How to safely handle user input data in PHP development
How to safely handle user input data in PHP development requires specific code examples
With the development of the Internet, user input data plays an important role in websites and applications Crucial role. However, handling user input data unsafely can cause serious security vulnerabilities, such as SQL injection, cross-site scripting attacks, etc. In order to ensure the security of websites and applications, in PHP development, we need to take some security measures to handle user input data. This article will introduce some common secure methods for handling user input data and provide specific code examples.
- Verify user input data
First, we need to verify whether the user input data meets the expected format and requirements. By using PHP's built-in validation functions and custom validation rules, we can ensure that the data entered by the user is valid. The following is a simple example to verify whether the user's username conforms to the specified format:
$username = $_POST['username']; // 使用filter_var函数验证用户名是否符合规定的格式 if (!filter_var($username, FILTER_VALIDATE_REGEXP, array("options"=>array("regexp"=>"/^[a-zA-Z0-9_]+$/")))) { echo "用户名不符合要求"; exit; } // 用户名验证通过,进行其他操作
- Clean user input data
Even if the user input data passes the verification, we Data sanitization is still required to prevent malicious code injection and other attacks. PHP provides multiple functions for cleaning user input data, such as strip_tags, htmlspecialchars, etc. The following is an example to clean user-entered comment content:
$comment = $_POST['comment']; // 使用strip_tags函数去除评论内容中的HTML标签 $comment = strip_tags($comment); // 使用htmlspecialchars函数将评论内容中的特殊字符转义 $comment = htmlspecialchars($comment, ENT_QUOTES); // 对评论内容进行进一步处理
- Using parameterized queries
When we need to pass user-input data to the database as query conditions, Parameterized queries should be used to avoid SQL injection attacks. Parameterized queries ensure that user input data is properly escaped and processed, preventing malicious code injection. The following is an example of using parameterized queries:
$keyword = $_GET['keyword']; // 使用参数化查询来查询数据库 $stmt = $pdo->prepare('SELECT * FROM articles WHERE title LIKE ?'); $keyword = "%{$keyword}%"; $stmt->execute([$keyword]); // 处理查询结果
- Preventing cross-site scripting attacks
In user input data, special attention needs to be paid to whether the content entered by the user Contains malicious scripts. In order to prevent cross-site scripting attacks (XSS attacks), we need to escape and filter user input. The following is an example to prevent user input from being executed as a malicious script:
$content = $_POST['content']; // 使用htmlspecialchars函数将用户输入的内容进行转义 $content = htmlspecialchars($content, ENT_QUOTES); // 使用strip_tags函数去除用户输入内容中的HTML标签 $content = strip_tags($content); // 对内容进行进一步处理
- Using security modules and frameworks
In addition to the above security processing methods, we You can also use PHP's security modules and frameworks to enhance the security of user-entered data. For example, using third-party libraries such as Laravel, Symfony, etc. can provide more advanced security measures, such as automatic filtering of dangerous characters, request verification, CSRF tokens, etc.
To sum up, the security of handling user input data in PHP development is very important. We can effectively secure our websites and applications by validating user input, sanitizing data, using parameterized queries, preventing cross-site scripting attacks, and using security modules and frameworks. However, no matter what measures are taken, we should always remain vigilant about user input data and promptly repair and update security measures to deal with changing security threats.
The above is the detailed content of How to safely handle user input data in PHP development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
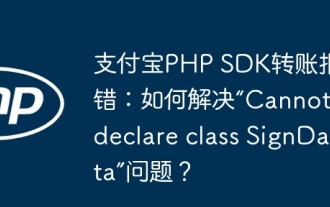
Alipay PHP...
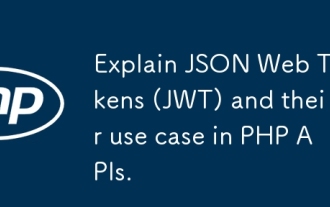
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
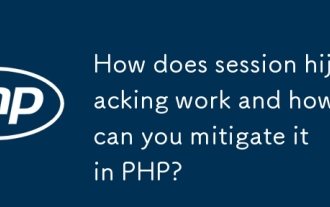
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
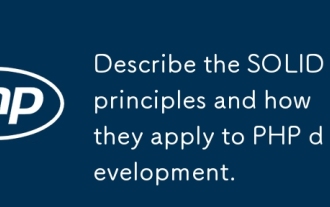
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
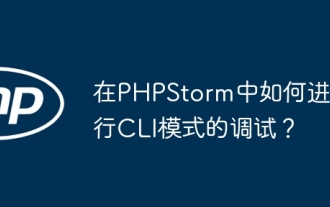
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
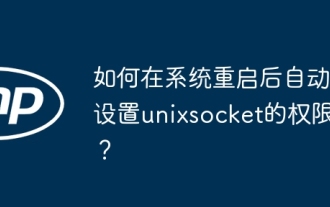
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
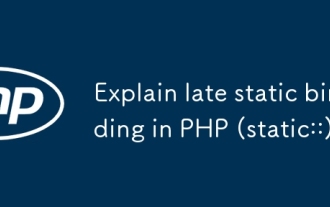
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
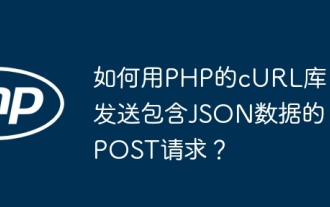
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
