Common PHP error level types and handling techniques
PHP is a commonly used server-side scripting language that is widely used in the field of web development. In the process of PHP programming, various errors are often encountered. These errors are mainly divided into different error levels, including Notice, Warning, Fatal error, etc. Understanding common error level types and corresponding handling techniques is crucial to improving code quality and development efficiency.
1. Types of error levels
- Notice (Note)
Notice is the mildest error level, which usually indicates that there are some potential problems in the code, but will not cause the program to Interrupt. For example, accessing undefined variables, using undefined functions, etc. - Warning
Warning level errors indicate that there are some serious problems, but will not cause the program to interrupt. For example, including files that do not exist, using expired functions, etc. - Fatal error (fatal error)
Fatal error is the most serious error level, indicating that there is a serious problem that prevents the program from continuing to execute, usually causing the program to interrupt. For example, syntax errors, calling non-existent classes, etc.
2. Processing skills
- Error logging
In PHP, you can help developers debug better by setting the error reporting level and error logging code. Use the error_reporting() function to set the required error level and record the error information to the log file.
error_reporting(E_ALL); ini_set('display_errors', 0); ini_set('log_errors', 1); ini_set('error_log', 'error.log');
- Exception handling
Use the try...catch block to capture and handle exceptions that may occur in the code, thereby preventing the program from being interrupted due to errors. Exception handling provides more granular control and error message display.
try { // 可能会出现异常的代码块 } catch (Exception $e) { // 异常处理代码 echo 'Caught exception: ' . $e->getMessage(); }
- Parameter checking
When writing a function or method, you should check and verify the legality of the input parameters in a timely manner to avoid errors caused by passing in illegal parameters. Parameter checking can be done using conditional statements or the assert() function.
function divide($numerator, $denominator) { if ($denominator == 0) { throw new Exception('Division by zero'); } return $numerator / $denominator; }
- Error handling function
By setting a custom error handling function, you can better control the error handling logic when the program is running. You can use the set_error_handler() function to register a custom error handling function.
function customErrorHandler($errno, $errstr, $errfile, $errline) { // 自定义错误处理逻辑 } set_error_handler('customErrorHandler');
- Debugging tools
Using debugging tools such as Xdebug, Zend Debugger, etc. can improve the efficiency of code debugging and help developers quickly locate and solve problems.
To sum up, understanding the common types of PHP error levels and handling techniques is crucial for developers in their daily work. By properly setting error reporting levels, recording error logs, exception handling, and parameter checking, you can effectively improve code quality, reduce the possibility of errors, and thereby improve development efficiency. By continuously accumulating experience and practice, developers can better handle various PHP errors and write high-quality code.
The above is the detailed content of Common PHP error level types and handling techniques. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










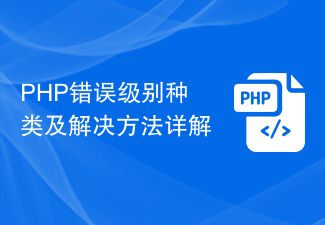
Detailed explanation of PHP error level types and solutions. As a commonly used server-side scripting language, PHP will inevitably encounter various errors during the development process. Understanding PHP error level types and corresponding solutions is crucial to improving development efficiency and code quality. This article will discuss PHP error level types and solutions in detail, and provide specific code examples. PHP error levels are mainly divided into three types: fatal errors, runtime errors and warning errors. Different levels of errors correspond to different handling methods. Below we discuss each error one by one.
