


Demystifying the Python GIL: Exploring and Breaking Down Concurrency Barriers
The principle of Python GIL
python GIL is a mutually exclusive lock, which ensures that only one thread executes Python bytecode at the same time. This is to prevent data inconsistency caused by simultaneous modification of shared data. However, the GIL also imposes limitations on the concurrency and scalability of multithreaded programs.
GIL’s impact on concurrency
Due to the GIL, threads in Python cannot truly execute in parallel. When a thread acquires the GIL, other threads must wait until it releases the GIL. This may cause the following concurrency issues:
- Low concurrency: Due to the existence of GIL, multi-threaded programs in Python cannot take full advantage of multi-core CPUs.
- Deadlock: A deadlock may occur if two threads wait for each other for the GIL.
- Performance degradation: GIL competition will increase the overhead of the program, resulting in performance degradation.
Strategies to Mitigate GIL Challenges
While GIL cannot be completely eliminated, there are several strategies to mitigate the challenges it poses:
1. Multi-process
Since the GIL only applies to threads in the same process, using multiple processes can circumvent the limitations of the GIL. In a multi-process program, each process has its own Python interpreter and GIL, so execution can be truly parallel.
Demo code:
import multiprocessing def worker(num): print(f"Worker {num}: {os.getpid()}") if __name__ == "__main__": pool = multiprocessing.Pool(processes=4) pool.map(worker, range(4))
2. Cython
Cython is a Python extension language that allows Python code to be compiled into C code. Because C code is not restricted by the GIL, Cython can significantly improve the performance of computationally intensive tasks in Python.
Demo code:
import cython @cython.boundscheck(False) @cython.wraparound(False) def fib(int n): if n == 0: return 0 if n == 1: return 1 return fib(n - 1) + fib(n - 2)
3. asyncio
asyncio is an asynchronous framework in Python. It allows coroutines (a type of lightweight thread) to execute in parallel without being restricted by the GIL. Coroutines avoid GIL contention by using an event loop to achieve parallelism.
Demo code:
import asyncio async def hello_world(): print("Hello, world!") async def main(): tasks = [hello_world() for _ in range(4)] await asyncio.gather(*tasks) if __name__ == "__main__": asyncio.run(main())
4. GIL release
GIL release is a Python built-in function that allows a thread to release the GIL within a specified period of time. This can help reduce GIL contention and improve higher concurrency performance.
Demo code:
import time def worker(): with release_gil(): time.sleep(1) threads = [threading.Thread(target=worker) for _ in range(4)] for thread in threads: thread.start() for thread in threads: thread.join()
in conclusion
The Python GIL is a necessary mechanism to prevent data inconsistencies in concurrent data access. However, it also places limitations on Python's concurrency performance. By understanding the principles and impact of the GIL and employing strategies such as multiprocessing, Cython, asyncio, or GIL release, developers can create scalable, high-performance concurrent applications in Python.
The above is the detailed content of Demystifying the Python GIL: Exploring and Breaking Down Concurrency Barriers. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
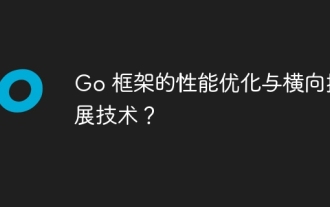
In order to improve the performance of Go applications, we can take the following optimization measures: Caching: Use caching to reduce the number of accesses to the underlying storage and improve performance. Concurrency: Use goroutines and channels to execute lengthy tasks in parallel. Memory Management: Manually manage memory (using the unsafe package) to further optimize performance. To scale out an application we can implement the following techniques: Horizontal Scaling (Horizontal Scaling): Deploying application instances on multiple servers or nodes. Load balancing: Use a load balancer to distribute requests to multiple application instances. Data sharding: Distribute large data sets across multiple databases or storage nodes to improve query performance and scalability.
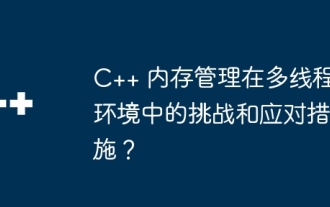
In a multi-threaded environment, C++ memory management faces the following challenges: data races, deadlocks, and memory leaks. Countermeasures include: 1. Use synchronization mechanisms, such as mutexes and atomic variables; 2. Use lock-free data structures; 3. Use smart pointers; 4. (Optional) implement garbage collection.
