Best practice guide for map deletion in Golang
Best practice guide for map deletion in Golang
In the Go language, map is a very important data structure, which provides a key-value pair mapping relationship. When using map, we often need to delete and clear the map. This guide will introduce best practices for map deletion operations in Golang and provide specific code examples.
1. Delete the specified key in the map
In Golang, to delete the specified key in the map, you can use the built-in delete
function. An example is as follows:
package main import "fmt" func main() { m := map[string]int{"a": 1, "b": 2, "c": 3} delete(m, "b") fmt.Println(m) // 输出 map[a:1 c:3] }
2. Delete all keys in the map
If you need to delete all keys in the map, you can do this by traversing the map and deleting the keys one by one. An example is as follows:
package main import "fmt" func main() { m := map[string]int{"a": 1, "b": 2, "c": 3} for k := range m { delete(m, k) } fmt.Println(m) // 输出 map[] }
3. Notes
When deleting a key in the map, you need to pay attention to the following points:
- When deleting a key in the map key, if the key does not exist, the
delete
function will not report an error, nor will it affect other keys in the map. - Deleting elements in the map does not release memory, so when processing large amounts of data, it may cause excessive memory usage. If you need to free up memory, you can consider recreating a new map to replace the original map.
To sum up, the above is the best practice guide for map deletion in Golang and the corresponding code examples. Hope these contents are helpful to you!
The above is the detailed content of Best practice guide for map deletion in Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
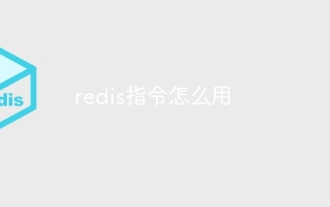
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
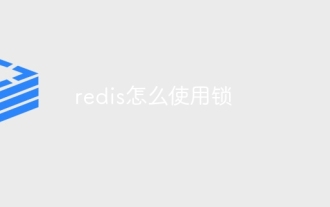
Using Redis to lock operations requires obtaining the lock through the SETNX command, and then using the EXPIRE command to set the expiration time. The specific steps are: (1) Use the SETNX command to try to set a key-value pair; (2) Use the EXPIRE command to set the expiration time for the lock; (3) Use the DEL command to delete the lock when the lock is no longer needed.
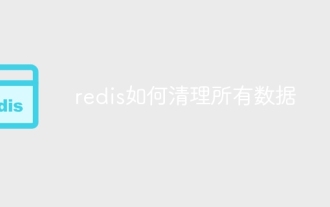
How to clean all Redis data: Redis 2.8 and later: The FLUSHALL command deletes all key-value pairs. Redis 2.6 and earlier: Use the DEL command to delete keys one by one or use the Redis client to delete methods. Alternative: Restart the Redis service (use with caution), or use the Redis client (such as flushall() or flushdb()).
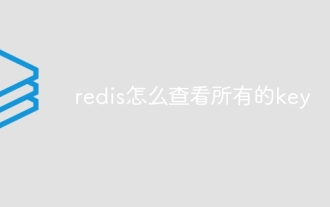
To view all keys in Redis, there are three ways: use the KEYS command to return all keys that match the specified pattern; use the SCAN command to iterate over the keys and return a set of keys; use the INFO command to get the total number of keys.
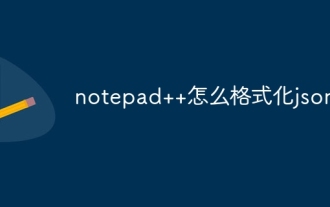
Use the JSON Viewer plug-in in Notepad to easily format JSON files: Open a JSON file. Install and enable the JSON Viewer plug-in. Go to "Plugins" > "JSON Viewer" > "Format JSON". Customize indentation, branching, and sorting settings. Apply formatting to improve readability and understanding, thus simplifying processing and editing of JSON data.

Redis uses hash tables to store data and supports data structures such as strings, lists, hash tables, collections and ordered collections. Redis persists data through snapshots (RDB) and append write-only (AOF) mechanisms. Redis uses master-slave replication to improve data availability. Redis uses a single-threaded event loop to handle connections and commands to ensure data atomicity and consistency. Redis sets the expiration time for the key and uses the lazy delete mechanism to delete the expiration key.
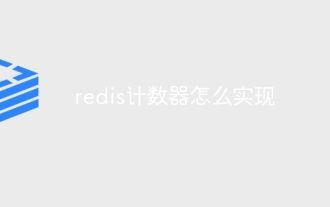
Redis counter is a mechanism that uses Redis key-value pair storage to implement counting operations, including the following steps: creating counter keys, increasing counts, decreasing counts, resetting counts, and obtaining counts. The advantages of Redis counters include fast speed, high concurrency, durability and simplicity and ease of use. It can be used in scenarios such as user access counting, real-time metric tracking, game scores and rankings, and order processing counting.
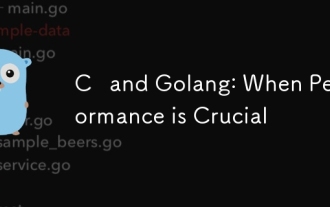
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
