


How to handle concurrent requests and task processing in PHP development
How to handle concurrent requests and task processing in PHP development
In modern Web development, it is often necessary to handle a large number of concurrent requests and tasks. When users send multiple requests at the same time, the server needs to be able to process these requests at the same time to improve the system's concurrency capability and response speed. At the same time, the processing of background tasks is also an important issue, such as asynchronous processing tasks, scheduled tasks, etc. In PHP development, the following methods can be used to handle concurrent requests and task processing.
- Use multi-threads or processes to handle concurrent requests
PHP does not natively support multi-threading, but you can use third-party extensions to achieve multi-threading functionality. A commonly used extension is pthreads. The following is a simple sample code:
<?php class MyThread extends Thread { private $url; public function __construct($url) { $this->url = $url; } public function run() { // 处理请求任务 $response = file_get_contents($this->url); echo "Response: " . $response . PHP_EOL; } } $urls = [ "http://example.com", "http://example.net", "http://example.org" ]; $threads = []; foreach ($urls as $url) { $thread = new MyThread($url); $thread->start(); $threads[] = $thread; } // 等待所有线程执行完成 foreach ($threads as $thread) { $thread->join(); } ?>
- Use asynchronous requests to handle concurrent requests
In PHP, you can use the curl library for asynchronous request processing. The following is a sample code implemented using the curl_multi_exec function:
<?php $urls = [ "http://example.com", "http://example.net", "http://example.org" ]; $curls = []; foreach ($urls as $url) { $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_multi_add_handle($multi_handle, $ch); $curls[] = $ch; } $running = null; do { curl_multi_exec($multi_handle, $running); } while ($running > 0); foreach ($curls as $ch) { $response = curl_multi_getcontent($ch); echo "Response: " . $response . PHP_EOL; curl_multi_remove_handle($multi_handle, $ch); curl_close($ch); } curl_multi_close($multi_handle); ?>
- Use message queue to process background tasks
Message queue is a commonly used method to solve concurrent task processing. In PHP, you can use various message queue tools, such as RabbitMQ, Redis, etc. The following is a sample code that uses RabbitMQ to handle background tasks:
<?php // 创建连接和通道 $connection = new AMQPStreamConnection('localhost', 5672, 'guest', 'guest'); $channel = $connection->channel(); // 声明队列 $channel->queue_declare('task_queue', false, true, false, false); $tasks = [ "Task 1", "Task 2", "Task 3" ]; foreach ($tasks as $task) { // 发送消息到队列 $msg = new AMQPMessage($task, ['delivery_mode' => 2]); $channel->basic_publish($msg, '', 'task_queue'); echo "Sent task: " . $task . PHP_EOL; } $channel->close(); $connection->close(); ?>
The above are several commonly used methods and code examples for handling concurrent requests and task processing. Developers can choose the ones that suit them based on actual needs. Methods. These methods can help improve the system's concurrency capabilities and processing efficiency, and provide better user experience and system performance.
The above is the detailed content of How to handle concurrent requests and task processing in PHP development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
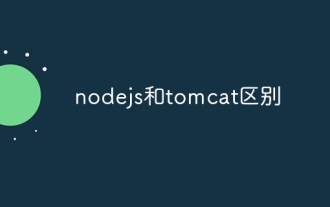
The main differences between Node.js and Tomcat are: Runtime: Node.js is based on JavaScript runtime, while Tomcat is a Java Servlet container. I/O model: Node.js uses an asynchronous non-blocking model, while Tomcat is synchronous blocking. Concurrency handling: Node.js handles concurrency through an event loop, while Tomcat uses a thread pool. Application scenarios: Node.js is suitable for real-time, data-intensive and high-concurrency applications, and Tomcat is suitable for traditional Java web applications.
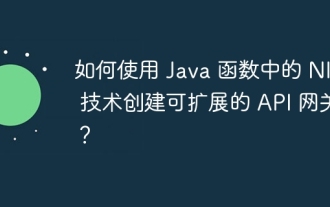
Answer: Using NIO technology you can create a scalable API gateway in Java functions to handle a large number of concurrent requests. Steps: Create NIOChannel, register event handler, accept connection, register data, read and write handler, process request, send response

Yes, Node.js is a backend development language. It is used for back-end development, including handling server-side business logic, managing database connections, and providing APIs.
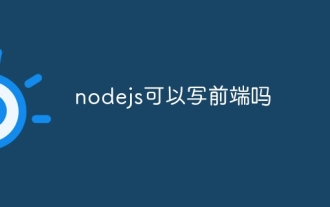
Yes, Node.js can be used for front-end development, and key advantages include high performance, rich ecosystem, and cross-platform compatibility. Considerations to consider are learning curve, tool support, and small community size.
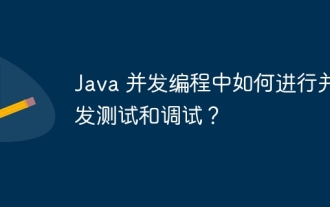
Concurrency testing and debugging Concurrency testing and debugging in Java concurrent programming are crucial and the following techniques are available: Concurrency testing: Unit testing: Isolate and test a single concurrent task. Integration testing: testing the interaction between multiple concurrent tasks. Load testing: Evaluate an application's performance and scalability under heavy load. Concurrency Debugging: Breakpoints: Pause thread execution and inspect variables or execute code. Logging: Record thread events and status. Stack trace: Identify the source of the exception. Visualization tools: Monitor thread activity and resource usage.
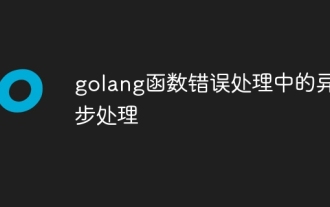
In Go functions, asynchronous error handling uses error channels to asynchronously pass errors from goroutines. The specific steps are as follows: Create an error channel. Start a goroutine to perform operations and send errors asynchronously. Use a select statement to receive errors from the channel. Handle errors asynchronously, such as printing or logging error messages. This approach improves the performance and scalability of concurrent code because error handling does not block the calling thread and execution can be canceled.
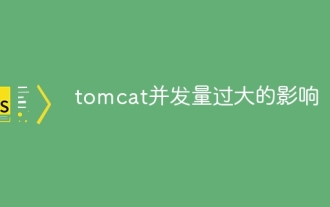
High concurrency in Tomcat leads to performance degradation and stability issues, including thread pool exhaustion, resource contention, deadlocks, and memory leaks. Mitigation measures include: adjusting thread pool settings, optimizing resource usage, monitoring server metrics, performing load testing, and using a load balancer.
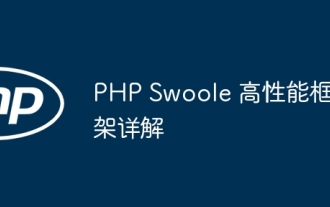
Swoole is a concurrency framework based on PHP coroutines, which has the advantages of high concurrency processing capabilities, low resource consumption, and simplified code development. Its main features include: coroutine concurrency, event-driven networks and concurrent data structures. By using the Swoole framework, developers can greatly improve the performance and throughput of web applications to meet the needs of high-concurrency scenarios.