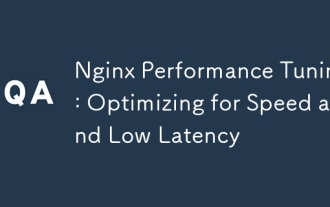
Nginx performance tuning can be achieved by adjusting the number of worker processes, connection pool size, enabling Gzip compression and HTTP/2 protocols, and using cache and load balancing. 1. Adjust the number of worker processes and connection pool size: worker_processesauto; events{worker_connections1024;}. 2. Enable Gzip compression and HTTP/2 protocol: http{gzipon;server{listen443sslhttp2;}}. 3. Use cache optimization: http{proxy_cache_path/path/to/cachelevels=1:2k
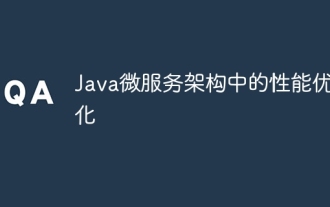
Performance optimization for Java microservices architecture includes the following techniques: Use JVM tuning tools to identify and adjust performance bottlenecks. Optimize the garbage collector and select and configure a GC strategy that matches your application's needs. Use a caching service such as Memcached or Redis to improve response times and reduce database load. Employ asynchronous programming to improve concurrency and responsiveness. Split microservices, breaking large monolithic applications into smaller services to improve scalability and performance.
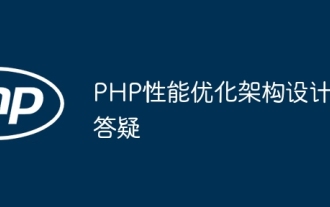
In order to improve the performance of concurrent, high-traffic PHP applications, it is crucial to implement the following architectural optimizations: 1. Optimize PHP configuration and enable caching; 2. Use frameworks such as Laravel; 3. Optimize code to avoid nested loops; 4. Optimize database, Build index; 5. Use CDN to cache static resources; 6. Monitor and analyze performance, and take measures to solve bottlenecks. For example, website user registration optimization successfully handled a surge in user registrations by fragmenting data tables and enabling caching.
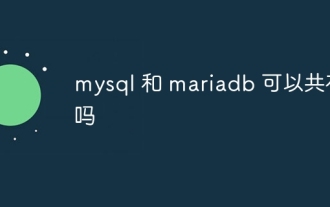
MySQL and MariaDB can coexist, but need to be configured with caution. The key is to allocate different port numbers and data directories to each database, and adjust parameters such as memory allocation and cache size. Connection pooling, application configuration, and version differences also need to be considered and need to be carefully tested and planned to avoid pitfalls. Running two databases simultaneously can cause performance problems in situations where resources are limited.
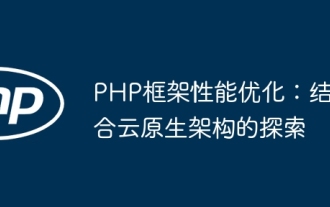
PHP Framework Performance Optimization: Embracing Cloud-Native Architecture In today’s fast-paced digital world, application performance is crucial. For applications built using PHP frameworks, optimizing performance to provide a seamless user experience is crucial. This article will explore strategies to optimize PHP framework performance by combining cloud-native architecture. Advantages of Cloud Native Architecture Cloud native architecture provides some advantages that can significantly improve the performance of PHP framework applications: Scalability: Cloud native applications can be easily scaled to meet changing load requirements, ensuring that peak periods do not occur bottleneck. Elasticity: The inherent elasticity of cloud services allows applications to recover quickly from failures and maintain availability and responsiveness. Agility: Cloud-native architecture supports continuous integration and continuous delivery
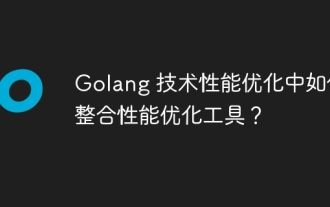
Integrating performance optimization tools into Golang technology performance optimization In Golang applications, performance optimization is crucial, and the efficiency of this process can be greatly improved with the help of performance optimization tools. This article will guide you through the step-by-step integration of popular performance optimization tools to help you conduct comprehensive performance analysis and optimization of your application. 1. Choose performance optimization tools. There are many performance optimization tools to choose from, such as: [pprof](https://github.com/google/pprof): a toolkit developed by Google for analyzing CPU and memory utilization. [go-torch](https://github.com/uber/go-torch):
