


In-depth discussion of the impact of Golang slicing out of bounds and countermeasures
Slice in Golang is a flexible and convenient data structure that can be dynamically adjusted in size to facilitate data cutting and management. However, out-of-bounds slice access is a common programming error that can cause a program to crash or produce unexpected results. This article will delve into the impact of Golang slicing out of bounds and countermeasures, and provide specific code examples.
The impact of slice out-of-bounds
When we try to access an index in a slice that exceeds its length range, it will cause slice out-of-bounds. This may cause the following problems:
- Program crash: Slice out-of-bounds access may cause the program to crash and output an error message similar to "slice bounds out of range" on the console.
- Memory leak: In some cases, a slice may continue to use unexpected memory space after an out-of-bounds access, causing memory leak problems.
- Data corruption: Out-of-bounds access to slices may overwrite other memory areas, causing program data corruption.
Code example
The following is a simple example of slice out-of-bounds access:
package main import "fmt" func main() { s := []int{1, 2, 3} // Attempt to access index beyond slice length fmt.Println(s[3]) }
In the above code, we create an integer slice [1, 2, 3]
with three elements and then try to access the element with index 3. Since the slice length is 3, accessing the element with index 3 will cause the slice to go out of bounds.
Countermeasures
To avoid slice out-of-bounds issues, follow these best practices:
- Check the boundaries: Before accessing the slice , always first check whether the index to be accessed is within the legal range.
- Use built-in functions: Use built-in functions such as
len
to get the length of the slice and avoid manually calculating the length. - Note to avoid modifying the length: When adding or removing elements via a slice, be sure to update the length of the slice to avoid going out of bounds.
- Be careful when passing slices: When passing slices between functions, make sure that the passed slices will not be modified inside the function and cause out-of-bounds.
Code Example
The following is a sample code that demonstrates how to avoid out-of-bounds access to slices:
package main import "fmt" func main() { s := []int{1, 2, 3} index := 3 if index < len(s) { fmt.Println(s[index]) } else { fmt.Println("Index out of range") } }
In the above code example, we first check whether the index to be accessed is less than the length of the slice to ensure that out-of-bounds access is avoided before accessing the slice element.
Summary
Slicing is a commonly used data structure in Golang, but out-of-bounds access to slices may cause serious problems. By following best practices and paying attention to details, you can effectively avoid the problem of out-of-bounds slicing and ensure the stability and reliability of your code. I hope the content of this article can help readers better understand the impact of Golang slicing out of bounds and the countermeasures.
The above is the detailed content of In-depth discussion of the impact of Golang slicing out of bounds and countermeasures. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
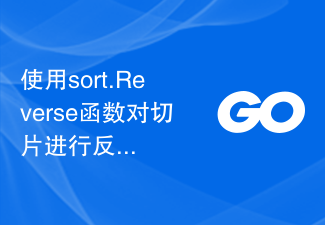
Use the sort.Reverse function to reverse sort a slice. In the Go language, a slice is an important data structure that can dynamically increase or decrease the number of elements. When we need to sort slices, we can use the functions provided by the sort package to perform sorting operations. Among them, the sort.Reverse function can help us reverse sort the slices. The sort.Reverse function is a function in the sort package. It accepts a sort.Interface interface type.
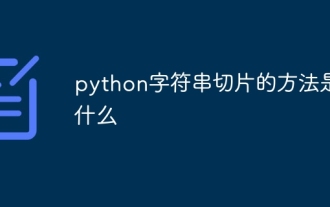
In Python, you can use string slicing to get substrings in a string. The basic syntax of string slicing is "substring = string[start:end:step]".

Video slicing authorization refers to the process of dividing video files into multiple small fragments and authorizing them in video services. This authorization method can provide better video fluency, adapt to different network conditions and devices, and protect the security of video content. Through video slicing authorization, users can start playing videos faster and reduce waiting and buffering times. Video slicing authorization can dynamically adjust video parameters according to network conditions and device types to provide the best playback effect. Video slicing authorization also helps protect The security of video content prevents unauthorized users from piracy and infringement.

There are three methods to remove slice elements in Go language: append function (not recommended), copy function and manually modifying the underlying array. The append function can delete tail elements, the copy function can delete middle elements, and manually modify the underlying array to directly assign and delete elements.
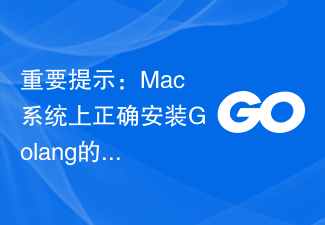
A must-read for Mac users: How to correctly install Golang on your computer? Go language (Golang) is an open source programming language developed by Google. It has the characteristics of efficiency, simplicity, concurrency, etc., and is suitable for developing various types of applications. If you are a Mac user and want to start learning and using the Go language, it is crucial to correctly install Golang to your computer. Through this article, you will learn how to correctly install and configure the Go language environment on Mac, and conduct simple tests to help you smoothly
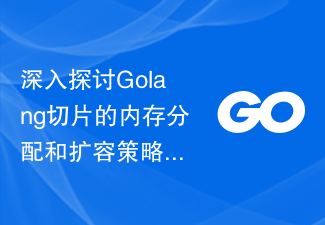
In-depth analysis of Golang slicing principle: memory allocation and expansion strategy Introduction: Slicing is one of the commonly used data types in Golang. It provides a convenient way to operate continuous data sequences. When using slices, it is important to understand its internal memory allocation and expansion strategies to improve program performance. In this article, we will provide an in-depth analysis of the principles of Golang slicing, accompanied by specific code examples. 1. Memory structure and basic principles of slicing In Golang, slicing is a reference type to the underlying array.
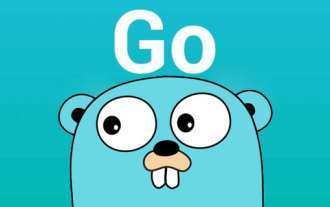
Modification method: 1. Use the append() function to add new values, the syntax is "append(slice, value list)"; 2. Use the append() function to delete elements, the syntax is "append(a[:i], a[i+N" :]...)"; 3. Reassign the value directly according to the index, the syntax is "slice name [index] = new value".
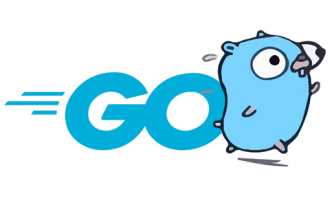
This article will teach you about Golang and talk about slices in the basics of Go language. I hope it will be helpful to you.
