How to implement database partitioning strategy in React Query?
How to implement database partitioning strategy in React Query?
Overview:
React Query is a very powerful state management library that can easily manage and synchronize your component state and backend data. When dealing with large amounts of data, it is very likely that the data will need to be partitioned according to some strategy. This article will introduce how to implement the database partitioning strategy in React Query and provide specific code examples.
Introduction to partitioning strategy:
The partitioning strategy of the database is to divide the data into different areas according to different conditions to achieve the purpose of improving query performance and optimizing storage space. A common partitioning strategy is to partition by time, for example, storing each month's data in a different table or collection. In React Query, we can use Query Keys to implement a similar partitioning strategy.
Steps to implement partitioning strategy:
- Define partitioning strategy: First, we need to define the partitioning strategy, for example, partitioning according to time, region or other conditions. In this example, we will partition by time, one for each month.
- Create Query Client: In React Query, we can manage queries and status by using
QueryClient
. First, we need to create a globalQueryClient
instance.
import { QueryClient, QueryClientProvider } from 'react-query'; const queryClient = new QueryClient(); function App() { return ( <QueryClientProvider client={queryClient}> {/* Application Components */} </QueryClientProvider> ); }
- Use Query Hook for data query: Next, we can use React Query’s
useQuery
hook to perform data query. When usinguseQuery
, we need to specify a unique Query Key, which will be used to identify the queried data. According to the partitioning strategy, we can design the Query Key as a string containing partition information.
import { useQuery } from 'react-query'; function MyComponent() { const queryKey = 'data:2022-01'; // 根据分区策略生成 Query Key const { isLoading, error, data } = useQuery(queryKey, fetchData); if (isLoading) { return <div>Loading...</div>; } if (error) { return <div>Error: {error.message}</div>; } return <div>Data: {data}</div>; }
- Update cache when data is updated: When React Query completes data query, it automatically stores the data in the cache. If we have new data that needs to be updated, we can use the
queryClient.setQueryData
method to update the data in the cache. According to the partition strategy, we need to update the corresponding cache data according to different partitions.
// 在某个函数中更新数据 const newData = 'New data from API'; const queryKey = 'data:2022-01'; // 根据分区策略生成 Query Key queryClient.setQueryData(queryKey, newData);
Through the above steps, we can implement data operations in React Query according to the partitioning strategy of the database.
Summary:
The partitioning strategy of the database can help us improve data query performance and manage data storage. By using React Query, we can easily implement the partitioning strategy of the database and use Query Keys in the code to split and manage the data. This gives us better scalability and flexibility when processing large amounts of data.
The above are the detailed steps and code examples on how to implement the database partitioning strategy in React Query. Hope this article helps you!
The above is the detailed content of How to implement database partitioning strategy in React Query?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
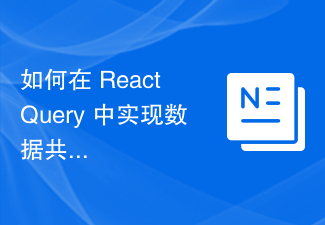
How to implement data sharing and permission management in ReactQuery? Advances in technology have made data management in front-end development more complex. In the traditional way, we may use state management tools such as Redux or Mobx to handle data sharing and permission management. However, after the emergence of ReactQuery, we can use it to deal with these problems more conveniently. In this article, we will explain how to implement data sharing and permissions in ReactQuery
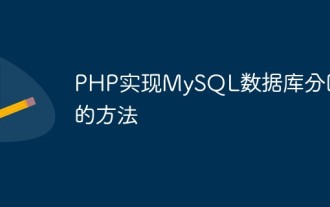
As business and data volumes continue to grow, database performance and availability have gradually become a real-time concern. As a mainstream database, MySQL sometimes needs to be partitioned when building a high-performance and high-availability system. This article will introduce how to implement MySQL database partitioning in PHP. 1. MySQL database partitioning MySQL database partitioning is a technology that divides data into different parts for storage. By spreading data across multiple hardware locations, MySQL database partitioning can greatly improve table performance.
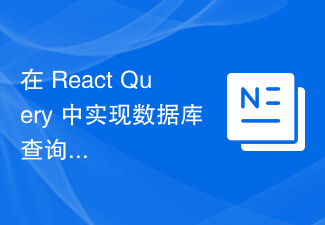
Implementing the error handling mechanism of database queries in ReactQuery ReactQuery is a library for managing and caching data, and it is becoming increasingly popular in the front-end field. In applications, we often need to interact with databases, and database queries may cause various errors. Therefore, implementing an effective error handling mechanism is crucial to ensure application stability and user experience. The first step is to install ReactQuery. Add it to the project using the following command: n
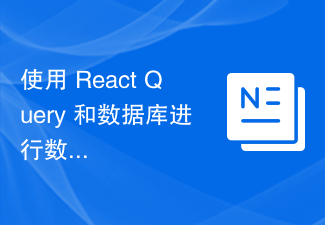
Introduction to data cache merging using ReactQuery and database: In modern front-end development, data management is a very important part. In order to improve performance and user experience, we usually need to cache the data returned by the server and merge it with local database data. ReactQuery is a very popular data caching library that provides a powerful API to handle data query, caching and updating. This article will introduce how to use ReactQuery and database
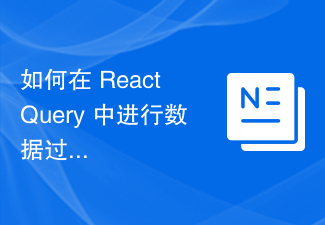
How to do data filtering and searching in ReactQuery? In the process of using ReactQuery for data management, we often encounter the need to filter and search data. These features can help us find and display data under specific conditions more easily. This article will introduce how to use filtering and search functions in ReactQuery and provide specific code examples. ReactQuery is a tool for querying data in React applications

Data Management with ReactQuery and Databases: A Best Practice Guide Introduction: In modern front-end development, managing data is a very important task. As users' demands for high performance and stability continue to increase, we need to consider how to better organize and manage application data. ReactQuery is a powerful and easy-to-use data management tool that provides a simple and flexible way to handle the retrieval, update and caching of data. This article will introduce how to use ReactQ
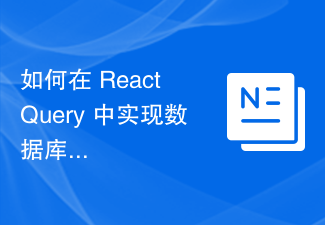
How to achieve separation of read and write in database in ReactQuery? In modern front-end development, the separation of reading and writing in the database is an important architectural design consideration. ReactQuery is a powerful state management library that can optimize the data acquisition and management process of front-end applications. This article will introduce how to use ReactQuery to achieve separation of read and write in the database, and provide specific code examples. The core concepts of ReactQuery are Query, Mutatio
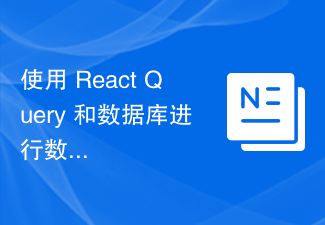
Title: Data Encryption and Decryption Using ReactQuery and Database Introduction: This article will introduce how to use ReactQuery and database for data encryption and decryption. We will use ReactQuery as the data management library and combine it with the database to perform data encryption and decryption operations. By combining these two technologies, we can securely store and transmit sensitive data, and perform encryption and decryption operations when needed to ensure data security. Text: 1. ReactQue
