


PHP programming tips: How to quickly locate missing numbers in an array
PHP Programming Tips: How to quickly locate missing numbers in an array
In programming, we often encounter situations where we need to check whether certain numbers are missing in an array. At this time, we need a fast and effective way to locate the missing numbers in the array so that they can be processed in a timely manner. This article will introduce a PHP-based programming technique, using specific code examples to demonstrate how to quickly locate missing numbers in an array.
1. Method 1: Use a loop to traverse the array
First, we can check for missing numbers in the array by looping through the array. The specific steps are as follows:
function findMissingNumbers($arr) { $maxNum = max($arr); $minNum = min($arr); $missingNumbers = []; for ($i = $minNum; $i <= $maxNum; $i++) { if (!in_array($i, $arr)) { $missingNumbers[] = $i; } } return $missingNumbers; } // 示例 $array = [1, 3, 5, 7, 9]; $missingNumbers = findMissingNumbers($array); echo "数组中缺失的数字为:" . implode(", ", $missingNumbers);
In the above code, we first find the maximum and minimum values in the array, then loop through the numbers in this range, checking one by one whether they exist in the array, and if they do not exist, Add it to the array of missing numbers.
2. Method 2: Use the difference function array_diff
Another way to quickly locate missing numbers in an array is to use PHP's array_diff function. This function can calculate the difference of arrays to find the difference between two arrays.
function findMissingNumbers($arr) { $maxNum = max($arr); $minNum = min($arr); $fullArray = range($minNum, $maxNum); $missingNumbers = array_diff($fullArray, $arr); return $missingNumbers; } // 示例 $array = [1, 3, 5, 7, 9]; $missingNumbers = findMissingNumbers($array); echo "数组中缺失的数字为:" . implode(", ", $missingNumbers);
In this code, we first use the range function to generate a complete array of numbers, and then use the array_diff function to calculate the difference between the complete array and the given array, which is the missing number.
To summarize, the above are two commonly used methods to quickly locate missing numbers in an array. Choose the appropriate method according to the specific situation to quickly and effectively check for missing numbers in the array and process them in a timely manner. I hope this article will be helpful to your practice in PHP programming!
The above is the detailed content of PHP programming tips: How to quickly locate missing numbers in an array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
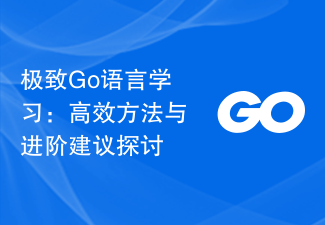
Ultimate Go Language Learning: Discussion of Efficient Methods and Advanced Suggestions In today's era of rapid development of information technology, full-stack development has become a trend, and Go language, as an efficient, concise and powerful programming language with strong concurrency performance, is highly developed. favored by readers. However, in order to truly master the Go language, one must not only be familiar with its basic syntax and common library functions, but also need to have an in-depth understanding of its design principles and advanced features. This article will discuss how to improve the learning efficiency of Go language through efficient methods and advanced suggestions, and deepen understanding through specific code examples.
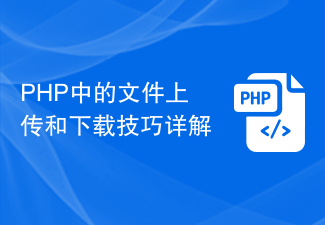
PHP is a very popular server-side programming language that is widely used in website development. Among them, file uploading and downloading are one of the commonly used functions of websites, and PHP provides a wealth of functions and techniques to implement these functions. In this article, we will introduce in detail the file upload and download techniques in PHP so that you can develop your website more efficiently. File upload File upload refers to sending files from the local computer to a remote server. After uploading the files, we can store, process and display these files. In PHP
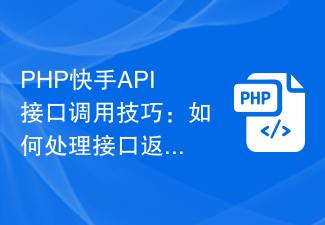
PHP Kuaishou API interface calling tips: How to handle the error information returned by the interface When using PHP to call the Kuaishou API interface, we often encounter situations where the interface returns errors. For error information returned by the processing interface, we need to provide appropriate processing and feedback to improve the stability and user experience of the application. This article will introduce some techniques for handling error information returned by interfaces and provide corresponding code examples. Use try-catch to catch exceptions. When calling the API interface, some exception errors may occur.
