Golang data processing skills revealed
Golang data processing skills revealed, need specific code examples
Introduction:
Golang is an efficient and powerful programming language, widely used in data processing field. This article will share some commonly used data processing techniques in Golang and give specific code examples to help readers better understand and apply these techniques.
1. Use slicing for fast data filtering
For a slice that contains a large amount of data, we can use Golang's slicing feature to quickly filter the data. The sample code is as follows:
package main import ( "fmt" ) func filter(data []int, callback func(int) bool) []int { var result []int for _, value := range data { if callback(value) { result = append(result, value) } } return result } func main() { data := []int{1, 2, 3, 4, 5, 6, 7, 8, 9, 10} filteredData := filter(data, func(value int) bool { return value%2 == 0 }) fmt.Println(filteredData) // 输出:[2 4 6 8 10] }
In the above code, we define a filter function, which receives a slice and a callback function to determine whether each element needs to be retained. By traversing the original slice, the return value of the callback function is used to decide whether to add the current element to the resulting slice. In this way, data can be filtered quickly and processing efficiency can be improved.
2. Use Interface to achieve more flexible data processing
Golang’s Interface mechanism allows us to process different types of data more flexibly. The sample code is as follows:
package main import ( "fmt" ) type Shape interface { Area() float64 } type Rectangle struct { Width float64 Height float64 } type Circle struct { Radius float64 } func (r Rectangle) Area() float64 { return r.Width * r.Height } func (c Circle) Area() float64 { return 3.14 * c.Radius * c.Radius } func main() { shapes := []Shape{ Rectangle{Width: 10, Height: 5}, Circle{Radius: 2}, } for _, shape := range shapes { fmt.Println(shape.Area()) } }
In the above code, we define a Shape interface, which contains a method named Area. Then we created a Rectangle and a Circle structure and implemented the Area method for them. By storing these structures in a Shape type slice, we can call the Area methods of different structures by traversing the slice and achieve a more flexible data processing method.
3. Use concurrent processing to accelerate large data set operations
When faced with large-scale data sets, using concurrent processing can significantly improve the operating efficiency of the program. The following is a simple sample code:
package main import ( "fmt" "sync" ) func processData(data []int) []int { var result []int var wg sync.WaitGroup var mu sync.Mutex for _, value := range data { wg.Add(1) go func(val int) { defer wg.Done() // 假设这里是需要耗时的数据处理操作 processedValue := val * 2 mu.Lock() result = append(result, processedValue) mu.Unlock() }(value) } wg.Wait() return result } func main() { data := []int{1, 2, 3, 4, 5, 6, 7, 8, 9, 10} result := processData(data) fmt.Println(result) // 输出:[2 4 6 8 10 12 14 16 18 20] }
In the above code, we define a processData function to process the data set. By processing data concurrently, we can use multiple goroutines to perform time-consuming data processing operations in parallel, improving the overall processing speed. During concurrent operations, we need to pay attention to mutually exclusive access to shared variables. Here, sync.WaitGroup and sync.Mutex are used for thread synchronization and mutually exclusive access.
Conclusion:
This article introduces some commonly used data processing techniques in Golang and gives specific code examples. Fast filtering of slices, using interfaces to achieve more flexible data processing, and using concurrent processing to accelerate large data set operations, these techniques can help us process data more efficiently. By learning and applying these techniques, I believe readers can discover more application scenarios in actual projects and improve the efficiency and quality of data processing.
