


How to optimize data storage and access performance in PHP development
How to optimize data storage and access performance in PHP development
In the PHP development process, the optimization of data storage and access performance is very important. Good optimization can improve the system's response speed, reduce resource consumption, and improve user experience. This article will introduce some common optimization techniques and provide specific code examples.
- Reasonable selection of database engines
When selecting a database engine, you need to make a selection based on specific business needs and scenarios. If you need high-performance read operations, you can consider choosing the MyISAM engine; if you need high concurrency and transaction support, you can choose the InnoDB engine. In addition, you can also optimize performance by adjusting database parameters, such as adjusting buffer size, thread pool size, etc.
- Use caching technology
Cache technology can significantly improve the response speed of the system and reduce the number of database accesses. Common caching technologies include memory caching and file caching. In PHP, you can use memory caching tools such as Memcached and Redis, or you can use file caching to store some frequently accessed data.
The following is a sample code for caching using Memcached:
$memcached = new Memcached(); $memcached->addServer('localhost', 11211); $key = 'data_key'; $data = $memcached->get($key); if (!$data) { // 如果缓存中不存在,则从数据库中获取数据 $data = getDataFromDatabase(); // 将数据存储到缓存中,缓存有效期为1小时 $memcached->set($key, $data, 3600); } // 使用$data进行后续处理
- Use indexes to improve query performance
In database design, adding indexes appropriately can significantly To improve query performance. Typically, you can add indexes to fields that are frequently used in query conditions. In MySQL, you can use the CREATE INDEX statement to create an index.
The following is a sample code for creating an index:
CREATE INDEX idx_name ON table_name (column_name);
- Batch data operation
In development, batch data operations are often required, such as inserting a large number of data or update multiple records. At this time, using batch operations can reduce the number of interactions with the database and improve performance.
The following is a sample code for using batch insertion of data:
$values = array(); for ($i = 1; $i <= 1000; $i++) { $values[] = "('name{$i}', {$i})"; } $sql = "INSERT INTO table_name (name, age) VALUES " . implode(',', $values); $result = mysqli_query($conn, $sql); if ($result) { echo '插入成功'; } else { echo '插入失败'; }
- Avoid unnecessary queries
When performing data queries, you need to avoid unnecessary query. For example, caching technology can be used to cache query results to avoid repeated queries; JOIN statements can be used to merge multiple queries into one query to reduce the burden on the database; EXPLAIN statements can also be used to analyze the execution plan of query statements and optimize query efficiency.
To sum up, by choosing the appropriate database engine, using caching technology, optimizing query statements and other methods, you can effectively optimize the data storage and access performance in PHP development. I hope the tips and examples provided in this article are helpful!
The above is the detailed content of How to optimize data storage and access performance in PHP development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










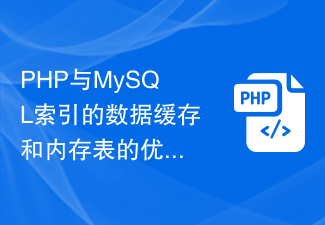
Optimization strategies for data caching and in-memory tables of PHP and MySQL indexes and their impact on query performance Introduction: PHP and MySQL are a very common combination when developing and optimizing database-driven applications. In the interaction between PHP and MySQL, index data caching and memory table optimization strategies play a crucial role in improving query performance. This article will introduce the optimization strategies for data caching and memory tables of PHP and MySQL indexes, and explain their impact on query performance in detail with specific code examples.
