


Implementing highly concurrent Select Channels Go programming technology and golang
Realize highly concurrent Select Channels Go programming technology and Golang
Introduction:
In the current highly concurrent programming environment, being able to effectively handle high concurrent requests is very important. The emergence of Golang gives developers a powerful tool to meet this challenge. This article will explore how to use Select Channels programming technology in Golang to achieve highly concurrent processing and provide specific code examples.
1. Select Channels in Golang
Channels in Golang are a synchronization mechanism used for communication between goroutines. By using channels, multiple coroutines can safely pass and receive data. Select Channels is a structure for selecting between multiple channels, similar to switch statements in other programming languages.
In Golang, you can use the Select statement to combine multiple channels to achieve high concurrency processing. The Select statement waits for any one of multiple channels to be ready and executes the corresponding code block. This mechanism allows us to implement non-blocking processing between different channels and improves the concurrency performance of the program.
2. Implementing Highly Concurrent Select Channels Go Programming Technology
The following is a sample code that uses Select Channels to achieve high concurrency:
package main import ( "fmt" "time" ) func main() { ch1 := make(chan string) ch2 := make(chan string) go func() { time.Sleep(time.Second * 1) ch1 <- "Hello from goroutine 1" }() go func() { time.Sleep(time.Second * 2) ch2 <- "World from goroutine 2" }() for i := 0; i < 2; i++ { select { case msg1 := <-ch1: fmt.Println(msg1) case msg2 := <-ch2: fmt.Println(msg2) } } }
In the above example, we created two Channels ch1
and ch2
, and send data to the channels in two anonymous functions respectively. The first anonymous function will send data to ch1
after 1 second, and the second anonymous function will send data to ch2
after 2 seconds.
In the main function, we use the Select statement to monitor two channels. Select will wait for the data in ch1
or ch2
to be ready first, and then execute the corresponding code block. Finally, the Select statement is repeatedly executed in the for
loop until the data in both channels is obtained.
By using Select Channels programming technology, we can achieve highly concurrent processing. Multiple coroutines can interact with different channels at the same time, improving the concurrency performance of the program.
Conclusion:
This article introduces how to use the Select Channels programming technology in Golang to achieve highly concurrent processing. By using the Select statement to combine multiple channels, we can achieve non-blocking processing between different channels and improve the concurrency performance of the program. Through concrete code examples, we show how to use Select Channels in Golang to achieve a high degree of concurrency. I hope this article can help readers better understand and apply Select Channels programming technology.
The above is the detailed content of Implementing highly concurrent Select Channels Go programming technology and golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
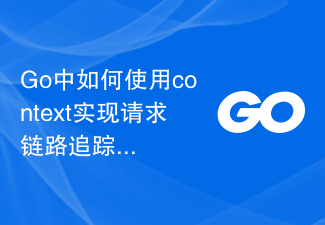
How to use context to implement request link tracking in Go. In the microservice architecture, request link tracking is a very important technology that is used to track the delivery and processing of a request between multiple microservices. In the Go language, we can use the context package to implement request link tracking. This article will introduce how to use context for request link tracking and give code examples. First, we need to understand the basic concepts and usage of the context package. The context package provides a mechanism
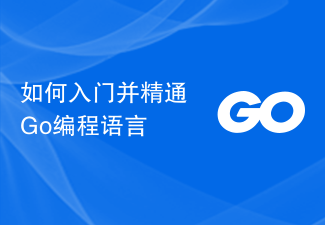
How to get started and become proficient in the Go programming language. The Go language is an open source programming language developed by Google. It has the characteristics of efficiency, simplicity, concurrency, etc., and has been favored by more and more developers in recent years. For those who want to learn and become proficient in the Go language, this article will provide some suggestions for getting started and in-depth learning, coupled with specific code examples, hoping to help readers better master this language. 1. Install the Go language at the entry stage. First, to learn the Go language, you need to install the Go compiler on your computer. Can be found on the official website

With the rapid development of Internet technology, more and more application scenarios require efficient caching mechanisms to improve system performance and response speed. In the Go language, there are many excellent caching libraries, such as go-cache, groupcache, etc., but how to use the cache in conjunction with the preheating mechanism is a topic worth discussing. This article will introduce what the preheating mechanism is, why the preheating mechanism is needed, the implementation of the preheating mechanism in the Go language cache library, and how to apply the preheating mechanism to improve cache usage efficiency. 1. What is the preheating mechanism?
