


How to use PHP cache development to reduce network bandwidth consumption
How to use PHP to develop cache to reduce network bandwidth consumption
Network bandwidth consumption is a headache, especially when the website has a large number of visits and a large amount of data when. To reduce network bandwidth consumption, an effective method is to use caching. In this article, we will introduce how to use PHP to develop cache to reduce network bandwidth consumption, and attach specific code examples.
- Understand the principles of caching
Before you start using caching, you must first understand the principles of caching. Simply put, caching is to store some frequently accessed data in the memory or file system so that it can be quickly obtained when needed next time without having to obtain it again from the database or other data sources. By using cache, you can reduce the number of accesses to the database or other data sources, thereby reducing network bandwidth consumption.
- Using PHP cache extensions
PHP provides some cache extensions, such as APC, Redis, Memcached, etc. These extensions can help us implement caching functions conveniently. Here is a sample code using the APC extension:
<?php $key = 'cache_key'; $data = apc_fetch($key); if ($data === false) { // 从数据库或者其他数据源获取数据 $data = fetchDataFromDatabase(); // 将数据存入缓存 apc_store($key, $data, 3600); // 缓存1小时 } // 使用获取到的数据进行其他操作 processData($data); ?>
In this example, we first try to get the data from the cache, and if the retrieval fails, get the data from the database and store the data in the cache. The next time the data is needed, it can be retrieved directly from the cache without accessing the database again.
- Set a reasonable cache time
When using cache, you need to set a reasonable cache time according to specific business needs. If the data does not change frequently, you can set a longer cache time, which can reduce the number of accesses to the database or other data sources. However, if the data changes frequently and needs to be updated in a timely manner, a shorter cache time should be set to ensure that the data obtained is the latest.
- Cache update strategy
When updating data, the cache needs to be updated in time to prevent old data from being obtained. A common approach is to delete the corresponding cache after the data is updated, so that the next time data is needed, the latest data will be obtained from the database or other data sources. For example:
<?php // 更新数据 updateData(); // 删除缓存 $key = 'cache_key'; apc_delete($key); ?>
- Cache cleaning strategy
Since the cache is stored in the memory or file system, if it is not cleaned regularly, it may cause memory or disk space Too occupied. Therefore, a reasonable cache cleaning strategy needs to be developed. A common approach is to set the cache expiration time and automatically clear the cache when it expires. For example:
<?php $key = 'cache_key'; $data = apc_fetch($key); if ($data === false) { // 从数据库或者其他数据源获取数据 $data = fetchDataFromDatabase(); // 将数据存入缓存,并设置过期时间 apc_store($key, $data, 3600); // 缓存1小时 } ?>
In this example, the cache expiration time is set to 1 hour. When the cache expires, the next time the data is needed, the cache will be automatically cleared and the data will be obtained from the database again.
Through the above points, we can use PHP to develop cache to effectively reduce the consumption of network bandwidth. Of course, in actual development, more factors may need to be considered, such as cache storage location, cache distributed processing, etc. But no matter what, understanding the principles of caching, choosing appropriate cache extensions, and setting reasonable cache time and cleanup strategies are all effective ways to reduce network bandwidth consumption.
The above is the detailed content of How to use PHP cache development to reduce network bandwidth consumption. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
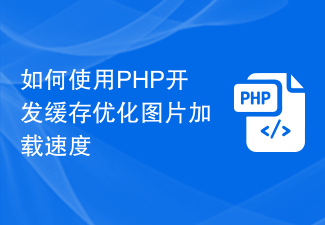
How to use PHP to develop cache and optimize image loading speed. With the rapid development of the Internet, web page loading speed has become one of the important factors in user experience. Image loading speed is one of the important factors affecting web page loading speed. In order to speed up the loading of images, we can use PHP development cache to optimize the image loading speed. This article will introduce how to use PHP to develop cache to optimize image loading speed, and provide specific code examples. 1. Principle of cache Cache is a technology for storing data by temporarily storing data in high-speed memory.

Output caching in the PHP language is one of the commonly used performance optimization methods, which can greatly improve the performance of web applications. This article will introduce output caching in PHP and how to use it to optimize the performance of web applications. 1. What is output caching? In web applications, when we use PHP to output a piece of HTML code, PHP will output this code to the client line by line. Each line output will be immediately sent to the client. This method will cause a large number of network I/O operations, and network I/O is a bottleneck for web application performance.
