Golang and FFmpeg: How to achieve audio noise reduction and gain
Golang and FFmpeg: How to implement audio noise reduction and gain
Overview
Audio processing is an important part in many application fields, such as speech recognition , audio editing, etc. In this regard, FFmpeg is a powerful open source tool that can be used to process audio and video files. Golang is a powerful and flexible programming language that can be used in conjunction with FFmpeg to implement various audio processing functions. This article will focus on how to use FFmpeg to implement audio noise reduction and gain functions in Golang.
Installing FFmpeg and Golang
Before starting, make sure you have installed FFmpeg and Golang. You can download and install FFmpeg from the official website (https://www.ffmpeg.org/). For Golang, you can download it from the official website and install it according to the instructions (https://golang.org/).
Import FFmpeg library
In Golang, you can use CGo technology to call FFmpeg functions by importing the C language library. First, we need to create a header file ffmpeg.go and copy the following content into the file:
package main /* #cgo pkg-config: libavformat libavcodec libavutil #include <libavformat/avformat.h> #include <libavcodec/avcodec.h> #include <libavutil/channel_layout.h> #include <libavutil/common.h> #include <libavutil/samplefmt.h> */ import "C"
The cgo
directive is used here to specify the FFmpeg library that needs to be linked.
Audio Noise Reduction
Audio noise reduction is a method of reducing background noise and other distracting sounds. The following is a sample code for using FFmpeg to implement audio noise reduction in Golang:
package main import "C" func main() { // 初始化FFmpeg C.av_register_all() C.avcodec_register_all() // 打开输入文件 var formatContext *C.AVFormatContext if C.avformat_open_input(&formatContext, C.CString("input.wav"), nil, nil) != 0 { panic("无法打开输入文件") } // 获取音频流索引 var audioStreamIndex C.int if C.avformat_find_stream_info(formatContext, nil) < 0 { panic("无法读取流信息") } for i := 0; i < int(formatContext.nb_streams); i++ { if formatContext.streams[i].codecpar.codec_type == C.AVMEDIA_TYPE_AUDIO { audioStreamIndex = C.int(i) break } } if audioStreamIndex == -1 { panic("找不到音频流") } // 打开解码器 codecParameters := formatContext.streams[audioStreamIndex].codecpar codec := C.avcodec_find_decoder(codecParameters.codec_id) codecContext := C.avcodec_alloc_context3(codec) if C.avcodec_open2(codecContext, codec, nil) < 0 { panic("无法打开解码器") } // 准备存储解码后数据的缓冲区 frame := C.av_frame_alloc() // 开始解码 packet := C.av_packet_alloc() for C.av_read_frame(formatContext, packet) == 0 { if packet.stream_index == audioStreamIndex { C.avcodec_send_packet(codecContext, packet) for C.avcodec_receive_frame(codecContext, frame) == 0 { // 在这里对音频帧进行降噪处理 // ... // 处理完后释放缓冲区 C.av_frame_unref(frame) } } C.av_packet_unref(packet) } // 清理资源 C.avformat_close_input(&formatContext) C.avcodec_free_context(&codecContext) C.av_frame_free(&frame) C.av_packet_free(&packet) }
This code first opens the input file, then gets the index of the audio stream, then opens the decoder and prepares a buffer to Store decoded audio data. Then, it starts to read audio frames in a loop, and perform noise reduction processing on the audio frames by calling FFmpeg's API. After processing is complete, release the audio frame's buffer. Finally, clean up the resources and close the input file.
