


Decoding Laravel performance bottlenecks: Optimization techniques fully revealed!
Decoding Laravel performance bottlenecks: Optimization techniques fully revealed!
Laravel, as a popular PHP framework, provides developers with rich functions and a convenient development experience. However, as the size of the project increases and the number of visits increases, we may face the challenge of performance bottlenecks. This article will delve into Laravel performance optimization techniques to help developers discover and solve potential performance problems.
1. Database query optimization
- Use Eloquent to delay loading
When using Eloquent to query the database, avoid frequent related queries. You can use lazy loading to load relevant models when needed to reduce the number of database queries and improve performance.
$users = User::all(); foreach ($users as $user) { echo $user->profile->name; // 延迟加载 profile 模型 }
- Use native query
In some complex query scenarios, you can consider using native SQL query to avoid the performance overhead of Eloquent. At the same time, pay attention to using prepared statements to prevent SQL injection.
$users = DB::select('SELECT * FROM users WHERE name = ?', ['John']);
- Avoid N 1 query problems
When multiple related models need to be loaded, to avoid N 1 query problems, you can use the with method to load all related models at once. Improve query efficiency.
$users = User::with('posts')->get();
2. Cache optimization
- Using cache
Laravel has a variety of built-in cache drivers, such as Redis, Memcached, etc. Reasonable use of cache can reduce the number of database queries and speed up data reading.
$users = Cache::remember('users', 60, function () { return User::all(); });
- Cache fragmentation
For some frequently changing page content, you can fragment the cache and only update the part of the content that needs to be changed instead of refreshing the page as a whole .
Cache::forget('users'); // 清除特定缓存
3. Code optimization
- Optimize routing
To avoid defining too many duplicate routes, you can merge similar routes into routing groups to improve Route matching efficiency.
Route::group(['prefix' => 'admin'], function () { Route::get('dashboard', 'AdminController@dashboard'); Route::get('users', 'AdminController@users'); });
- Using queues
Asynchronous tasks can be processed through queues to avoid time-consuming operations blocking threads and improve the concurrent processing capabilities of the program.
dispatch(function () { // 长时间处理任务 });
4. Performance Analysis
Use performance analysis tools, such as Blackfire, Xdebug, etc., to perform performance tuning of the application. By analyzing time-consuming operations, we can optimize performance bottlenecks and improve system response speed.
Route::get('/profile', function () { // Blackfire 性能分析 blackfire()->profile(function () { // 代码逻辑 }); });
To sum up, through database query optimization, cache optimization, code optimization and performance analysis, we can effectively solve the performance bottleneck problems that may arise in Laravel applications. In the actual development process, developers can combine specific business scenarios and data characteristics to adopt corresponding optimization strategies to improve application performance and user experience.
The above is the detailed content of Decoding Laravel performance bottlenecks: Optimization techniques fully revealed!. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
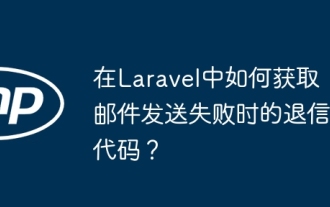
Method for obtaining the return code when Laravel email sending fails. When using Laravel to develop applications, you often encounter situations where you need to send verification codes. And in reality...
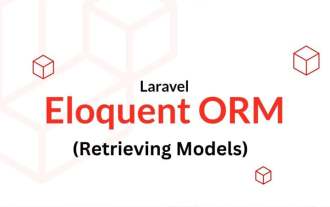
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
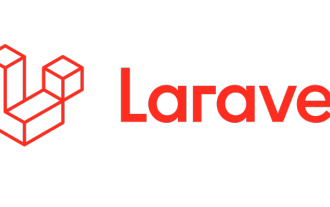
Efficiently process 7 million records and create interactive maps with geospatial technology. This article explores how to efficiently process over 7 million records using Laravel and MySQL and convert them into interactive map visualizations. Initial challenge project requirements: Extract valuable insights using 7 million records in MySQL database. Many people first consider programming languages, but ignore the database itself: Can it meet the needs? Is data migration or structural adjustment required? Can MySQL withstand such a large data load? Preliminary analysis: Key filters and properties need to be identified. After analysis, it was found that only a few attributes were related to the solution. We verified the feasibility of the filter and set some restrictions to optimize the search. Map search based on city
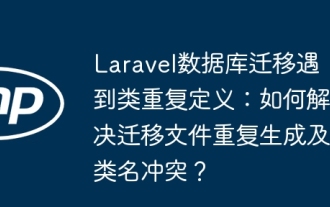
A problem of duplicate class definition during Laravel database migration occurs. When using the Laravel framework for database migration, developers may encounter "classes have been used...
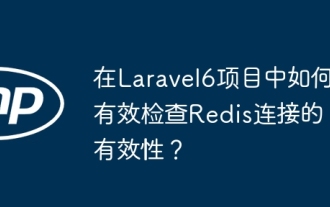
How to check the validity of Redis connections in Laravel6 projects is a common problem, especially when projects rely on Redis for business processing. The following is...

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.
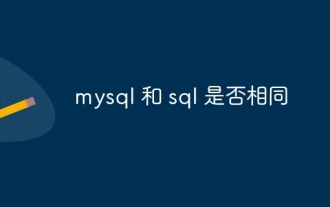
MySQL and SQL are brothers, not twins. SQL is a database query language standard, while MySQL is a relational database management system (RDBMS) that follows the SQL standard. There is the following difference between the two: SQL defines rules for interacting with the database, and MySQL is a concrete implementation of these rules. Standard SQL statements can run on any database system that complies with SQL standards, but may require fine tuning. Functions and syntaxes specific to a specific database system are only applicable to that system, such as the LOAD_FILE() function of MySQL. Learning SQL is essential for operating any database system, while learning MySQL and other specifics
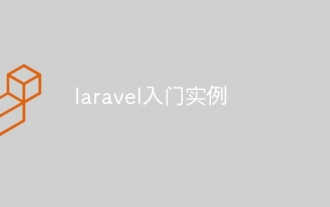
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
