How to implement data binding and rendering in Vue projects
How to implement data binding and rendering in Vue projects
Vue.js is a popular JavaScript framework that is widely used to build user interfaces. It provides a simple and efficient way to implement data binding and rendering. In this article, we will introduce in detail the method of implementing data binding and rendering in Vue projects, and provide specific code examples.
- Data binding
In Vue, data binding is the core concept to achieve two-way binding between data and interface. Through data binding, data can be dynamically bound to HTML templates, allowing it to automatically respond to data changes.
In Vue, there are two main ways of data binding: interpolation expressions and instructions.
a) Interpolation expression
Interpolation expression is the most basic form of data binding in Vue. It uses double curly braces {{}} to bind data to the HTML template. For example:
<div>{{ message }}</div>
In the above code, message
is a property of a Vue instance, which is bound to the div
element through an interpolation expression.
b) Instructions
Vue provides a series of instructions for implementing more complex data binding logic. Commonly used instructions include v-bind
, v-on
, and v-if
.
v-bind
directive is used to bind attributes of HTML elements. For example:
<img src="/static/imghw/default1.png" data-src="imageUrl" class="lazy" v-bind: alt="How to implement data binding and rendering in Vue projects" >
In the above code, imageUrl
is a property of a Vue instance, and the v-bind
instruction binds it to img# On the
src attribute of the ## element.
- v-on
directive is used to bind event listeners. For example:
<button v-on:click="handleClick">点击我</button>
handleClick is a method defined in the Vue instance, and the
v-on instruction binds it to the click of the button. on events.
- v-if
directive is used for conditional rendering. For example:
<div v-if="showMessage">{{ message }}</div>
showMessage is a property of a Vue instance. Only when
showMessage is
true,
div elements will be rendered.
- Data Rendering
- In Vue, data rendering is implemented through template syntax. Template syntax can dynamically render data into HTML templates to display data.
Interpolation expression can render data into HTML template. For example:
<div>{{ message }}</div>
message is a property of a Vue instance, which is rendered into the
div element through an interpolation expression.
Instructions can control the rendering logic of data. For example:
<div v-if="showMessage">{{ message }}</div>
showMessage is a property of a Vue instance. Only when
showMessage is
true,
div elements will be rendered.
In Vue, you can use the
v-for directive to implement loop rendering. For example:
<ul> <li v-for="item in list">{{ item }}</li> </ul>
list is an array containing multiple data, and the
v-for instruction renders each element in the array as
liElement.
Vue realizes the function of dynamically binding and rendering data into HTML templates through data binding and rendering. Data binding can be achieved through interpolation expressions and directives, and data rendering is achieved through template syntax. Vue provides a wealth of instructions and control statements, making data binding and rendering more flexible and efficient.
Vue数据绑定和渲染示例 <script> var app = new Vue({ el: '#app', data: { title: 'Vue数据绑定和渲染示例', list: ['数据1', '数据2', '数据3'] }, methods: { handleClick: function () { alert('按钮被点击了'); } } }); </script>{{ title }}
<button v-on:click="handleClick">点击我</button>
- {{ item }}
title Attributes are bound to the
h1 element, using the
v-for instruction to loop through each element in the
list array, using
v- The on directive binds the
handleClick method to the button's click event.
The above is the detailed content of How to implement data binding and rendering in Vue projects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
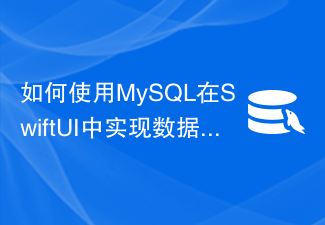
How to use MySQL to implement data binding function in SwiftUI. In SwiftUI development, data binding can realize automatic updating of interface and data, improving user experience. As a popular relational database management system, MySQL can store and manage large amounts of data. This article will introduce how to use MySQL to implement data binding function in SwiftUI. We will make use of Swift's third-party library MySQLConnector, which provides connections and queries to MySQL data.
