


PHP team collaboration process and code review mechanism following PSR2 and PSR4 specifications
PHP team collaboration process and code review mechanism following PSR2 and PSR4 specifications
Overview:
In a PHP team, in order to improve the readability of the code , maintainability and scalability, it is very important to follow PHP code specifications. This article will introduce how to follow the PSR2 and PSR4 specifications to establish an efficient PHP team collaboration process and code review mechanism, and provide some specific code examples.
1. PSR2 specification
PSR2 specification defines the coding style and formatting requirements of PHP code, including indentation, bracket spacing, line length, etc. Here are some common rules:
- Use 4 spaces for indentation.
- Each line of code should not exceed 80 characters.
- Use Unix-style newlines (
). - Add spaces before parentheses, but not inside parameter lists for function calls and control structures.
- The brackets of the control structure are on the same line as the first line of code, and there is no space before the brackets.
- Add spaces between operators, but do not add spaces for commas, semicolons, etc.
Team collaboration process:
During the team collaboration process, each member is required to conduct self-checks before submitting code to ensure that their code follows the PSR2 specification. A consistent code style can be achieved through the automatic formatting function of the IDE or code editor.
Code review mechanism:
Code review is an effective method that can identify potential problems and provide suggestions for improvement. The following is an example of a simple code review process:
- Self-review before code submission: Each developer should review his or her own code before submitting it to ensure that the code is of high quality and conforms to specifications.
- Selection of code reviewers: Select experienced and technically capable members from the team to serve as code reviewers.
- Code review process: The reviewer reviews the submitted code to check whether it complies with the PSR2 specification, whether the code logic is correct, whether there are performance issues, etc. Reviewers can use some tools to assist in the review, such as using code static analysis tools for inspection.
- Provide feedback and improvement suggestions: The reviewer provides feedback and improvement suggestions to the developer based on the review results. Communicate using notes, email, or online collaboration tools.
- Developers correct the code: The developer corrects the code based on the reviewer's feedback and suggestions, and submits the corrected code again.
- Recording and tracking of review results: Record the results of the review and suggestions for improvement. You can use tools to track the review process and record issues.
Code sample:
The following is a sample code for a simple PHP class that demonstrates how to follow the PSR2 specification:
<?php namespace App; class Calculator { protected $precision; public function __construct($precision = 2) { $this->precision = $precision; } public function add($a, $b) { return round($a + $b, $this->precision); } public function subtract($a, $b) { return round($a - $b, $this->precision); } }
The above sample code follows the indentation rules, line length PSR2 specifications such as limits and bracket spacing.
Summary:
Following the PSR2 and PSR4 specifications can improve the consistency and readability of PHP code, effectively improving team collaboration and code quality. Through team collaboration processes and code review mechanisms, we can better ensure consistent code quality among team members and provide opportunities for mutual learning and improvement. I believe that on the basis of following the specifications, the team's code quality will be significantly improved.
