How to use generics efficiently in Java
Generics in Java can improve code readability and maintainability, and can prevent runtime type errors, thereby enhancing security. Best practices for using generics in Java include limiting the types of generic type parameters, using wildcard types and avoiding overgenericization.
How to use generics efficiently in Java
Generics are a powerful Java feature that allows us to write code that can operate on different types of data. Reuse code. This can greatly improve the readability, maintainability and security of your code.
Syntax
Declare a generic class:
public class Example<T> { // ... code }
Where, <T>
is the placeholder for the type parameter .
Declare generic methods:
public static <T> void printArray(T[] arr) { // ... code }
Use generic methods:
Example<Integer> example = new Example<>(); example.someMethod(10); Example<String> example2 = new Example<>(); example2.someMethod("Hello");
Practical case: Comparator interface
One of the common applications of generics is the Comparable
interface for comparing objects. This interface defines the compareTo
method, which is used to compare the size order of two objects.
We can use generics to create our own comparator class:
public class PersonComparator<T extends Comparable<T>> implements Comparator<T> { @Override public int compare(T o1, T o2) { return o1.compareTo(o2); } }
This generic comparator can be used to compare any type of object that implements the Comparable
interface:
PersonComparator<Person> comparator = new PersonComparator<>(); Collections.sort(people, comparator);
Generics and Security
Using generics can enhance the security of your code. When we use generics, the Java compiler checks the type safety of the type parameters. This helps us avoid runtime type errors, thereby improving the reliability of our code.
Generics and Efficiency
Using generics usually does not have a significant impact on performance. The Java compiler optimizes generic code to eliminate runtime type checking. However, in some cases, generic code may be slightly slower than non-generic code.
Best Practices
To use generics effectively, here are some best practices:
- Restricting Generic Types: By Restricting With the type of generic type parameters, we can improve the readability and reliability of the code.
-
Use wildcard types: Wildcard types (such as
?
and? extends T
) can represent a wide range of types, thereby increasing the flexibility of your code . - Avoid excessive genericization: Excessive genericization may make the code difficult to understand and maintain.
Conclusion
Generics are a powerful feature in Java that can be used to write reusable, efficient and safe code. By following the above best practices, we can use generics effectively to improve code quality.
The above is the detailed content of How to use generics efficiently in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
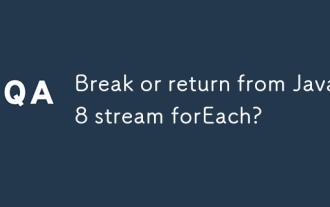
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
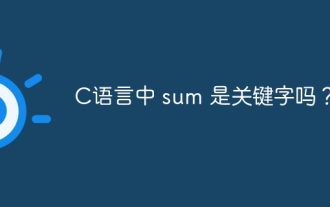
The sum keyword does not exist in C language, it is a normal identifier and can be used as a variable or function name. But to avoid misunderstandings, it is recommended to avoid using it for identifiers of mathematical-related codes. More descriptive names such as array_sum or calculate_sum can be used to improve code readability.
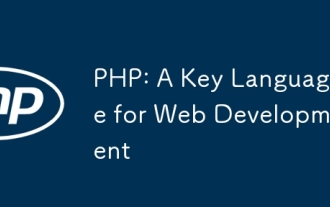
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
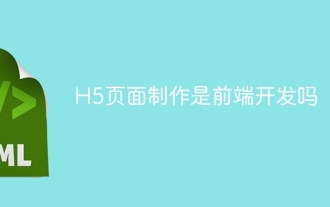
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
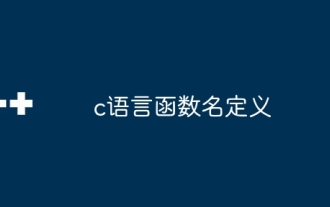
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
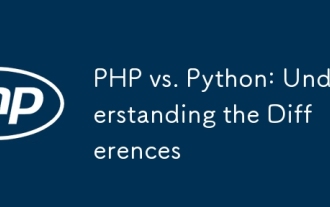
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
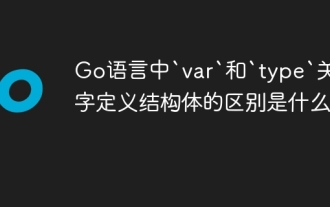
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
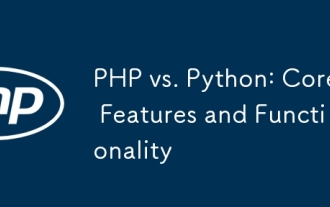
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