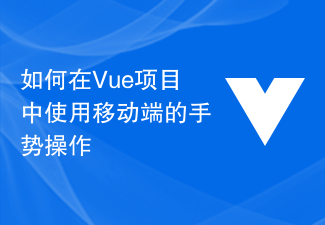
How to use mobile gesture operations in Vue projects With the popularity of mobile devices, more and more applications need to provide a more friendly interactive experience on the mobile terminal. Gesture operation is one of the common interaction methods on mobile devices, which allows users to complete various operations by touching the screen, such as sliding, zooming, etc. In the Vue project, we can implement mobile gesture operations through third-party libraries. The following will introduce how to use gesture operations in the Vue project and provide specific code examples. First, we need to introduce a special
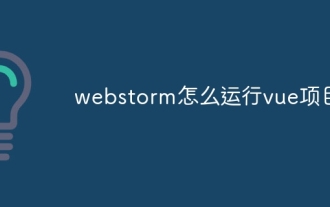
To run a Vue project using WebStorm, you can follow these steps: Install Vue CLI Create a Vue project Open WebStorm Start a development server Run the project View the project in the browser Debug the project in WebStorm
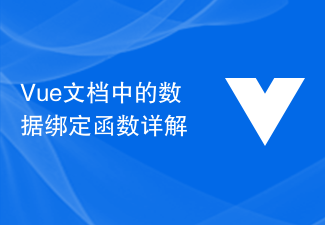
Vue is an open source JavaScript framework mainly used for building user interfaces. The core of Vue is data binding, which provides a convenient and efficient way to achieve two-way binding between data and views. Vue's data binding mechanism is handled through some special functions. These functions can help us automatically bind the data in the template to the corresponding properties in the JavaScript object, so that when the properties in the JavaScript object are modified, the data in the template will also automatically
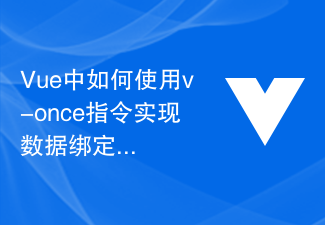
Vue is a popular front-end JavaScript framework that provides many instructions to simplify the data binding process. One of the very useful instructions is v-once. In this article, we will delve into the use of the v-once directive and how to implement data-bound one-time rendering in Vue. What is the v-once instruction? v-once is a directive in Vue. Its function is to cache the rendering results of elements or components so that their rendering process can be skipped in subsequent updates.
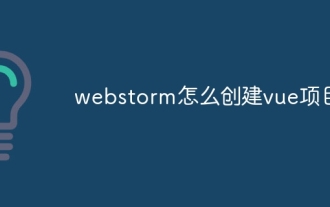
Create a Vue project in WebStorm by following these steps: Install WebStorm and the Vue CLI. Create a Vue project template in WebStorm. Create the project using Vue CLI commands. Import existing projects into WebStorm. Use the "npm run serve" command to run the Vue project.
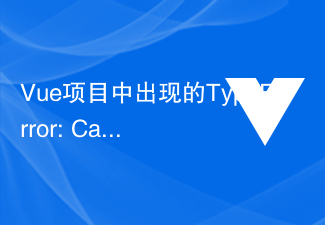
In Vue project development, we often encounter error messages such as TypeError:Cannotreadproperty'length'ofundefined. This error means that the code is trying to read a property of an undefined variable, especially a property of an array or object. This error usually causes application interruption and crash, so we need to deal with it promptly. In this article, we will discuss how to deal with this error. Check variable definitions in code
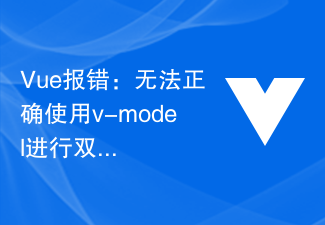
Vue error: v-model cannot be used correctly for two-way data binding. How to solve it? Introduction: Two-way data binding is a very common and powerful feature when developing with Vue. However, sometimes we may encounter a problem, that is, when we try to use v-model for two-way data binding, we encounter an error. This article describes the cause and solution of this problem, and provides a code example to demonstrate how to solve the problem. Problem Description: When we try to use v-model in Vue
