


PHP Message Queue Development Guide: Implementing a Distributed Cache Refresher
PHP Message Queue Development Guide: Implementing Distributed Cache Refresher
With the continuous development of Internet applications, caching technology plays an important role in improving system performance and response speed. important role. However, due to the high concurrency and distributed deployment characteristics of Internet applications, how to achieve cache consistency and timely updates has become a challenge. In this article, we will introduce how to use PHP message queue to develop a distributed cache refresher to achieve cache consistency and automatic refresh.
- Introduction to Message Queue
Message queue is a common distributed communication method. It sends messages to the queue, and then the consumer takes them out of the queue and processes them. Message queue has the characteristics of decoupling, asynchronousness, peak clipping, etc., and is very suitable for solving task scheduling and communication problems in high concurrency scenarios. - Using Redis as a message queue
Redis is a high-performance key-value storage system. It supports a variety of data structures and rich operations, and has excellent performance and reliability. We can use Redis as the message queue of PHP and realize the sending and consumption of messages by operating the List type of Redis. - The process of implementing a distributed cache refresher
First, we need to define a cache refresh message structure, including the cache key and refresh time. Then, when a cache needs to be refreshed, the refresh message is sent to the Redis queue. Consumers can obtain and process cache refresh messages in real time and update the cache by listening to the Redis queue. - Producer implementation
In PHP, we can use the Predis library to operate Redis. First, we need to configure the Redis connection information, and then create the Redis connection object. Next, we can use the lpush command to push the cache refresh message to the Redis queue. For example:
<?php require 'predis/autoload.php'; PredisAutoloader::register(); $redis = new PredisClient([ 'scheme' => 'tcp', 'host' => '127.0.0.1', 'port' => 6379, ]); $key = 'user:1'; // 缓存的key $time = time(); // 刷新时间 $message = json_encode(['key' => $key, 'time' => $time]); $redis->lpush('cache_refresh', $message); // 推送消息到队列 echo "缓存刷新消息已发送"; ?>
- Consumer implementation
Consumers can use a background process to listen to the Redis queue, obtain and process cache refresh messages in real time. In PHP, we can use the pcntl extension to implement multi-process programming. First, we need to create a parent process, and then create multiple child processes through the fork function. These sub-processes can obtain cache refresh messages and update the cache in real time by listening to the Redis queue.
<?php require 'predis/autoload.php'; PredisAutoloader::register(); $redis = new PredisClient([ 'scheme' => 'tcp', 'host' => '127.0.0.1', 'port' => 6379, ]); $pid = pcntl_fork(); if ($pid == -1) { die("子进程创建失败"); } elseif ($pid > 0) { // 父进程 exit(); } else { // 子进程 $redis->subscribe(['cache_refresh'], function ($redis, $channel, $message) { // 处理缓存刷新消息 $data = json_decode($message, true); $key = $data['key']; $time = $data['time']; // 刷新缓存逻辑 // ... }); } ?>
- Summary
By using PHP message queue to implement a distributed cache refresher, we can solve the cache consistency and automatic refresh problems in high concurrency scenarios. Using Redis as a message queue, you can easily send and receive messages by operating the List type of Redis. At the same time, multi-process programming can realize concurrent processing of consumers and improve the system's processing power and response speed.
The above is the detailed content of PHP Message Queue Development Guide: Implementing a Distributed Cache Refresher. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
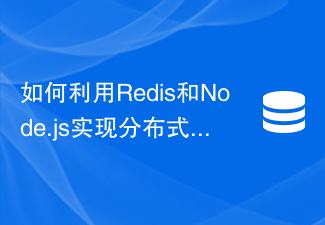
How to use Redis and Node.js to implement distributed caching functions. Redis is an open source in-memory database that provides fast and scalable key-value storage and is often used in scenarios such as caching, message queues, and data storage. Node.js is a JavaScript runtime based on the ChromeV8 engine, suitable for high-concurrency web applications. This article will introduce how to use Redis and Node.js to implement the distributed cache function, and help readers understand and practice it through specific code examples.
