


Java polymorphism: the secret weapon that makes objects flexible and changeable
In object-oriented programming, Java's polymorphism is a powerful feature that allows objects to exhibit flexible and changeable behavior. Through polymorphism, the same method can show different behaviors according to different object types, which brings great convenience to the flexibility and scalability of the code. In this article, PHP editor Xinyi will reveal the secret weapon of Java polymorphism and take you to have an in-depth understanding of this important programming concept so that it can be better applied in actual development.
1. Inheritance to achieve polymorphism
In Java, inheritance is the most common way to achieve polymorphism. When a class is derived from another class, the child class inherits all the properties and methods of the parent class. In addition, subclasses can also define their own properties and methods, thereby extending the functionality of the parent class.
Demo code:
class Animal { public void eat() { System.out.println("Animal is eating"); } } class Dog extends Animal { @Override public void eat() { System.out.println("Dog is eating"); } } public class Main { public static void main(String[] args) { Animal animal = new Dog(); animal.eat(); // 输出:Dog is eating } }
In this example, the Dog
class inherits from the Animal
class and overrides the eat()
method. When we create a Dog
object and assign it to a Animal
variable, we can call the eat()
method, but what is actually executed is ## The eat()
method in the #Dog class.
2. Interface implementation polymorphism
In Java, interfaces are also an important way to achieve polymorphism. An interface is a collection of methods that defines the behavior of an object, but does not define the state of the object. When a class implements an interface, it must implement all methods defined in the interface.
Demo code:
interface Drawable { void draw(); } class Rectangle implements Drawable { @Override public void draw() { System.out.println("Drawing a rectangle"); } } class Circle implements Drawable { @Override public void draw() { System.out.println("Drawing a circle"); } } public class Main { public static void main(String[] args) { Drawable drawable = new Rectangle(); drawable.draw(); // 输出:Drawing a rectangle drawable = new Circle(); drawable.draw(); // 输出:Drawing a circle } }
Drawable interface defines a
draw() method, and both the
Rectangle and
Circle classes implement this interface. When we create a
Drawable object and assign it to a
Rectangle or
Circle variable, we can call the
draw() method, But what is actually executed is the
draw() method in the
Rectangle or
Circle class.
3. Benefits of polymorphism
Polymorphism brings many benefits to Java, including:
- Improve code reusability: Polymorphism allows us to use the same interface in different classes, thereby improving code reusability.
- Improve code maintainability: Polymorphism makes code easier to maintain because we can change the behavior of the object by modifying methods in the subclass without having to modify methods in the parent class.
- Improve the scalability of the code: Polymorphism makes the code easier to extend, because we can extend the functionality of the program by adding new subclasses without having to modify the existing code.
The above is the detailed content of Java polymorphism: the secret weapon that makes objects flexible and changeable. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
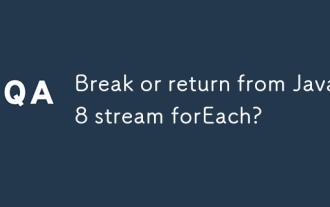
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
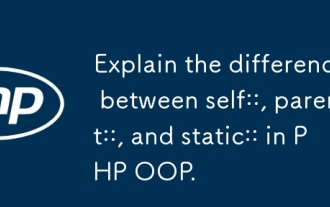
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
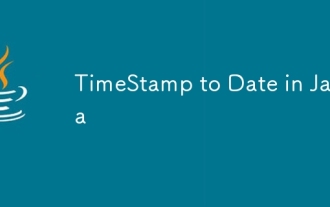
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
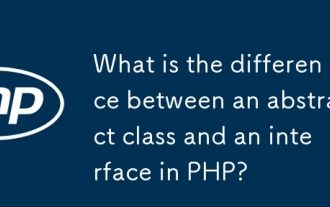
The main difference between an abstract class and an interface is that an abstract class can contain the implementation of a method, while an interface can only define the signature of a method. 1. Abstract class is defined using abstract keyword, which can contain abstract and concrete methods, suitable for providing default implementations and shared code. 2. The interface is defined using the interface keyword, which only contains method signatures, which is suitable for defining behavioral norms and multiple inheritance.
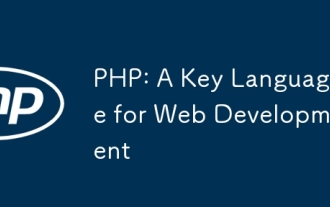
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
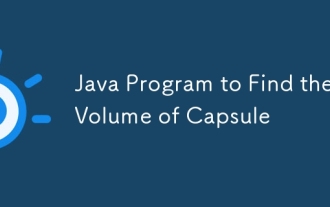
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
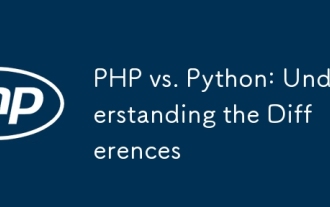
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
