Why do we need to use collections framework in Java?
An array is a collection that stores elements of the same type in consecutive memory allocations. They are used to represent lists of things like numbers, strings, etc.
grammar
<element-type>[] <array-name> = new <element-type>[<array-size>];
algorithm
To implement an array, please follow these steps
Step 1 − should be carefully considered in advance for each of the elements that will populate the required array Select the appropriate data type for individual elements.
Step 2 − In addition, determining the required capacity by considering specific usage requirements will be able to Choose an accurate and optimal array size.
< /里>Step 3 − Declare an array variable.
Step 4 - Access individual elements of the array. Just use the indexing operator [] and do whatever is necessary.
Step 5 - Perform relevant operations on each element contained in the array. Loops can be used to systematically iterate through them and perform the required tasks.
Example
is:Example
public class Main { public static void main(String[] args) { int[] marks = new int[3]; marks[0] = 75; marks[1] = 20; marks[2] = 87; for (int i = 0; i < marks.length; i++) { System.out.println("Marks of student " + (i + 1) + ": " + marks[i]); } int sum = 0; for (int i = 0; i < marks.length; i++) { sum += marks[i]; } double average = (double) sum / marks.length; System.out.println("Average marks: " + average); } }
Output
Marks of student 1: 75 Marks of student 2: 20 Marks of student 3: 87 Average marks: 60.66
Limitations of arrays in Java
The size of the array is fixed - The size of the array cannot be increased or decreased at runtime.
Arrays are not memory efficient - If the number of elements added to an array is less than the allocated size, memory may be wasted. p>
Array has no built-in available methods− Array does not have any built-in methods to perform common operations such as adding, searching, etc.
Arrays contain only data elements of the same type - Arrays can only store elements of the same type.
No underlying data structure - The idea of an array is not implemented using standard data structures. Therefore, no ready method support is available.
Collection framework in java
In Java, a framework aims to provide a standardized way, or we can say it provides a ready-made architecture to solve a specific problem or task by utilizing a set of abstract classes and interfaces and other components.
A collection is a combination of multiple individual objects as a whole. Java's collection framework provides several different classes and interfaces that can effectively represent collections. Common choices include ArrayList, LinkedList, HashSet, and TreeSet, which can be accessed through the java.util package.
grammar
The syntax depends on the specific class, for example -
The translation ofArrayList
is:ArrayList
ArrayList<T> list = new ArrayList<T>();
LinkedList
is:Linked List
LinkedList<T> list = new LinkedList<T>();
algorithm
To implement, follow these steps -
Step 1 - Select the appropriate collection class based on the requirements of the program.
Step 2 - Import the classes required for the collection.
Step 3 - Declare a variable of the collection class.
Step 4 - Instantiate the collection using the appropriate constructor.
Step 5 - Use method as per requirement.
Step 6 - Use this collection in your program as needed.
Example
is:Example
import java.util.ArrayList; public class Main { public static void main(String[] args) { ArrayList<String> names = new ArrayList<String>(); names.add("Apoorva"); names.add("Anamika"); names.add("Supriya"); System.out.println("Names: " + names); names.remove(1); names.set(1, "Neha"); System.out.println("Names: " + names); for (String name : names) { System.out.println("Name: " + name); } } }
Output
Names: [Apoorva, Anamika, Supriya] Names: [Apoorva, Neha, Supriya] Name: Apoorva Name: Neha Name: Supriya
Thus, using the Java collection framework can overcome the shortcomings or limitations of arrays. So we need a collection framework. The advantages of this framework are as follows -
Scalable nature of collections − Now, due to the scalable nature of collections, size is no longer an issue and we can increase or decrease the size at runtime.
Collections are memory efficient - Elements can be increased or decreased as per requirement, so from a memory perspective, it is recommended to use collections.
Built-in methods available in collections− Collections have many built-in methods to perform common operations such as add, search, etc.
Collections hold homogeneous and heterogeneous data elements - Collections can hold elements of the same as well as different types.
Standard Data Structures - Collections are based on standard data structures, so each collection supports ready-made methods.
in conclusion
Obviously, both arrays and collections have unique advantages and disadvantages. The specific requirements of your program are critical in deciding between them. Arrays prove to be more suitable in situations where the data size is predetermined and fast access to elements is required. Collections are better suited when you need a more flexible data structure and need built-in methods to manipulate the data.
