


Master the in-depth understanding of the definition and operation skills of Java arrays
In-depth understanding of the definition and operation skills of Java arrays
Arrays in Java are a common data structure that can store multiple elements of the same type and have Fixed size. In this article, we will delve into the definition and manipulation techniques of Java arrays, and provide specific code examples.
-
Definition of array
Arrays can be defined in Java in the following ways:数据类型[] 数组名 = new 数据类型[数组长度];
Copy after loginor:
数据类型[] 数组名 = {元素1, 元素2, ...};
Copy after loginThe data type can be basic Data type, which can also be a reference type.
Array access
The elements of the array can be accessed through the index. The index starts from 0 and increases in sequence. For example, to access the first element of the array, you can use the following code:数组名[0]
Copy after loginExample:
int[] numbers = {1, 2, 3, 4, 5}; System.out.println(numbers[0]); // 输出:1
Copy after loginTraverse the array
You can use a for loop or a foreach loop Traverse the array. The following is an example of using a for loop to traverse an array:int[] numbers = {1, 2, 3, 4, 5}; for (int i = 0; i < numbers.length; i++) { System.out.println(numbers[i]); }
Copy after loginAn example of using a foreach loop to traverse an array:
int[] numbers = {1, 2, 3, 4, 5}; for (int number : numbers) { System.out.println(number); }
Copy after loginThe length of the array
You can use thearray Name.length
Get the length of the array. For example:int[] numbers = {1, 2, 3, 4, 5}; System.out.println(numbers.length); // 输出:5
Copy after loginInitialization of arrays
Arrays in Java are automatically initialized for basic data types, and are initialized to null for reference types. For example:int[] numbers = new int[5]; System.out.println(numbers[0]); // 输出:0 String[] names = new String[3]; System.out.println(names[0]); // 输出:null
Copy after loginCopy of array
You can use theSystem.arraycopy()
method or theArrays.copyOf()
method to copy an array Copy an array into another array. Examples are as follows:int[] source = {1, 2, 3, 4, 5}; int[] target = new int[source.length]; System.arraycopy(source, 0, target, 0, source.length); System.out.println(Arrays.toString(target)); // 输出:[1, 2, 3, 4, 5] int[] source = {1, 2, 3, 4, 5}; int[] target = Arrays.copyOf(source, source.length); System.out.println(Arrays.toString(target)); // 输出:[1, 2, 3, 4, 5]
Copy after loginSorting of arrays
You can use theArrays.sort()
method to sort the array. For example:int[] numbers = {5, 3, 1, 4, 2}; Arrays.sort(numbers); System.out.println(Arrays.toString(numbers)); // 输出:[1, 2, 3, 4, 5]
Copy after loginMultidimensional array
In addition to one-dimensional arrays, Java also supports multi-dimensional arrays. For example, a two-dimensional array can be defined as follows:数据类型[][] 数组名 = new 数据类型[行数][列数];
Copy after loginExample:
int[][] matrix = new int[3][3]; matrix[0][0] = 1; matrix[0][1] = 2; matrix[0][2] = 3; // ...
Copy after login
The above is an in-depth understanding of Java array definition and operation techniques. By learning the definition and operation methods of arrays, we can process data more flexibly and efficiently. I hope the code examples provided in this article can help you better understand and use Java arrays.
The above is the detailed content of Master the in-depth understanding of the definition and operation skills of Java arrays. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
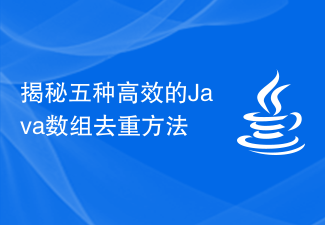
Five efficient Java array deduplication methods revealed In the Java development process, we often encounter situations where we need to deduplicate arrays. Deduplication is to remove duplicate elements in an array and keep only one. This article will introduce five efficient Java array deduplication methods and provide specific code examples. Method 1: Use HashSet to deduplicate HashSet is an unordered, non-duplicate collection that automatically deduplicates when adding elements. Therefore, we can use the characteristics of HashSet to deduplicate arrays. public
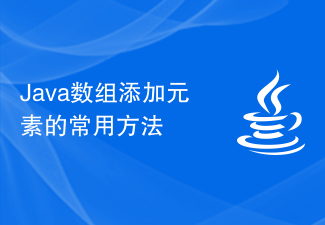
Common ways to add elements to Java arrays, specific code examples are required In Java, an array is a common data structure that can store multiple elements of the same type. In actual development, we often need to add new elements to the array. This article will introduce common methods of adding elements to arrays in Java and provide specific code examples. A simple way to create a new array using a loop is to create a new array, copy the elements of the old array into the new array, and add the new elements. The code example is as follows: //original array i
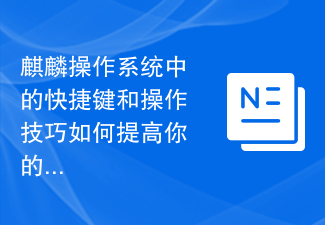
How can the shortcut keys and operation skills in Kirin OS improve your efficiency? Kirin operating system is an open source operating system based on Linux. It is favored by users for its stability, security and powerful functions. When using Kirin operating system on a daily basis, being familiar with and using some shortcut keys and operating techniques can greatly improve work efficiency. This article will introduce you to some common shortcut keys and operating techniques in Kirin operating system, and provide code examples to help you better master these techniques. 1. Open the terminal window. The terminal window is the Kirin operating system.
