How to use Laravel to implement user rights management functions
How to use Laravel to implement user rights management functions
With the development of web applications, user rights management has become more and more important in many projects. Laravel, as a popular PHP framework, provides many powerful tools and functions for handling user rights management. This article will introduce how to use Laravel to implement user rights management functions and provide specific code examples.
- Database design
First, we need to design a database model to store the relationship between users, roles and permissions. To simplify the operation, we will use Laravel's own migration tool to create database tables. Open the command line tool and switch to the project root directory, enter the following command to create the migration file:
php artisan make:migration create_roles_table --create=roles php artisan make:migration create_permissions_table --create=permissions php artisan make:migration create_role_user_table --create=role_user php artisan make:migration create_permission_role_table --create=permission_role
Then find the generated migration files in the database/migrations
directory, and edit them. The following is sample code:
// roles表迁移文件 public function up() { Schema::create('roles', function (Blueprint $table) { $table->increments('id'); $table->string('name')->unique(); $table->timestamps(); }); } // permissions表迁移文件 public function up() { Schema::create('permissions', function (Blueprint $table) { $table->increments('id'); $table->string('name')->unique(); $table->timestamps(); }); } // role_user关联表迁移文件 public function up() { Schema::create('role_user', function (Blueprint $table) { $table->integer('role_id')->unsigned(); $table->integer('user_id')->unsigned(); $table->foreign('role_id')->references('id')->on('roles'); $table->foreign('user_id')->references('id')->on('users'); }); } // permission_role关联表迁移文件 public function up() { Schema::create('permission_role', function (Blueprint $table) { $table->integer('permission_id')->unsigned(); $table->integer('role_id')->unsigned(); $table->foreign('permission_id')->references('id')->on('permissions'); $table->foreign('role_id')->references('id')->on('roles'); }); }
After completing editing the migration file, run the following command to perform the migration:
php artisan migrate
- Create model and relationship
Connect Next, we need to create Laravel models to map database tables and establish relationships between them. Open the command line tool and enter the following command to generate the model file:
php artisan make:model Role php artisan make:model Permission
Then open the generated model file and add the following code:
// Role模型 class Role extends Model { public function users() { return $this->belongsToMany(User::class); } public function permissions() { return $this->belongsToMany(Permission::class); } } // Permission模型 class Permission extends Model { public function roles() { return $this->belongsToMany(Role::class); } }
- Add user association
Open the User
model file and add the following method to the class:
public function roles() { return $this->belongsToMany(Role::class); } public function hasRole($role) { if (is_string($role)) { return $this->roles->contains('name', $role); } return !! $role->intersect($this->roles)->count(); } public function assignRole($role) { return $this->roles()->save( Role::whereName($role)->firstOrFail() ); }
In the code, the Role
model is created using the belongsToMany
method The many-to-many relationship with the User
model, the hasRole
method is used to determine whether the user has a certain role, and the assignRole
method is used to assign a role to the user .
- Add Permission Association
In the Role
model, we have defined multiple pairs with the Permission
model There are many relationships, so existing methods can be used directly.
- Middleware configuration
Laravel provides middleware functions to control routing permissions. We need to configure middleware to restrict user access. Open the app/Http/Kernel.php
file and add the following code in the $routeMiddleware
array:
'role' => AppHttpMiddlewareRoleMiddleware::class, 'permission' => AppHttpMiddlewarePermissionMiddleware::class,
- Create middleware
In the command line tool, enter the following command to generate the middleware file:
php artisan make:middleware RoleMiddleware php artisan make:middleware PermissionMiddleware
Then open the generated middleware file and add the following code:
// RoleMiddleware class RoleMiddleware { public function handle($request, Closure $next, $role) { if (! $request->user()->hasRole($role)) { abort(403, 'Unauthorized'); } return $next($request); } } // PermissionMiddleware class PermissionMiddleware { public function handle($request, Closure $next, $permission) { if (! $request->user()->hasPermissionTo($permission)) { abort(403, 'Unauthorized'); } return $next($request); } }
In the code, RoleMiddleware
Checks whether the user has the specified role, PermissionMiddleware
Checks whether the user has the specified permissions.
