C++ program to convert binary number to Gray code using recursion
Gray code or reflected binary code is a special type of binary representation of a number in which two consecutive values differ in only one bit. For example, the binary equivalents of 1 and 2 are 01 and 10, where two bits are changing. But in Gray code, 1 is 01, 2 is 11, and only one bit is changing. In this article, we will see how to convert a given binary number to its Gray code equivalent using recursion in C.
Pass numbers as decimal integers
In the first example, we provide decimal numbers. The numbers are only 0 and 1, but the numbers are in decimal. For example, if we want to pass 6 as input, we pass 110 (one hundred and ten in decimal), which is equivalent to 6 in binary. The program returns output similarly.
algorithm
- Define a function solve(), which will take a binary number
- If n is 0, then
- Return 0
- If it ends
- Last := the last digit of n
- second_last = the penultimate digit of n
- If the last digit and the penultimate digit are different, then
- Enter 1 and call solve(n cuts the last bit)
- otherwise
- Enter 0 and call solve(n cuts the last bit)
- If it ends
- solve() function ends
Example
#include <iostream> using namespace std; int solve( int n ) { if( n == 0 ) return 0; int last = n % 10; int second_last = (n / 10) % 10; if( (last && !second_last) || (!last && second_last) ) { return (1 + 10 * solve( n / 10 )); } return (10 * solve( n / 10 )); } int main() { cout << "Gray code for the number 2 (10) is: " << solve( 10 ) << endl; cout << "Gray code for the number 6 (110) is: " << solve( 110 ) << endl; cout << "Gray code for the number 13 (1101) is: " << solve( 1101 ) << endl; cout << "Gray code for the number 93 (1011101) is: " << solve( 1011101 ) << endl; }
Output
Gray code for the number 2 (10) is: 11 Gray code for the number 6 (110) is: 101 Gray code for the number 13 (1101) is: 1011 Gray code for the number 93 (1011101) is: 1110011
in conclusion
Gray code or reflected binary code can be found by applying XOR operation to consecutive bits. The same thing is achieved by taking the last two digits of the given number, when they are not the same, call the function recursively and pass the number except the last digit, the result will be concatenated with 1, otherwise with 0, etc. And so on. In the example, we have provided input as an integer decimal number and the output is also in integer decimal format. The same problem can be solved by taking a string type input which can be used to provide larger input when required.
The above is the detailed content of C++ program to convert binary number to Gray code using recursion. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

The recursion depth of C++ functions is limited, and exceeding this limit will result in a stack overflow error. The limit value varies between systems and compilers, but is usually between 1,000 and 10,000. Solutions include: 1. Tail recursion optimization; 2. Tail call; 3. Iterative implementation.
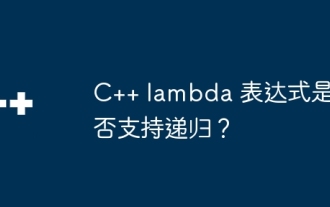
Yes, C++ Lambda expressions can support recursion by using std::function: Use std::function to capture a reference to a Lambda expression. With a captured reference, a Lambda expression can call itself recursively.

The recursive algorithm solves structured problems through function self-calling. The advantage is that it is simple and easy to understand, but the disadvantage is that it is less efficient and may cause stack overflow. The non-recursive algorithm avoids recursion by explicitly managing the stack data structure. The advantage is that it is more efficient and avoids the stack. Overflow, the disadvantage is that the code may be more complex. The choice of recursive or non-recursive depends on the problem and the specific constraints of the implementation.
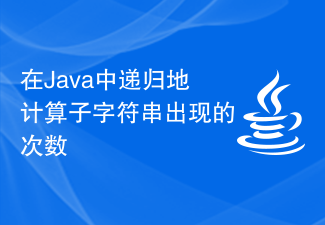
Given two strings str_1 and str_2. The goal is to count the number of occurrences of substring str2 in string str1 using a recursive procedure. A recursive function is a function that calls itself within its definition. If str1 is "Iknowthatyouknowthatiknow" and str2 is "know" the number of occurrences is -3. Let us understand through examples. For example, input str1="TPisTPareTPamTP", str2="TP"; output Countofoccurrencesofasubstringrecursi

Binary arithmetic is an operation method based on binary numbers. Its basic operations include addition, subtraction, multiplication and division. In addition to basic operations, binary arithmetic also includes logical operations, displacement operations and other operations. Logical operations include AND, OR, NOT and other operations, and displacement operations include left shift and right shift operations. These operations have corresponding rules and operand requirements.
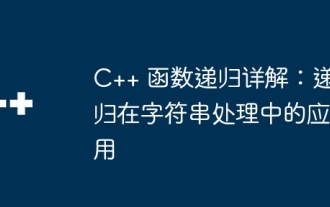
A recursive function is a technique that calls itself repeatedly to solve a problem in string processing. It requires a termination condition to prevent infinite recursion. Recursion is widely used in operations such as string reversal and palindrome checking.
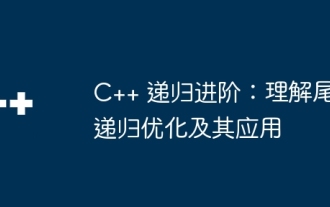
Tail recursion optimization (TRO) improves the efficiency of certain recursive calls. It converts tail-recursive calls into jump instructions and saves the context state in registers instead of on the stack, thereby eliminating extra calls and return operations to the stack and improving algorithm efficiency. Using TRO, we can optimize tail recursive functions (such as factorial calculations). By replacing the tail recursive call with a goto statement, the compiler will convert the goto jump into TRO and optimize the execution of the recursive algorithm.
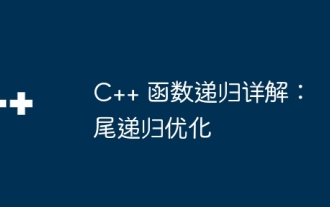
Recursive definition and optimization: Recursive: A function calls itself internally to solve difficult problems that can be decomposed into smaller sub-problems. Tail recursion: The function performs all calculations before making a recursive call, which can be optimized into a loop. Tail recursion optimization condition: recursive call is the last operation. The recursive call parameters are the same as the original call parameters. Practical example: Calculate factorial: The auxiliary function factorial_helper implements tail recursion optimization, eliminates the call stack, and improves efficiency. Calculate Fibonacci numbers: The tail recursive function fibonacci_helper uses optimization to efficiently calculate Fibonacci numbers.
