronous Servlet to optimize database access
Java Servlet handles thread safety and parallel requests
Preface
In high-concurrency web applications, handles thread safety and parallel requests Requests are critical to ensure the application is stable and reliable. Java Servlets provide several mechanisms to manage thread safety and parallel requests.
Thread safety
Thread safety means that a Servlet can run safely in multiple threads at the same time without causing data corruption. The following techniques help achieve thread safety:
- Using ThreadLocal storage: ThreadLocal storage allows private data to be saved in each thread, thus preventing data races.
- Synchronization method: Use the synchronized keyword synchronization method to prevent other threads from accessing shared data at the same time.
- Use immutable objects: immutable objects cannot be modified, so they are thread-safe.
Parallel request processing
The Java Servlet container can handle multiple requests at the same time. The following techniques can help improve parallel request processing:
- Using thread pools: Thread pools manage a collection of threads for processing requests. This is more efficient than creating a new thread for each request.
- Using asynchronous Servlets: Asynchronous Servlets allow tasks to be performed on the request thread while returning control of the request to the container. This helps process requests in parallel.
- Optimize database access: Efficient concurrent access to the database is crucial. Using a connection pool and enabling transaction isolation can minimize conflicts when making parallel requests.
Practical Case
Consider using Servlet to deploy a simple shopping cart application. The application needs to manage the shopping cart session and display the shopping cart details to the user. Here's how to manage thread safety and parallel requests:
public class ShoppingCartServlet extends HttpServlet { // 使用 ThreadLocal 存储购物车会话 private ThreadLocal<Map<String, Product>> cart = new ThreadLocal<>(); @Override public doGet(HttpServletRequest req, HttpServletResponse res) { // 获取当前线程的购物车会话 Map<String, Product> cart = this.cart.get(); // ... 处理请求业务逻辑 ... } }
Conclusion
By applying these techniques, Java Servlet developers can create applications that are thread-safe and handle parallel requests efficiently. Thread safety ensures data integrity, while parallel processing increases performance and scalability, providing a reliable and responsive user experience.
The above is the detailed content of How does Java Servlet handle thread safety and parallel requests?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










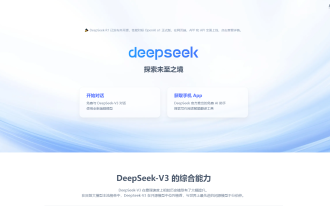
DeepSeek: How to deal with the popular AI that is congested with servers? As a hot AI in 2025, DeepSeek is free and open source and has a performance comparable to the official version of OpenAIo1, which shows its popularity. However, high concurrency also brings the problem of server busyness. This article will analyze the reasons and provide coping strategies. DeepSeek web version entrance: https://www.deepseek.com/DeepSeek server busy reason: High concurrent access: DeepSeek's free and powerful features attract a large number of users to use at the same time, resulting in excessive server load. Cyber Attack: It is reported that DeepSeek has an impact on the US financial industry.
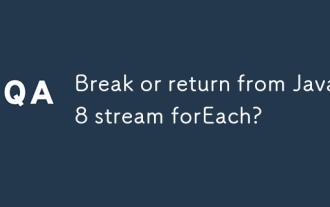
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
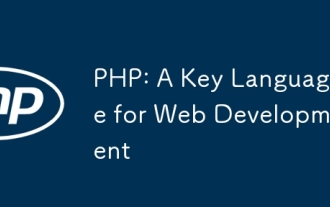
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
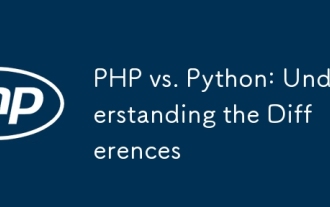
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
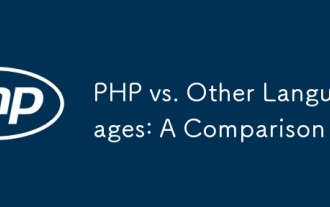
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
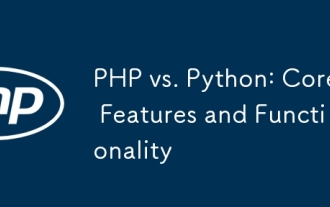
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
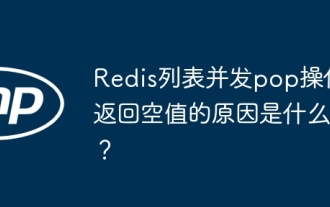
redis...
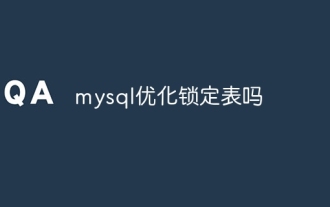
MySQL uses shared locks and exclusive locks to manage concurrency, providing three lock types: table locks, row locks and page locks. Row locks can improve concurrency, and use the FOR UPDATE statement to add exclusive locks to rows. Pessimistic locks assume conflicts, and optimistic locks judge the data through the version number. Common lock table problems manifest as slow querying, use the SHOW PROCESSLIST command to view the queries held by the lock. Optimization measures include selecting appropriate indexes, reducing transaction scope, batch operations, and optimizing SQL statements.
