How to use middleware for role management in Laravel
How to use middleware for role management in Laravel
Role management is a very important feature when developing web applications. Through role management, the access rights of different users can be restricted to ensure system security and data confidentiality. In the Laravel framework, role management can be achieved through middleware.
Middleware is a feature of the Laravel framework that can perform some logic before or after the request reaches the route. By using middleware, you can easily restrict users' access based on their roles.
Let’s take a look at the specific steps on how to use middleware for role management.
- Create a middleware
First, we need to create a middleware. Run the following command on the command line to create a middleware named RoleMiddleware:
php artisan make:middleware RoleMiddleware
This command will create a RoleMiddleware.php file in the app/Http/Middleware directory.
In the RoleMiddleware.php file, we need to implement a handle method, which will be executed when the middleware is executed. In this method, we can write our logic to determine whether the user's role has the corresponding permissions.
- Writing middleware logic
In the handle method of the RoleMiddleware.php file, we can write our role management logic. For example, we can use Laravel's Auth facade to get the role of the currently logged in user and compare it with the role we set. If the role matches, we can continue to execute the request, otherwise return an error page or redirect to other pages. The following is a simple sample code:
public function handle($request, Closure $next, ...$roles) { $user = Auth::user(); if (!in_array($user->role, $roles)) { return redirect('/403'); //没有权限 } return $next($request); }
In this example, we get the role of the currently logged in user through the Auth facade and then compare it with the role passed into the middleware. If the user's role is not in the specified role array $roles, we redirect the user to a 403 page and return a page without permissions.
- Register middleware
In the Laravel framework, we need to register the middleware into the middleware group or route before it can be used. In the app/Http/Kernel.php file, we can find the $middlewareGroups attribute or the $routeMiddleware attribute. We can add the middleware we created to these properties in the appropriate places. For example, we can add middleware to the web middleware group so that it applies to all web routes:
protected $middlewareGroups = [ 'web' => [ ... AppHttpMiddlewareRoleMiddleware::class, ], ];
We can also apply middleware directly to a route. For example, we can create a routing group and specify the middleware in the routing group as follows:
Route::middleware('role:admin')->group(function () { //这里的路由只允许角色为admin的用户访问 });
In this example, we apply the RoleMiddleware middleware to this routing group, only those with the role of admin Only users can access these routes.
So far, we have completed the steps of using middleware for role management in Laravel. Through this simple example, you can perform more complex role management according to your actual needs.
Summary
Role management is an important function that can be achieved by using middleware. In the Laravel framework, we can manage roles by creating middleware, writing middleware logic, and registering middleware. Through reasonable use of middleware, we can easily restrict the access rights of different users and improve system security and data confidentiality.
I hope this article can help you use middleware for role management in Laravel. If you have any questions or suggestions, please leave a comment below.
The above is the detailed content of How to use middleware for role management in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
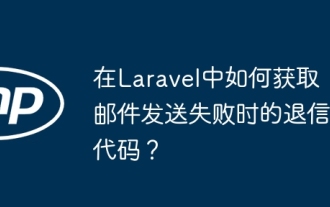
Method for obtaining the return code when Laravel email sending fails. When using Laravel to develop applications, you often encounter situations where you need to send verification codes. And in reality...
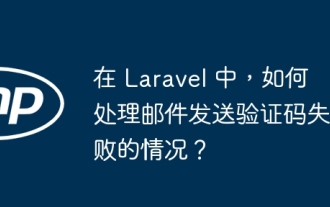
The method of handling Laravel's email failure to send verification code is to use Laravel...
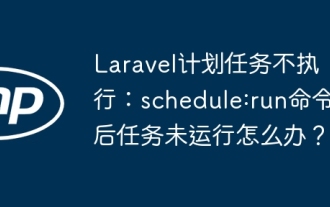
Laravel schedule task run unresponsive troubleshooting When using Laravel's schedule task scheduling, many developers will encounter this problem: schedule:run...
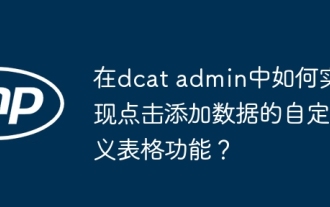
How to implement the table function of custom click to add data in dcatadmin (laravel-admin) When using dcat...
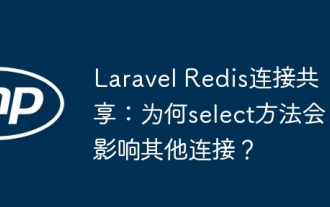
The impact of sharing of Redis connections in Laravel framework and select methods When using Laravel framework and Redis, developers may encounter a problem: through configuration...
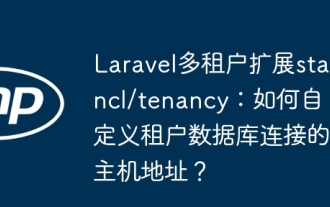
Custom tenant database connection in Laravel multi-tenant extension package stancl/tenancy When building multi-tenant applications using Laravel multi-tenant extension package stancl/tenancy,...
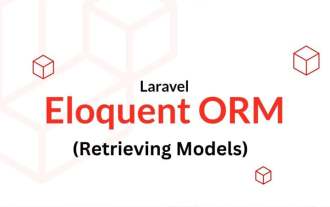
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
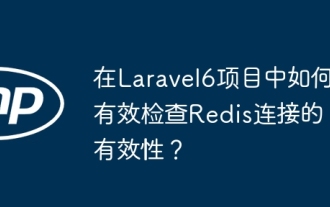
How to check the validity of Redis connections in Laravel6 projects is a common problem, especially when projects rely on Redis for business processing. The following is...