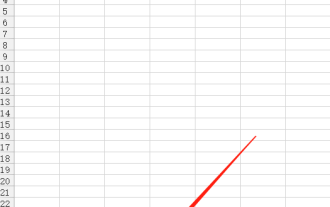
In daily work, we need to use office software, and excel is one of the more commonly used software. However, many friends who have just entered the workplace are not familiar with excel tables. Below, we share the basic skills of excel operation. We hope that You can quickly master these basic operating skills. 1. Open an excel sheet, double-click the sheet name in the lower left corner or right-click and select Rename to name the worksheet. 2. Under normal circumstances, start from the first cell to enter the table information. For example, we need to enter a serial number in the first column, arrange them in order, and enter a serial number first. 3. Let’s enter another “2”. If we need to fill down one by one, select the first and second cells, move the mouse to the lower right corner, and when the
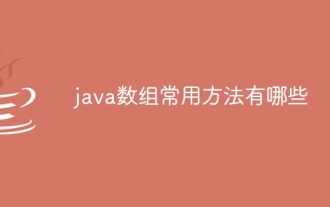
Commonly used methods include length attribute, copy array, array traversal, array sorting, array conversion to string, etc. Detailed introduction: 1. Length attribute: used to get the length of an array. It is an attribute rather than a method. Example: int[] arr = {1, 2, 3}; int length = arr.length;; 2. Copy the array: Use the System.arraycopy() method or the copyOf() method of the Arrays class to copy the contents of the array to a new Arrays etc.
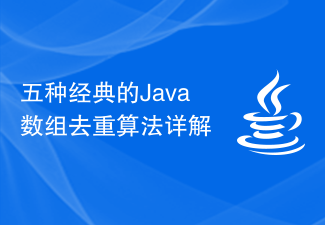
Detailed explanation of five classic Java array deduplication algorithms In Java programming, you often encounter situations where you need to perform deduplication operations on arrays, that is, remove duplicate elements in the array and retain unique elements. The following will introduce five classic Java array deduplication algorithms and provide corresponding code examples. Using HashSet HashSet is a collection class in Java that automatically removes duplicate elements. This feature can be used to quickly achieve array deduplication. Code example: importjava.util.Arr
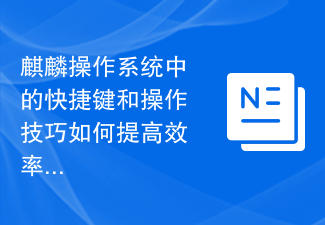
How can the shortcut keys and operating techniques in Kirin OS improve efficiency? Kirin operating system is an operating system for personal computers independently developed in China. As a powerful and stable operating system, Kirin operating system focuses on user experience and operating efficiency in user interface design. In addition to providing rich graphical interface functions, Kirin operating system also supports a wealth of shortcut keys and operating techniques. The optimized design of these functions allows users to manage and operate the system more efficiently. 1. Use of shortcut keys Desktop related shortcut keys: Win key: Display
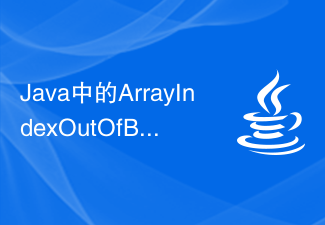
Java is a widely used programming language that provides programmers with many practical and powerful tools and features. When writing Java programs, you may encounter various exceptions. Among them, ArrayIndexOutOfBoundsException is a common exception. This exception is triggered when we try to access an element that does not exist in the array. In this article, we will discuss ArrayIndexOutOfBoundsExc in Java in detail
