


How to build large-scale data processing applications using React and Apache Hadoop
How to use React and Apache Hadoop to build large-scale data processing applications
In today's information age, data has become a key element in corporate decision-making and business development. With the explosive growth of data volume, processing large-scale data has become increasingly complex and difficult. To deal with such challenges, developers need to use powerful technologies and tools to process massive amounts of data. This article will introduce how to use React and Apache Hadoop to build large-scale data processing applications, and provide specific code examples.
React is a JavaScript library for building user interfaces. Its main advantage is its componentization and reusability. React handles user interface updates efficiently and provides a wealth of tools and libraries to simplify front-end development. Apache Hadoop is an open source software framework for distributed storage and processing of large-scale data. It provides important components such as HDFS (Hadoop Distributed File System) and MapReduce (for distributed computing), which can easily process and analyze large-scale data.
First, we need to build a React front-end application. You can use create-react-app to quickly create a React project. Next, we need to introduce some necessary libraries, such as react-router to handle page routing, axios for data interaction with the backend, etc.
In React applications, we can use RESTful API to access backend data. In order to achieve this, we can use the axios library in the React component to initiate HTTP requests and handle the response from the backend. The following is a sample code that demonstrates how to obtain data from the backend and display it on the page:
import React, { useState, useEffect } from 'react'; import axios from 'axios'; const DataComponent = () => { const [data, setData] = useState([]); useEffect(() => { axios.get('/api/data') .then(response => { setData(response.data); }) .catch(error => { console.error(error); }); }, []); return ( <div> {data.map(item => ( <p>{item.name}</p> ))} </div> ); };
In the above code, we initiated a GET request through the axios library to obtain data from the backend/api/data . When the data is obtained successfully, the data is assigned to the data variable of useState, and then the data is traversed and displayed on the page.
Next, we need to integrate with Apache Hadoop. First, we need to build a data processing cluster on Apache Hadoop. Depending on the actual situation, you can choose to use some key components of Hadoop, such as HDFS and MapReduce. You can use hadoop2.7.1 version for demonstration.
In React applications, we can use the hadoop-streaming library to convert data processing logic into MapReduce tasks. The following is a sample code that demonstrates how to use the hadoop-streaming library to apply data processing logic to a Hadoop cluster:
$ hadoop jar hadoop-streaming-2.7.1.jar -input input_data -output output_data -mapper "python mapper.py" -reducer "python reducer.py"
In the above code, we use the hadoop-streaming library to run a MapReduce task. The input data is located in the input_data directory, and the output results will be saved in the output_data directory. mapper.py and reducer.py are the actual data processing logic and can be written in Python, Java, or other Hadoop-enabled programming languages.
In mapper.py, we can use the input stream provided by Hadoop to read the data, and use the output stream to send the processing results to reducer.py. The following is a sample code that demonstrates how to use the input and output streams provided by Hadoop in mapper.py:
import sys for line in sys.stdin: # process input data # ... # emit intermediate key-value pairs print(key, value)
In reducer.py, we can use the input stream provided by Hadoop to read mapper.py output, and use the output stream to save the final result to the Hadoop cluster. The following is a sample code that demonstrates how to use the input and output streams provided by Hadoop in reducer.py:
import sys for line in sys.stdin: # process intermediate key-value pairs # ... # emit final key-value pairs print(key, value)
In summary, using React and Apache Hadoop to build large-scale data processing applications can achieve the separation of front-end and back-end and parallel computing advantages. Through React's componentization and reusability, developers can quickly build user-friendly front-end interfaces. The distributed computing capabilities provided by Apache Hadoop can process massive amounts of data and accelerate data processing efficiency. Developers can use the powerful functions of React and Apache Hadoop to build large-scale data processing applications based on actual needs.
The above is just an example, actual data processing applications may be more complex. I hope this article can provide readers with some ideas and directions to help them better use React and Apache Hadoop to build large-scale data processing applications.
The above is the detailed content of How to build large-scale data processing applications using React and Apache Hadoop. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
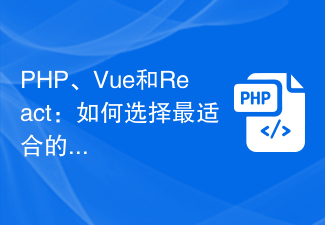
PHP, Vue and React: How to choose the most suitable front-end framework? With the continuous development of Internet technology, front-end frameworks play a vital role in Web development. PHP, Vue and React are three representative front-end frameworks, each with its own unique characteristics and advantages. When choosing which front-end framework to use, developers need to make an informed decision based on project needs, team skills, and personal preferences. This article will compare the characteristics and uses of the three front-end frameworks PHP, Vue and React.
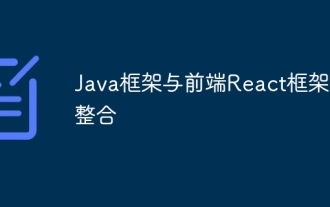
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
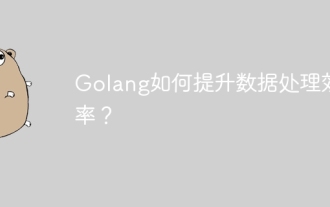
Golang improves data processing efficiency through concurrency, efficient memory management, native data structures and rich third-party libraries. Specific advantages include: Parallel processing: Coroutines support the execution of multiple tasks at the same time. Efficient memory management: The garbage collection mechanism automatically manages memory. Efficient data structures: Data structures such as slices, maps, and channels quickly access and process data. Third-party libraries: covering various data processing libraries such as fasthttp and x/text.
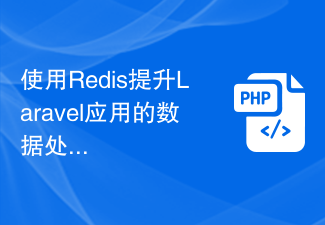
Use Redis to improve the data processing efficiency of Laravel applications. With the continuous development of Internet applications, data processing efficiency has become one of the focuses of developers. When developing applications based on the Laravel framework, we can use Redis to improve data processing efficiency and achieve fast access and caching of data. This article will introduce how to use Redis for data processing in Laravel applications and provide specific code examples. 1. Introduction to Redis Redis is a high-performance memory data
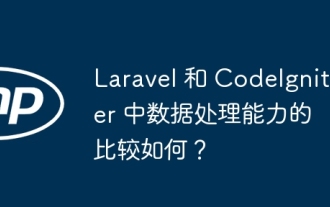
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
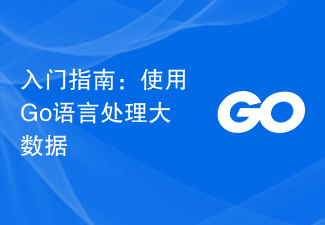
As an open source programming language, Go language has gradually received widespread attention and use in recent years. It is favored by programmers for its simplicity, efficiency, and powerful concurrent processing capabilities. In the field of big data processing, the Go language also has strong potential. It can be used to process massive data, optimize performance, and can be well integrated with various big data processing tools and frameworks. In this article, we will introduce some basic concepts and techniques of big data processing in Go language, and show how to use Go language through specific code examples.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
