Common ways to handle Java null pointer exceptions
Common solutions to Java null pointer exceptions
In the Java development process, handling null pointer exceptions is an essential task. A null pointer exception is an exception thrown by a program when it operates on an object with a null value. When a null pointer exception occurs in a program, it will cause the program to crash or produce unpredictable results. The following will introduce some common methods to solve null pointer exceptions, as well as specific code examples.
- Use conditional judgment
The most common way to solve the null pointer exception is to use conditional judgment to determine whether the object is null before operating. For example, before using a method of an object, you can first determine whether the object is null. If it is null, the method will not be called. The code example is as follows:
String str = null; if (str != null) { str.length(); }
- Using the Optional class
The Optional class introduced in Java 8 can effectively solve the null pointer exception. The Optional class encapsulates a possibly null value and provides methods to handle the value. For example, you can use the Optional.ofNullable() method to create an Optional object, which may contain a value or be null. If the Optional object is not empty, you can use the get() method to obtain the value. The code example is as follows:
String str = null; Optional<String> optionalStr = Optional.ofNullable(str); if (optionalStr.isPresent()) { String result = optionalStr.get(); result.length(); }
- Use the try-catch statement
When processing a code block where a null pointer exception may occur, you can use the try-catch statement to capture and handle the exception. . By catching exceptions, you can prevent the program from crashing and handle different exceptions accordingly. The code example is as follows:
String str = null; try { str.length(); } catch (NullPointerException e) { // 处理空指针异常 e.printStackTrace(); }
- Using the requireNonNull method of the Objects class
The new Objects class in Java 7 provides some useful methods, one of which is the requireNonNull method . This method can be used to check whether the object is null. If it is null, a NullPointerException is thrown. The code example is as follows:
String str = null; Objects.requireNonNull(str, "str不能为null");
By using the above method, the null pointer exception problem can be effectively solved. However, when writing code, you should try to avoid using null and use default values or empty objects instead. In addition, the occurrence of null pointer exceptions can be reduced through good coding practices and code reviews.
To summarize, handling null pointer exceptions is crucial. During the development process, you should develop good programming habits, try to avoid using null, and carefully handle code blocks that may cause null pointer exceptions. Through reasonable conditional judgment, the use of Optional classes, the use of try-catch statements, and the use of the requireNonNull method of the Objects class, the null pointer exception problem can be effectively solved and the robustness and reliability of the code can be improved.
(Note: The above is only a sample code and does not consider the actual business logic. The specific use needs to be adjusted according to the actual situation)
The above is the detailed content of Common ways to handle Java null pointer exceptions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
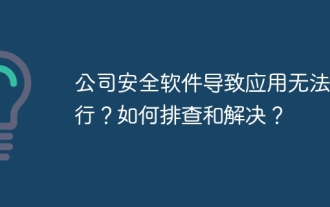
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
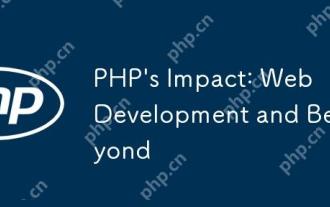
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
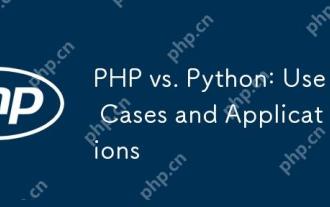
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
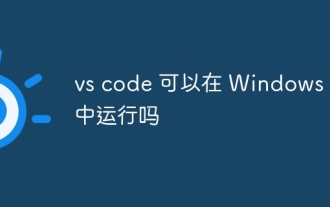
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
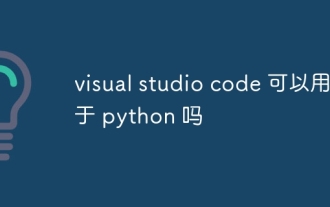
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
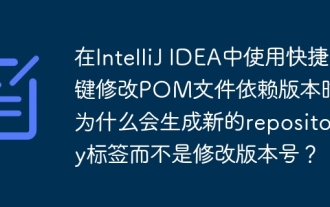
In IntelliJ...
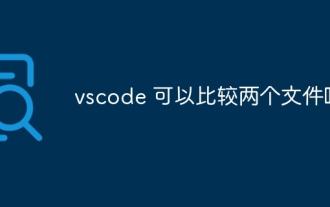
Yes, VS Code supports file comparison, providing multiple methods, including using context menus, shortcut keys, and support for advanced operations such as comparing different branches or remote files.
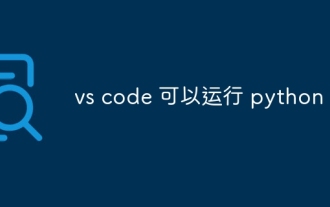
Yes, VS Code can run Python code. To run Python efficiently in VS Code, complete the following steps: Install the Python interpreter and configure environment variables. Install the Python extension in VS Code. Run Python code in VS Code's terminal via the command line. Use VS Code's debugging capabilities and code formatting to improve development efficiency. Adopt good programming habits and use performance analysis tools to optimize code performance.
