How to use WebSocket in golang for real-time data update
How to use WebSocket in Golang for real-time data updates
Overview:
WebSocket is a method for full duplex between a web browser and a server Communication technology designed for communication. In Golang, we can use net/http
and github.com/gorilla/websocket
in the standard library to implement WebSocket functionality. This article will introduce how to use WebSocket in Golang for real-time data updates and provide some code examples.
Steps:
Step One: Create HTTP Server
First, we need to create an HTTP server to handle WebSocket connection requests. Here is a simple example code:
package main import ( "log" "net/http" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { http.ServeFile(w, r, "index.html") }) log.Println("HTTP server is starting at http://localhost:8080") log.Fatal(http.ListenAndServe(":8080", nil)) }
This code creates a simple HTTP server that maps the root path ("/") to a file called index.html
static files.
Step 2: Handle WebSocket connections
Next, we need to modify the code of the HTTP server so that it can handle WebSocket connection requests. We can use the github.com/gorilla/websocket
library to handle WebSocket connections. The following is the modified sample code:
package main import ( "log" "net/http" "github.com/gorilla/websocket" ) var ( upgrader = websocket.Upgrader{ ReadBufferSize: 1024, WriteBufferSize: 1024, } clients = make(map[*websocket.Conn]bool) ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { http.ServeFile(w, r, "index.html") }) http.HandleFunc("/ws", func(w http.ResponseWriter, r *http.Request) { conn, err := upgrader.Upgrade(w, r, nil) if err != nil { log.Println("Failed to upgrade connection:", err) return } clients[conn] = true for { _, msg, err := conn.ReadMessage() if err != nil { log.Println("Failed to read message from client:", err) delete(clients, conn) break } for client := range clients { err := client.WriteMessage(websocket.TextMessage, msg) if err != nil { log.Println("Failed to write message to client:", err) client.Close() delete(clients, conn) } } } }) log.Println("WebSocket server is starting at ws://localhost:8080/ws") log.Fatal(http.ListenAndServe(":8080", nil)) }
In this code, we create an upgrader
object and define a clients
variable to save all connections client. When there is a new WebSocket request, we upgrade the connection to a WebSocket connection and add it to the clients
variable. Then, we read the message from the client through a loop and send the message to all connected clients.
Step 3: Create a front-end page
Finally, we need to create a front-end page to connect to the WebSocket server and display real-time data updates. The following is a simple HTML page example (index.html
):
<!DOCTYPE html> <html> <head> <title>WebSocket Demo</title> </head> <body> <h1 id="WebSocket-Demo">WebSocket Demo</h1> <input type="text" id="message-input"> <button onclick="send()">Send</button> <ul id="message-list"></ul> <script> var socket = new WebSocket("ws://localhost:8080/ws"); socket.onmessage = function(event) { var message = document.createElement("li"); message.textContent = event.data; document.getElementById("message-list").appendChild(message); }; function send() { var input = document.getElementById("message-input"); var message = input.value; input.value = ""; socket.send(message); } </script> </body> </html>
This code creates a WebSocket connection and listens to the onmessage
event when a message arrives When, add the message to a ul
element for display. In addition, an input box and a send button are provided so that users can enter messages and send them to the server via WebSocket.
Summary:
Through the above steps, we can use WebSocket in Golang for real-time data updates. By creating HTTP servers, handling WebSocket connections, and interacting with front-end pages, we can achieve real-time data communication in web applications. Of course, this is just a simple example and may need to be expanded and modified according to specific needs during actual use. I hope this article was helpful and I wish you success when using WebSocket!