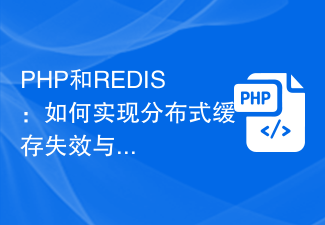
PHP and REDIS: How to implement distributed cache invalidation and update Introduction: In modern distributed systems, cache is a very important component, which can significantly improve the performance and scalability of the system. At the same time, cache invalidation and update is also a very important issue, because if the invalidation and update of cache data cannot be handled correctly, it will lead to system data inconsistency. This article will introduce how to use PHP and REDIS to implement distributed cache invalidation and update, and provide relevant code examples. 1. What is RED
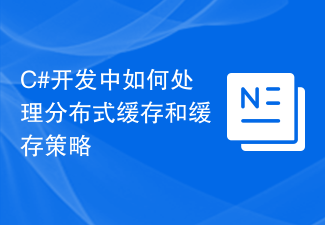
How to deal with distributed caching and caching strategies in C# development Introduction: In today's highly interconnected information age, application performance and response speed are crucial to user experience. Caching is one of the important ways to improve application performance. In distributed systems, dealing with caching and developing caching strategies becomes even more important because the complexity of distributed systems often creates additional challenges. This article will explore how to deal with distributed caching and caching strategies in C# development, and demonstrate the implementation through specific code examples. 1. Introduction using distributed cache
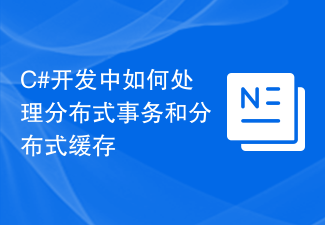
How to handle distributed transactions and distributed cache in C# development requires specific code examples Summary: In distributed systems, transaction processing and cache management are two crucial aspects. This article will introduce how to handle distributed transactions and distributed cache in C# development, and give specific code examples. Introduction As the scale and complexity of software systems increase, many applications adopt distributed architectures. In distributed systems, transaction processing and cache management are two key challenges. Transaction processing ensures data consistency, while cache management improves system
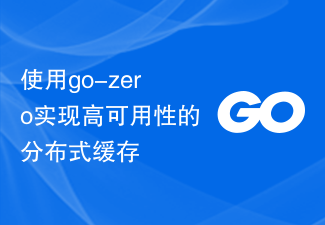
With the development of web applications, more and more attention is turning to how to improve application performance. The role of caching is to offset high traffic and busy loads and improve the performance and scalability of web applications. In a distributed environment, how to implement high-availability caching has become an important technology. This article will introduce how to use some tools and frameworks provided by go-zero to implement high-availability distributed cache, and briefly discuss the advantages and limitations of go-zero in practical applications. 1. What is go-
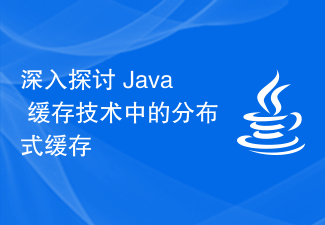
In the current Internet environment of high concurrency and big data, caching technology has become one of the important means to improve system performance. In Java caching technology, distributed caching is a very important technology. So what is distributed cache? This article will delve into distributed caching in Java caching technology. 1. Basic concepts of distributed cache Distributed cache refers to a cache system that stores cache data on multiple nodes. Among them, each node contains a complete copy of cached data and can back up each other. When one of the nodes fails,
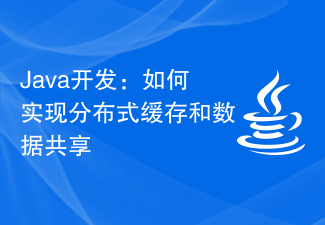
Java Development: How to Implement Distributed Caching and Data Sharing Introduction: As the scale of the system continues to expand, distributed architecture has become a common choice for enterprise application development. In distributed systems, efficient caching and data sharing is one of the key tasks. This article will introduce how to use Java to develop distributed caching and data sharing methods, and provide specific code examples. 1. Implementation of distributed cache 1.1Redis as a distributed cache Redis is an open source in-memory database that can be used as a distributed cache. The following is
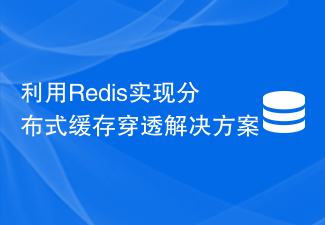
Using Redis to realize distributed cache penetration solution With the continuous development of Internet business, the amount of data access is also increasing. In order to improve the performance and user experience of the system, caching technology has gradually become an indispensable part, of which Redis is an essential part. An efficient and scalable caching middleware solution that is favored by developers. When using Redis as a distributed cache, in order to avoid performance problems caused by cache penetration, we need to implement a reliable solution. This article will introduce how to use Redis to achieve splitting