The above is the detailed content of PHP team collaboration process and code review mechanism following PSR2 and PSR4 specifications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
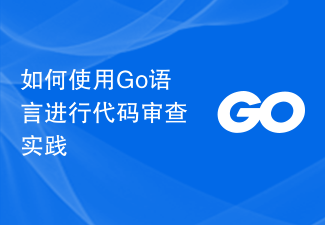
How to use Go language for code review practice Introduction: In the software development process, code review (CodeReview) is an important practice. By reviewing and analyzing each other's code, team members can identify potential problems, improve code quality, increase teamwork, and share knowledge. This article will introduce how to use Go language for code review practices, and attach code examples. 1. The importance of code review Code review is a best practice to promote code quality. It can find and correct potential errors in the code, improve the code
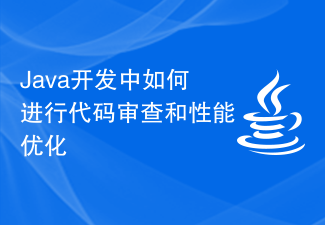
How to conduct code review and performance optimization in Java development requires specific code examples. In the daily Java development process, code review and performance optimization are very important links. Code review can ensure the quality and maintainability of the code, while performance optimization can improve the operating efficiency and response speed of the system. This article will introduce how to conduct Java code review and performance optimization, and give specific code examples. Code review Code review is the process of checking the code line by line as it is written and fixing potential problems and errors. the following
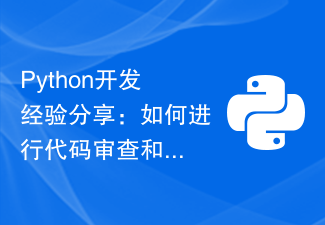
Python development experience sharing: How to conduct code review and quality assurance Introduction: In the software development process, code review and quality assurance are crucial links. Good code review can improve code quality, reduce errors and defects, and improve program maintainability and scalability. This article will share the experience of code review and quality assurance in Python development from the following aspects. 1. Develop code review specifications Code review is a systematic activity that requires a comprehensive inspection and evaluation of the code. In order to standardize code review
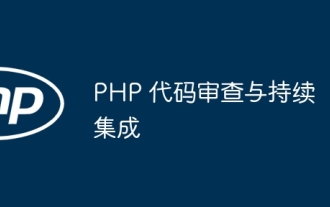
Yes, combining code reviews with continuous integration can improve code quality and delivery efficiency. Specific tools include: PHP_CodeSniffer: Check coding style and best practices. PHPStan: Detect errors and unused variables. Psalm: Provides type checking and advanced code analysis.
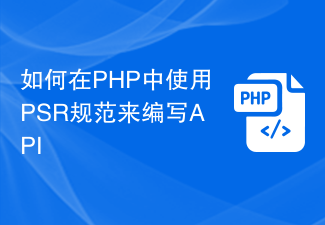
With the rapid development of the Internet, more and more enterprises and developers are beginning to use APIs (Application Programming Interfaces) to build their applications. APIs make it easier to interact between different applications and platforms. Therefore, API writing and design are becoming increasingly important. To achieve this goal, PHP has implemented PSR (PHP Standard Recommendation), which provides a set of standard specifications to help PHP programmers write more efficient and maintainable APIs. Below we will learn together how to use the PSR specification to compile
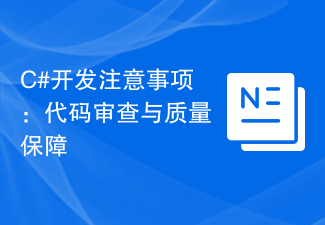
In the C# development process, code quality assurance is crucial. The quality of code directly affects the stability, maintainability and scalability of software. As an important quality assurance method, code review plays a role that cannot be ignored in software development. This article will focus on code review considerations in C# development to help developers improve code quality. 1. The purpose and significance of review Code review refers to the process of discovering and correcting existing problems and errors by carefully reading and inspecting the code. Its main purpose is to improve the
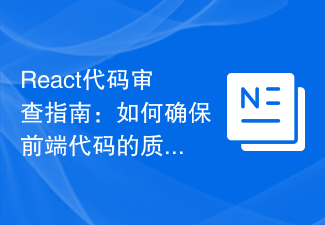
React Code Review Guide: How to Ensure the Quality and Maintainability of Front-End Code Introduction: In today’s software development, front-end code is increasingly important. As a popular front-end development framework, React is widely used in various types of applications. However, due to the flexibility and power of React, writing high-quality and maintainable code can become a challenge. To address this issue, this article will introduce some best practices for React code review and provide some concrete code examples. 1. Code style
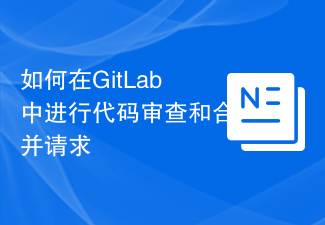
How to Conduct Code Reviews and Merge Requests in GitLab Code review is an important development practice that can help teams identify potential problems and improve code quality. In GitLab, through the merge request (MergeRequest) function, we can easily conduct code review and merge work. This article explains how to perform code reviews and merge requests in GitLab, while providing specific code examples. Preparation: Please make sure you have created a GitLab project and have the relevant