The above is the detailed content of Golang data processing skills revealed. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
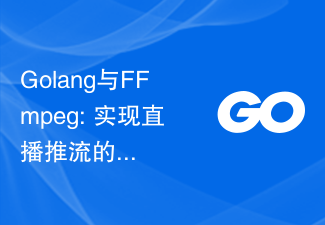
Golang and FFmpeg: Technical implementation of live streaming requires specific code examples. Introduction: In recent years, the rapid development and popularization of live broadcast technology has made live broadcast an increasingly popular media method. Among them, real-time streaming technology is the key to realizing live broadcast. This article will introduce how to use the programming language Golang and the multimedia processing tool FFmpeg to realize the technical implementation of live streaming, and provide some related code examples. 1. Introduction to Golang and FFmpeg technology 1.1
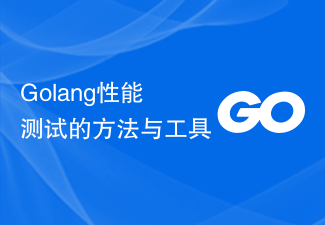
Introduction to Golang performance testing methods and tools: In software development, performance testing is an important link. Performance testing allows developers to verify a program's performance and identify potential performance bottlenecks. As a high-performance programming language, Golang also provides some methods and tools to help developers conduct performance testing. This article will introduce several commonly used performance testing methods and tools in Golang, with code examples. 1. Benchmark testing Golang provides a built-in benchmark testing framework that can be easily
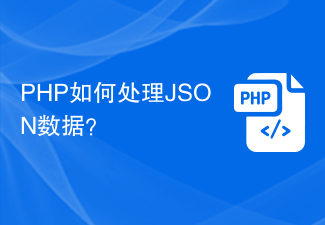
PHP is a widely used open source server-side scripting language with the ability to handle various data formats, including JSON (JavaScript Object Notation) data. JSON is a lightweight data exchange format that is very commonly used for front-end and back-end data transmission and storage. In PHP, you can use built-in functions and classes to process JSON data. Some commonly used methods and techniques will be introduced below. Parsing JSON data PHP provides multiple ways to parse JSON data
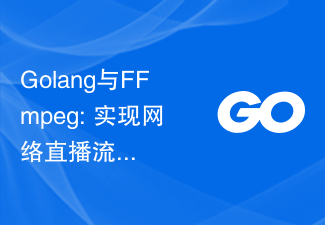
Golang and FFmpeg: To implement the push technology of online live streaming media, specific code examples are needed. Abstract: With the popularity of online live broadcast, more and more developers have begun to pay attention to how to implement the push technology of streaming media. This article will introduce how to use Golang and FFmpeg to realize the push of online live streaming media. Through specific code examples, readers will be guided step by step to understand how to build a push system based on Golang and FFmpeg. 1. Introduction to Golang language Golang
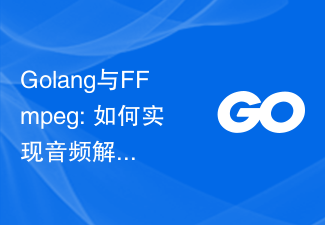
Golang and FFmpeg: How to implement audio decoding and encoding, specific code examples are needed. Introduction: With the continuous development of multimedia technology, audio processing has become an indispensable part of many applications. This article will introduce how to use Golang and FFmpeg libraries to implement audio decoding and encoding functions, and provide specific code examples. 1. What is FFmpeg? FFmpeg is a powerful open source multimedia processing tool that can decode, encode, convert, and stream audio and video.
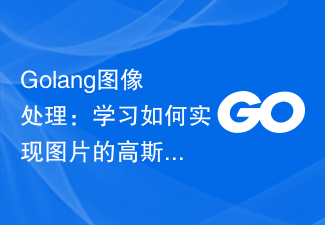
Golang Image Processing: Learn how to achieve the Gaussian blur effect of images Introduction: Image processing plays an important role in the field of computer vision. In image processing, Gaussian blur is a commonly used technique to blur images to reduce noise and detail in the image. In this article, we will learn how to use Golang to achieve the Gaussian blur effect of images, with code examples attached. Environment preparation: First, make sure that the Golang development environment has been installed correctly. By in Terminal or Command Prompt

How to use Golang to progressively load and compress images 1. Introduction Nowadays, the use of images on the Internet has become extremely common. However, problems such as slow loading of large images and inability to display progressively also occur frequently, affecting the user experience. This article will introduce how to use Golang to progressively load and compress images to improve user loading speed and experience. 2. Progressive loading. The so-called progressive loading means that before the image is completely loaded, a small part of its quality can be gradually displayed. exist
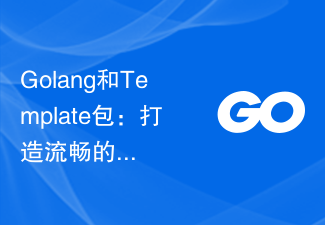
Golang and Template package: Create a smooth web experience In today's Internet era, the user experience of web pages is crucial to the success of the website. As web technology continues to develop and innovate, developers need to use efficient and concise tools to build smooth user interfaces. In the field of Golang development, the Template package is a powerful tool that helps developers implement fast and maintainable web page templates. The Template package is the template engine that comes with the Golang standard library. It provides a