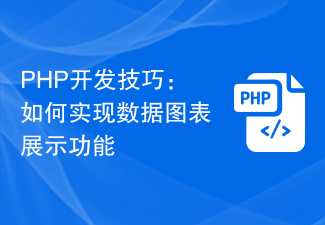
PHP development skills: How to implement data chart display function Introduction: In modern web application development, data visualization is a very important function. By using charts to display data, the data can be presented to users more intuitively and clearly, helping users better understand and analyze the data. This article will introduce how to use PHP to implement data chart display functions and provide specific code examples. 1. Preparation Before starting to write code, we need to install a PHP chart library. Here we recommend using GoogleC
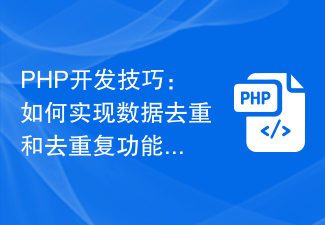
PHP development skills: How to implement data deduplication and deduplication functions. In actual development, we often encounter situations where we need to deduplicate or deduplicate data collections. Whether it is data in the database or data from external data sources, there may be duplicate records. This article will introduce some PHP development techniques to help developers implement data deduplication and deduplication functions. 1. Array-based data deduplication. If the data exists in the form of an array, we can use the array_unique() function to achieve it.
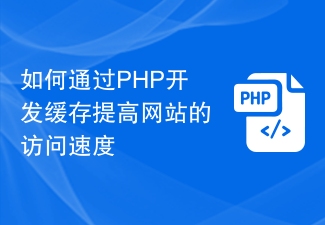
With the development of the Internet, website access speed has become one of the important factors for users to choose a website. For large websites with huge traffic, each page request may take a lot of time and resources. In order to solve this problem, we can greatly improve the access speed of the website by using caching technology. This article will introduce how to improve the access speed of the website through PHP cache development, including specific code examples. 1. Caching Concept and Principle Caching is a method of temporarily storing frequently used data in high-speed memory so that it can be retrieved faster.
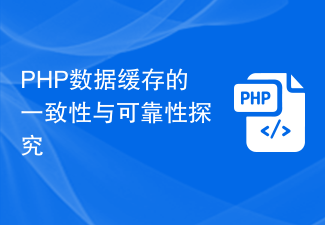
Research on the consistency and reliability of PHP data caching Introduction: In web development, data caching is one of the important means to improve application performance. As a commonly used server-side scripting language, PHP also provides a variety of data caching solutions. However, when using these caching solutions, we need to consider cache consistency and reliability issues. This article will explore the consistency and reliability of PHP data caching and provide corresponding code examples. 1. The issue of cache consistency When using data caching, the most important issue is how to ensure caching
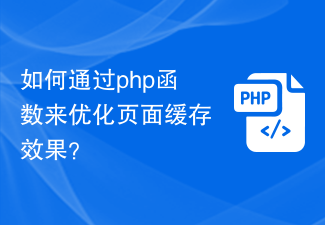
How to optimize page caching effect through PHP function? Overview: In website development, optimizing page caching is one of the important links in improving user experience and website performance. By properly setting the page cache, you can reduce the load on the server, speed up page loading, and improve user access experience. And PHP function is one of the tools we can use. This article will introduce some basic PHP functions and how to use them to optimize page caching. 1. Understand the PHP function: ob_start() function:
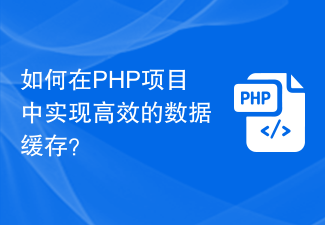
How to implement efficient data caching in PHP projects? Introduction: In developing PHP projects, data caching is a very important technology that can significantly improve the performance and response speed of the application. This article will introduce how to implement efficient data caching in PHP projects, including selecting appropriate caching technology, life cycle management of cached data, and usage examples. 1. Choose the appropriate caching technology. File caching: Use the file system to store cached data. The cached data can be saved on the disk. It is durable and suitable for processing large amounts of data, but reading