The above is the detailed content of How to use WebSocket in golang for real-time data update. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










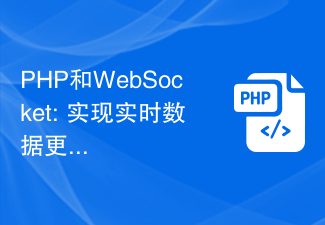
PHP and WebSocket: The best way to achieve real-time data updates Introduction: With the development of network technology, real-time data updates are becoming more and more important in many websites and applications. Although the traditional HTTP request-response model can update data, it has low utilization of server resources and bandwidth. In contrast, real-time data updates based on WebSocket can provide a low-latency and efficient way to push data to the client, and is suitable for scenarios such as real-time chat, real-time statistics, and real-time monitoring. This article will introduce
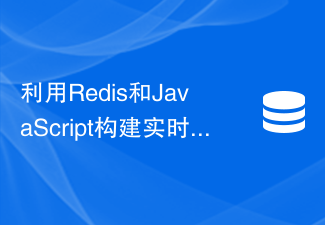
Using Redis and JavaScript to build a real-time stock quotation system: how to quickly update data. With the continuous development of the capital market, investors' demand for real-time stock quotations is also increasing. Building a real-time stock quotation system that quickly updates data is undoubtedly the pursuit of investors. This article will introduce how to use Redis and JavaScript to build such a system, and provide corresponding code examples. 1. Introduction to Redis Redis is a high-performance key-value pair storage database that uses memory
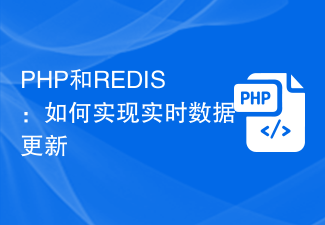
PHP and REDIS: How to implement real-time data updates Overview: Real-time data updates are one of the common requirements in modern web applications. By using a combination of PHP and REDIS, we can achieve real-time data updates in a simple and efficient way. This article will introduce how to use PHP and REDIS to implement real-time data updates in web applications and provide relevant code examples. What is REDIS: REDIS is an open source memory data storage system, which is often used to solve data reading and writing in high concurrency scenarios.

Real-time data updates using the ReactQuery database plugin ReactQuery is a powerful data management tool that can help us simplify the process of processing data in React applications. It provides elegant solutions for operations such as data retrieval, caching and updating. This article will introduce how to use the ReactQuery plug-in to implement real-time data update function, and provide specific code examples. To better understand this process, we'll take a simple task management application as an example. we fake
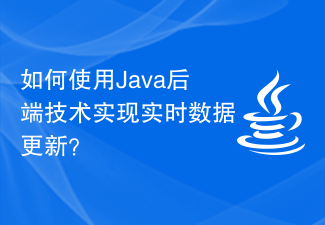
How to use Java backend technology to achieve real-time data updates? With the rapid development of the Internet, real-time data updates have become increasingly important for many businesses and applications. As a powerful programming language, Java has rich back-end technologies and tools that can help developers update real-time data. This article will introduce how to use Java backend technology to achieve real-time data updates and provide code examples. 1. Use WebSocket for real-time data transmission. WebSocket is a TCP-based protocol.
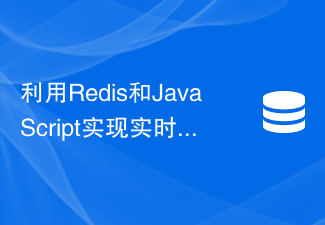
Real-time data update function using Redis and JavaScript In modern web applications, real-time data update function is widely used. For example, social media applications need to display new messages instantly; online games need to update information such as the user's score and location in real time. In order to achieve such a function, we can use Redis and JavaScript to achieve real-time data updates. Redis is a high-performance in-memory key-value storage database suitable for scenarios such as caching, messaging, and real-time analysis.
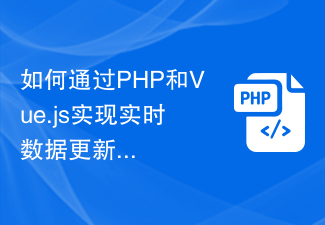
How to implement statistical charts with real-time data updates through PHP and Vue.js Introduction: In modern web applications, data visualization is a very important part. Through statistical charts, we can understand the trends and patterns of data more clearly. Moreover, if these statistical charts can be updated in real time, real-time data analysis and decision support can be provided. This article will introduce how to use PHP and Vue.js to implement statistical charts with real-time data updates. Technical preparation: PHP5.4 or higher version Vue.js2.
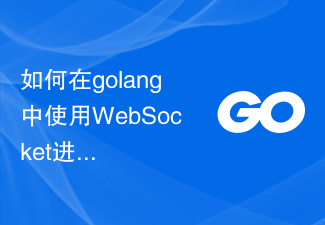
Overview of how to use WebSocket for real-time data updates in Golang: WebSocket is a communication technology designed for full-duplex communication between a web browser and a server. In Golang, we can use net/http and github.com/gorilla/websocket in the standard library to implement WebSocket functionality. This article will introduce how to use WebSocket in Golang for real-time data update