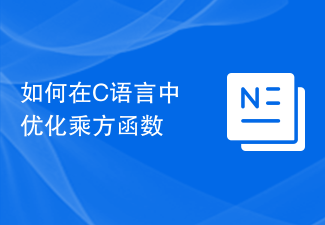
How to write an efficient exponentiation function in C language. The exponentiation operation is a commonly used mathematical operation in computer programs. In C language, we can use loops, recursion, bit operations and other methods to implement exponentiation operations. However, in the case of powers of large numbers, efficiency often becomes an important consideration. This article will introduce an efficient implementation method of the power function and give specific code examples. Before discussing efficient exponentiation functions, let's review the definition of exponentiation. The mathematical definition of exponentiation is to multiply a number (called the base) from
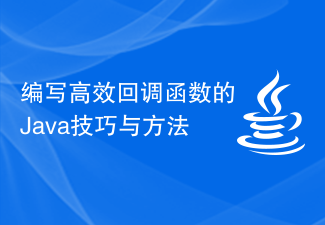
How to write efficient callback functions in Java Callback functions are a common way to implement asynchronous programming. They provide a mechanism so that a function can be called after another function has completed execution. In Java, callback functions are often used in event-driven systems or concurrent programming. This article will introduce how to write efficient callback functions in Java through specific code examples. Defining the callback interface Callback functions are usually defined by the callback interface. A callback method is defined in this interface to be called at the appropriate time. In this way, any
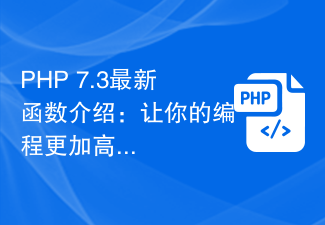
As a widely used programming language, PHP is constantly evolving and adding new features. In early 2019, PHP 7.3 version was grandly launched, including many eye-catching new functions and features. In this article, we will introduce you to some of the latest functions of PHP7.3. We hope that these new functions can make your programming more efficient. is_countable function The new function is_countable can determine whether a variable has a counting function. Returns true if the variable can be counted
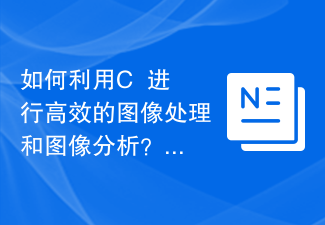
How to use C++ for efficient image processing and image analysis? Image processing and analysis is a very important task in the field of computer vision, which involves the acquisition, processing, analysis and understanding of images. As a high-performance programming language, C++ can provide a rich image processing and analysis library, allowing us to perform image processing and analysis work quickly and efficiently. This article will introduce how to use C++ for efficient image processing and image analysis, and give corresponding code examples. Reading and display of images in image processing and analysis, first
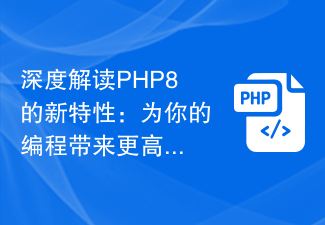
Analysis of new features of PHP8: To make your programming more efficient, specific code examples are required Introduction: PHP8 is the latest version of the PHP programming language, which brings many exciting new features and improvements. These new features can not only improve your programming efficiency, but also make your code more concise, readable and maintainable. This article will introduce some important new features of PHP8, with specific code examples to help you better understand and apply these features. Changes to weakly typed declarations In PHP8, the behavior of weakly typed declarations has changed. before
