


How to use PHP7's anonymous functions and closures to achieve more flexible and scalable business logic processing?
How to use PHP7’s anonymous functions and closures to achieve more flexible and scalable business logic processing?
In PHP development, anonymous functions and closures are very powerful features. With the help of anonymous functions and closures, we can handle business logic more flexibly and improve the scalability and maintainability of the code. The following will introduce how to use PHP7's anonymous functions and closures to achieve this goal, and provide specific code examples.
1. The basic concept of anonymous functions
Anonymous functions, also called closure functions, are functions without a specified name. We can assign anonymous functions directly to a variable or pass them as parameters to other functions. Using anonymous functions, we can more conveniently handle some logic that is only used once.
2. Usage scenarios of anonymous functions
1. Callback function: Pass the anonymous function as a parameter to other functions to implement the callback function.
2. Filter the array: Use an anonymous function to filter the array and only retain elements that meet the conditions.
3. Delayed execution: Encapsulate the logic in an anonymous function and call it manually as needed.
3. The basic concept of closure
A closure is a special form of anonymous function that can "remember" the variables in the context in which it was created. In other words, a closure can still access previously existing variables after the function has completed execution.
4. Usage scenarios of closures
1. Encapsulating privatized variables: Using closures, variables can be defined and used in areas that cannot be directly accessed outside the function.
2. Protect variables: Variables in closures can be protected and cannot be modified externally.
The following are specific code examples:
1. Callback function example:
function performAction($callback) { $result = '进行某些操作'; $callback($result); } performAction(function($result) { echo '回调函数被调用,结果为:' . $result; });
2. Filter array example:
$numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; $oddNumbers = array_filter($numbers, function($number) { return $number%2 != 0; }); print_r($oddNumbers);
3. Delayed execution example :
function logMessage($message) { return function() use ($message) { echo '日志消息:' . $message; }; } // 延迟执行日志 $log = logMessage('这是一条延迟执行的日志消息'); $log();
The above examples show how to use anonymous functions and closures to handle business logic in different scenarios. Through anonymous functions and closures, we can encapsulate and execute business logic more flexibly, improving the scalability and readability of the code.
Summary:
PHP7’s anonymous functions and closures are very powerful features that can help us better design and process business logic. In actual development, we should flexibly use anonymous functions and closures, choose appropriate methods to handle business logic as needed, and improve code quality and efficiency.
The above is the detailed content of How to use PHP7's anonymous functions and closures to achieve more flexible and scalable business logic processing?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
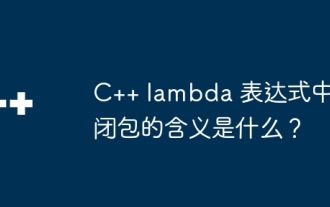
In C++, a closure is a lambda expression that can access external variables. To create a closure, capture the outer variable in the lambda expression. Closures provide advantages such as reusability, information hiding, and delayed evaluation. They are useful in real-world situations such as event handlers, where the closure can still access the outer variables even if they are destroyed.
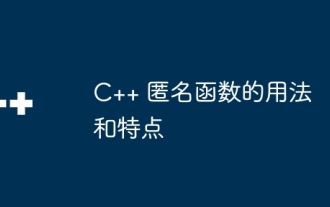
An anonymous function, also known as a lambda expression, is a function that does not specify a name and is used for one-time use or to pass a function pointer. Features include: anonymity, one-time use, closures, return type inference. In practice, it is often used for sorting or other one-time function calls.
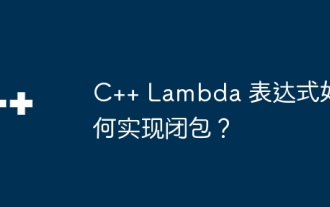
C++ Lambda expressions support closures, which save function scope variables and make them accessible to functions. The syntax is [capture-list](parameters)->return-type{function-body}. capture-list defines the variables to capture. You can use [=] to capture all local variables by value, [&] to capture all local variables by reference, or [variable1, variable2,...] to capture specific variables. Lambda expressions can only access captured variables but cannot modify the original value.
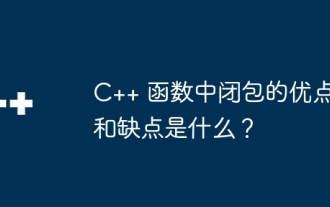
A closure is a nested function that can access variables in the scope of the outer function. Its advantages include data encapsulation, state retention, and flexibility. Disadvantages include memory consumption, performance impact, and debugging complexity. Additionally, closures can create anonymous functions and pass them to other functions as callbacks or arguments.

Go language function closures play a vital role in unit testing: Capturing values: Closures can access variables in the outer scope, allowing test parameters to be captured and reused in nested functions. Simplify test code: By capturing values, closures simplify test code by eliminating the need to repeatedly set parameters for each loop. Improve readability: Use closures to organize test logic, making test code clearer and easier to read.
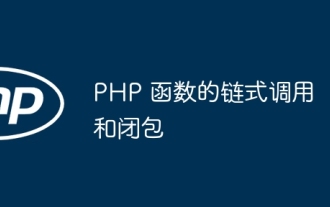
Yes, code simplicity and readability can be optimized through chained calls and closures: chained calls link function calls into a fluent interface. Closures create reusable blocks of code and access variables outside functions.
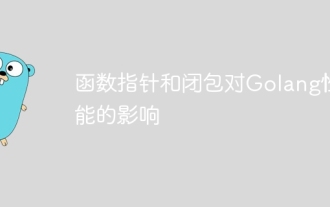
The impact of function pointers and closures on Go performance is as follows: Function pointers: Slightly slower than direct calls, but improves readability and reusability. Closures: Typically slower, but encapsulate data and behavior. Practical case: Function pointers can optimize sorting algorithms, and closures can create event handlers, but they will bring performance losses.
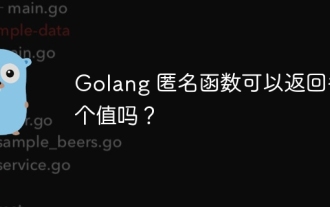
Yes, anonymous functions in Go language can return multiple values. Syntax: func(arg1,arg2,...,argN)(ret1,ret2,...,retM){//Function body}. Usage: Use the := operator to receive the return value; use the return keyword to return multiple values.