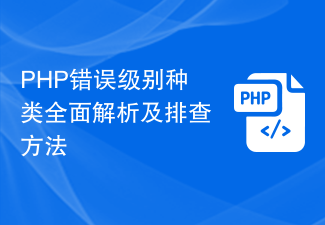
Title: Comprehensive analysis of PHP error level types and troubleshooting methods During the PHP development process, we often encounter various errors. It is very important to understand the types of PHP error levels and the corresponding troubleshooting methods. This article will provide a comprehensive analysis of PHP error level types and provide specific code examples to help you better understand and handle these errors. 1. Types of PHP error levels PHP error levels are divided into the following types: E_ERROR (1): fatal error, causing the script to terminate execution. E_WARNING(
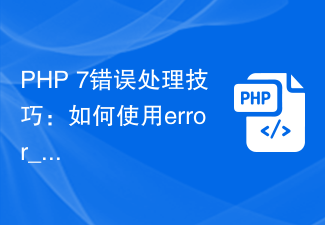
PHP7 error handling tips: How to use the error_reporting function to set the error reporting level In PHP development, error handling is a very important part. Properly setting the error reporting level can help us discover and solve problems in the program in a timely manner, and improve the stability and security of the program. In PHP7, we can use the error_reporting function to set the error reporting level. This article describes how to use this function to handle errors flexibly. In PHP7, error
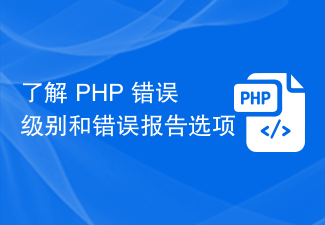
Understand PHP error levels and error reporting options When writing PHP programs, you often encounter various errors, including syntax errors, runtime errors, etc. In order to be able to detect and debug these errors in a timely manner, it is important to understand PHP error levels and error reporting options. This article will detail PHP's error levels and how to set error reporting options. PHP error levels PHP defines different error levels to represent the severity of the error. These error levels are represented by the following constants: E_ERROR: Cause
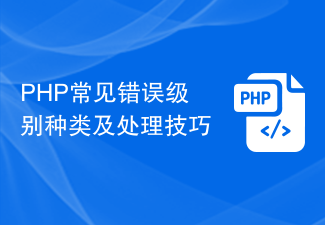
PHP is a commonly used server-side scripting language that is widely used in the field of web development. In the process of PHP programming, various errors are often encountered. These errors are mainly divided into different error levels, including Notice, Warning, Fatalerror, etc. Understanding common error level types and corresponding handling techniques is crucial to improving code quality and development efficiency. 1. Error level types Notice (Note) Notice is the mildest error level, usually indicating that there is an error in the code.

How to deal with code optimization errors in PHP? When writing PHP code, we often encounter some errors, such as syntax errors, logic errors or performance issues. In order to improve the quality and efficiency of the code, we need to learn to deal with these errors and perform code optimization. Some common error handling and code optimization techniques are detailed below. Using error reporting levels PHP provides a series of error reporting levels for setting which errors will be displayed and recorded when the code is running. During the development phase, you can set the error reporting level to E_A
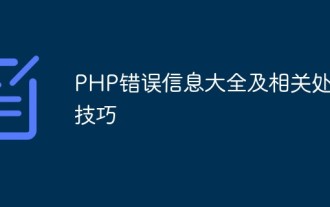
PHP is a very popular server-side scripting language that is widely used in web development. However, like any programming language, various errors and exceptions may occur during PHP development. During the development process, it is very critical to handle these errors and exceptions correctly, otherwise it may have an impact on the performance and security of the web application. This article will introduce some common PHP error messages and related handling techniques to help PHP developers debug and optimize web applications more effectively. one.
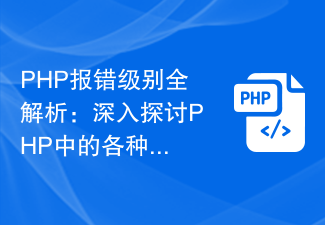
"Full Analysis of PHP Error Levels: In-depth discussion of various error levels in PHP, specific code examples are required" PHP is a commonly used server-side scripting language, and various errors often occur during the development process. In order to better debug and optimize your code, it is very important to understand the different error levels in PHP. This article will delve into the various error levels in PHP and use specific code examples to help readers better understand and respond to various error situations. E_ERROR - Fatal error E_ERROR is the most common error in PHP