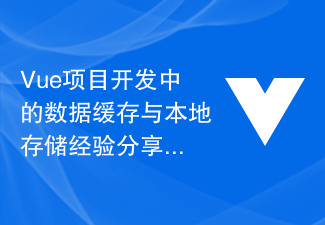
Data caching and local storage experience sharing in Vue project development In the development process of Vue project, data caching and local storage are two very important concepts. Data caching can improve application performance, while local storage can achieve persistent storage of data. In this article, I will share some experiences and practices in using data caching and local storage in Vue projects. 1. Data caching Data caching is to store data in memory so that it can be quickly retrieved and used later. In Vue projects, there are two commonly used data caching methods:
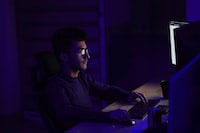
SpringBoot is a popular Java framework known for its ease of use and rapid development. However, as the complexity of the application increases, performance issues can become a bottleneck. In order to help you create a springBoot application as fast as the wind, this article will share some practical performance optimization tips. Optimize startup time Application startup time is one of the key factors of user experience. SpringBoot provides several ways to optimize startup time, such as using caching, reducing log output, and optimizing classpath scanning. You can do this by setting spring.main.lazy-initialization in the application.properties file
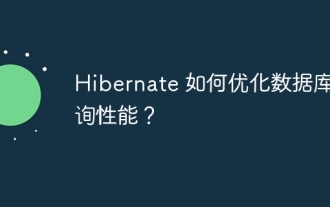
Tips for optimizing Hibernate query performance include: using lazy loading to defer loading of collections and associated objects; using batch processing to combine update, delete, or insert operations; using second-level cache to store frequently queried objects in memory; using HQL outer connections , retrieve entities and their related entities; optimize query parameters to avoid SELECTN+1 query mode; use cursors to retrieve massive data in blocks; use indexes to improve the performance of specific queries.
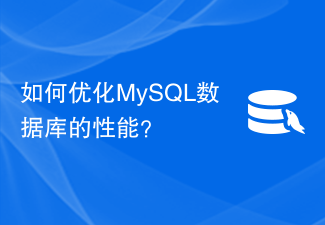
How to optimize the performance of MySQL database? In the modern information age, data has become an important asset for businesses and organizations. As one of the most commonly used relational database management systems, MySQL is widely used in all walks of life. However, as the amount of data increases and the load increases, the performance problems of the MySQL database gradually become apparent. In order to improve the stability and response speed of the system, it is crucial to optimize the performance of the MySQL database. This article will introduce some common MySQL database performance optimization methods to help readers
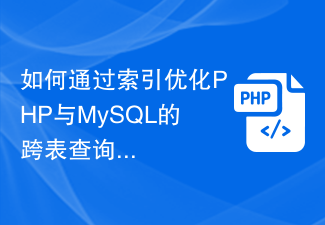
How to optimize cross-table queries and cross-database queries in PHP and MySQL through indexes? Introduction: In the development of applications that need to process large amounts of data, cross-table queries and cross-database queries are inevitable requirements. However, these operations are very resource intensive for database performance and can cause applications to slow down or even crash. This article will introduce how to optimize cross-table queries and cross-database queries in PHP and MySQL through indexes, thereby improving application performance. 1. Using indexes Index is a data structure in the database
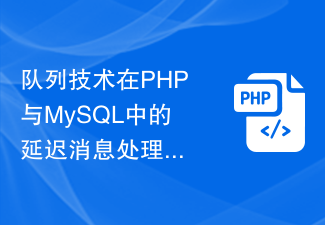
Application of queue technology in delayed message processing and data caching in PHP and MySQL Introduction: With the rapid development of the Internet, the demand for real-time data processing is getting higher and higher. However, traditional database operation methods often cause performance bottlenecks when processing large amounts of real-time data. In order to solve this problem, queue technology came into being, which can help us implement asynchronous processing of data and improve system performance and response speed. This article will introduce the application of queue technology in delayed message processing and data caching in PHP and MySQL, and through specific code
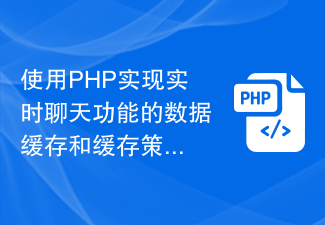
Data caching and caching strategies for real-time chat function using PHP Introduction: In modern social media and Internet applications, real-time chat function has become an important part of user interaction. In order to provide an efficient real-time chat experience, data caching and caching strategies have become the focus of developers. This article will introduce data caching and caching strategies for implementing real-time chat functionality using PHP, and provide relevant code examples. 1. The role of data caching Data caching is to reduce the burden on the database and improve the response speed of the system. in live chat