Audio Gain
Audio gain is a method of increasing the volume of audio. The following is a sample code that uses FFmpeg to implement the audio gain function in Golang:
package main import "C" func main() { // 初始化FFmpeg C.av_register_all() C.avcodec_register_all() // 打开输入文件 var formatContext *C.AVFormatContext if C.avformat_open_input(&formatContext, C.CString("input.wav"), nil, nil) != 0 { panic("无法打开输入文件") } // 获取音频流索引 var audioStreamIndex C.int if C.avformat_find_stream_info(formatContext, nil) < 0 { panic("无法读取流信息") } for i := 0; i < int(formatContext.nb_streams); i++ { if formatContext.streams[i].codecpar.codec_type == C.AVMEDIA_TYPE_AUDIO { audioStreamIndex = C.int(i) break } } if audioStreamIndex == -1 { panic("找不到音频流") } // 打开解码器 codecParameters := formatContext.streams[audioStreamIndex].codecpar codec := C.avcodec_find_decoder(codecParameters.codec_id) codecContext := C.avcodec_alloc_context3(codec) if C.avcodec_open2(codecContext, codec, nil) < 0 { panic("无法打开解码器") } // 准备存储解码后数据的缓冲区 frame := C.av_frame_alloc() // 开始解码 packet := C.av_packet_alloc() for C.av_read_frame(formatContext, packet) == 0 { if packet.stream_index == audioStreamIndex { C.avcodec_send_packet(codecContext, packet) for C.avcodec_receive_frame(codecContext, frame) == 0 { // 在这里对音频帧进行增益处理 // ... // 处理完后释放缓冲区 C.av_frame_unref(frame) } } C.av_packet_unref(packet) } // 清理资源 C.avformat_close_input(&formatContext) C.avcodec_free_context(&codecContext) C.av_frame_free(&frame) C.av_packet_free(&packet) }
This code is similar to the sample code for audio noise reduction, except that gain processing is performed before processing the audio frame. You can use FFmpeg's API to achieve the desired gain effect.
Summary
In this article, we introduced how to use FFmpeg to implement audio noise reduction and gain functions in Golang. These sample codes can be used as a starting guide to help you start using Golang and FFmpeg to process audio files. By using the power of FFmpeg and the flexibility of Golang, you can implement various complex audio processing operations. Hope these sample codes can be helpful to you!
The above is the detailed content of Golang and FFmpeg: How to achieve audio noise reduction and gain. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
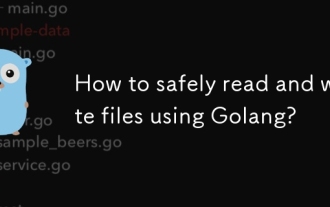
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
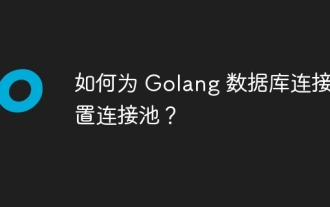
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
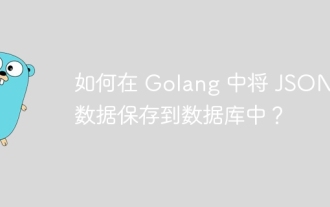
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
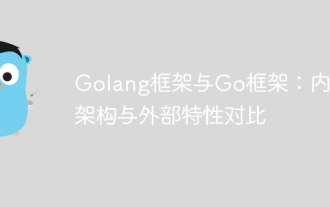
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
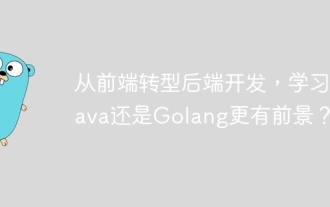
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
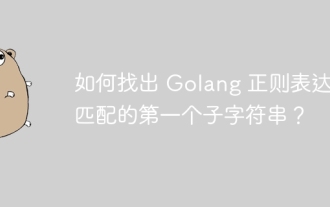
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
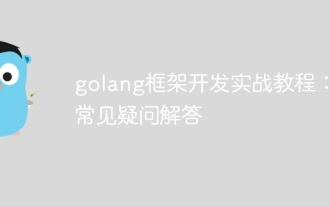
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
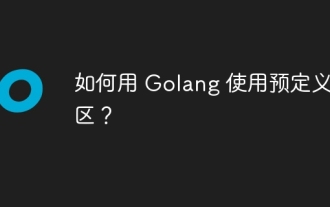
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