- Using middleware
Now, you can use the middleware we defined to restrict access on routes that require permission control. In the routing file, use the middleware
method and pass in the middleware name, as in the following example:
Route::get('/admin', function () { // 限制只有拥有admin角色的用户才能访问 })->middleware('role:admin'); Route::get('/delete-user', function () { // 限制只有拥有delete-user权限的用户才能访问 })->middleware('permission:delete-user');
So far, we have implemented the function of using Laravel for user rights management, and passed the middleware Access rights are restricted by the software. Through the use of database models, relationships, middleware and other functions, flexible management and control of users, roles and permissions are achieved.
Summary:
User rights management is an integral part of web applications, and Laravel provides us with powerful tools and functions to achieve this need. This article demonstrates how to use Laravel to implement user rights management functions through detailed steps such as database design, model association, and middleware configuration, and provides specific code examples. I hope this article will be helpful to you when managing user rights.
The above is the detailed content of How to use Laravel to implement user rights management functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
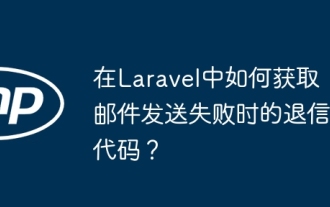
Method for obtaining the return code when Laravel email sending fails. When using Laravel to develop applications, you often encounter situations where you need to send verification codes. And in reality...
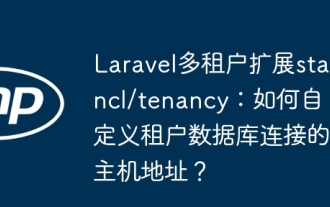
Custom tenant database connection in Laravel multi-tenant extension package stancl/tenancy When building multi-tenant applications using Laravel multi-tenant extension package stancl/tenancy,...
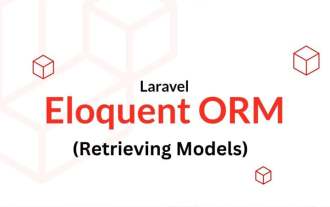
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
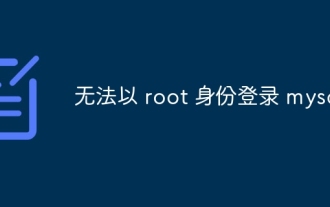
The main reasons why you cannot log in to MySQL as root are permission problems, configuration file errors, password inconsistent, socket file problems, or firewall interception. The solution includes: check whether the bind-address parameter in the configuration file is configured correctly. Check whether the root user permissions have been modified or deleted and reset. Verify that the password is accurate, including case and special characters. Check socket file permission settings and paths. Check that the firewall blocks connections to the MySQL server.
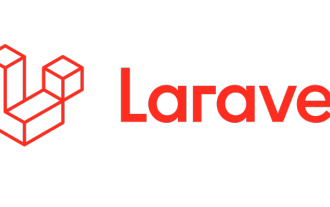
Efficiently process 7 million records and create interactive maps with geospatial technology. This article explores how to efficiently process over 7 million records using Laravel and MySQL and convert them into interactive map visualizations. Initial challenge project requirements: Extract valuable insights using 7 million records in MySQL database. Many people first consider programming languages, but ignore the database itself: Can it meet the needs? Is data migration or structural adjustment required? Can MySQL withstand such a large data load? Preliminary analysis: Key filters and properties need to be identified. After analysis, it was found that only a few attributes were related to the solution. We verified the feasibility of the filter and set some restrictions to optimize the search. Map search based on city
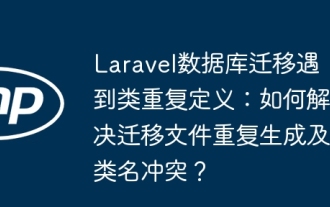
A problem of duplicate class definition during Laravel database migration occurs. When using the Laravel framework for database migration, developers may encounter "classes have been used...
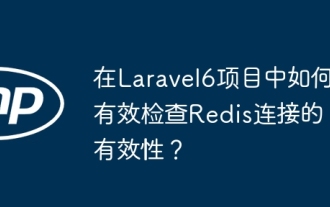
How to check the validity of Redis connections in Laravel6 projects is a common problem, especially when projects rely on Redis for business processing. The following is...

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.