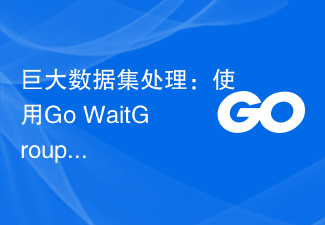
Huge Data Set Processing: Using GoWaitGroup to Optimize Performance Introduction: With the continuous development of technology, the growth of data volume is inevitable. Performance optimization becomes particularly important when dealing with huge data sets. This article will introduce how to use WaitGroup in Go language to optimize the processing of huge data sets. Understanding WaitGroupWaitGroup is a concurrency primitive in the Go language, which can be used to coordinate the execution of multiple goroutines. WaitGroup has three methods:
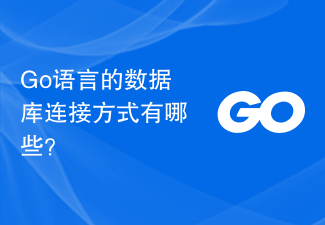
As a powerful programming language, Go language has rich database connection methods to facilitate developers to perform database operations in applications. In the Go language, common database connection methods mainly include using native database drivers and using ORM frameworks. These two methods will be introduced in detail below, with specific code examples. 1. Use native database driver Go language provides native database connection function through the database/sql package in the standard library, developers can directly manipulate data in the application
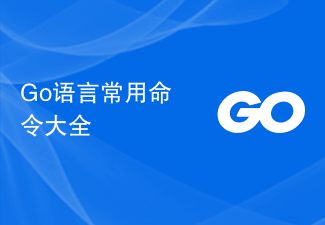
A list of commonly used commands in Go language. As a fast and reliable programming language, Go language has been widely praised and applied. In daily development, it is very important to master some commonly used commands. This article will introduce some common commands in Go language and provide specific code examples to help readers better understand and use them. gorun uses the gorun command to run Go programs directly. Enter the following code example at the command line: gorunmain.go This will compile and run the Go program named main.go
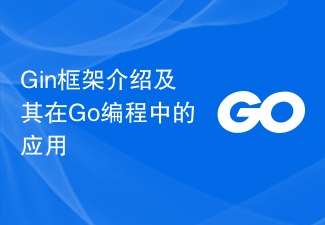
The Gin framework is a lightweight Go language web development framework that can quickly build high-performance web applications. Compared with other web frameworks, the Gin framework has many unique features and advantages. This article will introduce the characteristics, advantages and application of Gin framework in Go programming. 1. Characteristics of the Gin framework 1. Simple and easy to use The Gin framework is a very simple and easy-to-use Web framework. It does not require learning too much Web development knowledge and skills. Even developers who are not familiar with the Go language can get started quickly.
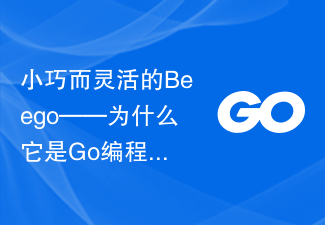
Go language has become one of the preferred languages for developers in the fields of cloud computing, big data and artificial intelligence in recent years. The Go language is simple, efficient, has powerful concurrency performance and excellent network programming features. It is also a language for rapid iterative development, which makes it an increasingly popular programming language. However, the Go language alone cannot realize its full potential. When we face multiple threads, multiple modules, multiple coroutines and complex business logic, we need a good framework to assist us in completing our development.