The above is the detailed content of Why do we need to use collections framework in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










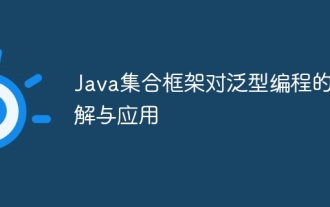
The Java collection framework applies generic programming, allowing the creation of reusable code that is independent of data types. By specifying type parameters, you can create type-safe collections and prevent type errors: Generics allow type parameterization, which is specified when creating a class or method and replaced by the actual type at compile time. Collection frameworks make extensive use of generics such as ArrayList, LinkedList, and HashMap. The benefits of generic collections include type safety, flexibility, and readability. In practice, generics can prevent type errors, such as ensuring that a grade list contains only integer types.
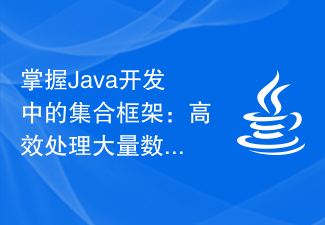
With the rapid development of information technology, big data has become an important resource in today's society. In the process of processing large amounts of data, efficient data structures and algorithms are crucial. The collection framework in Java development is a very powerful and efficient data processing tool. This article will explore how to make full use of the collection framework in Java development to efficiently process large amounts of data. First, we need to understand what a collection framework is. Collection framework is an API in Java used to store and manipulate data. It provides a series of data structures
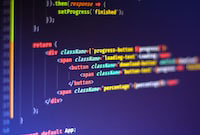
Overview of Java Collection Framework Java Collection Framework is a collection used to store and manipulate data. It provides various data structures, such as lists, sets, maps, etc. The main purpose of the collections framework is to provide a unified interface to access and manipulate these data structures, thereby simplifying programming. Underlying Principles of Collections Framework To understand the collections framework, you need to understand its underlying principles. Collection framework uses arrays and linked lists as its basic data structures. An array is a contiguous memory space that stores data elements of the same type. A linked list is a dynamic data structure composed of nodes, each node stores a data element and a pointer to the next node. Collections framework implements various data structures by using these basic data structures. For example, a list can be created using
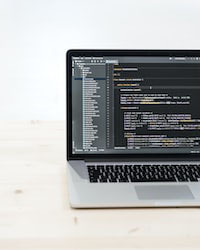
Overview of Java Collection Framework The Java collection framework is a large and complex system that contains a variety of collection containers. These containers can be classified based on the type of data they store, how they are accessed, thread safety, and other characteristics. In general, the Java collection framework mainly contains the following types of collection containers: List (List): List is one of the most common data structures, which allows you to store and access data in order. Elements in the list can be accessed by index, and elements can be added, removed, and modified. Stack: The stack is a last-in-first-out (LIFO) data structure. This means that elements added later will be removed first. The stack is typically used to store temporary data or function calls. Queue:
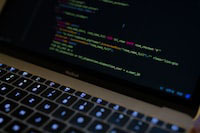
1. Tutorial for beginners: Java Collection Framework Tutorial This is a must-have guide for Java collection framework learners. This tutorial comprehensively introduces the Java collection framework, including collections, lists, mappings, stacks, queues, etc., and provides a large number of sample codes to help you understand. . Java Collections Framework Tutorial (GeeksforGeeks) GeeksforGeeks' Java Collections Framework Tutorial is another high-quality learning resource that provides in-depth explanations and plenty of sample code to help you quickly master the Java Collections Framework. Java Collection Framework Tutorial (javatpoint) javatpoint's Java Collection Framework Tutorial explains the Java collection framework in a concise and easy-to-understand language and provides
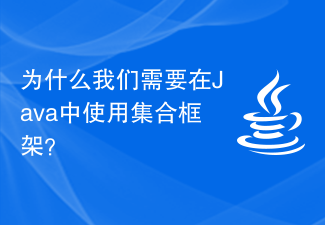
An array is a collection of elements of the same type stored in contiguous memory allocations. They are used to represent lists of things like numbers, strings, etc. Syntax<element-type>[]<array-name>=new<element-type>[<array-size>]; Algorithm To implement an array, please follow the steps below Step 1 − Careful consideration should be given in advance to fill all The appropriate data type needs to be selected for each individual element of the array. Step 2 − Additionally, determining the required capacity by considering specific usage requirements will enable the selection of an accurate and optimal array size. Step 3−Declare an array variable. Step 4 - Visit
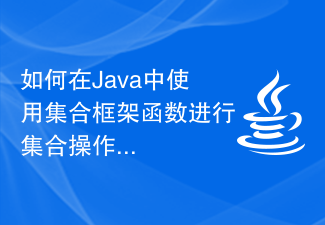
How to use collection framework functions for collection operations in Java Collections are a commonly used data structure in Java programming. They provide a convenient way to store and operate a set of objects. Java's collection framework provides a wealth of functions to operate on collections, including addition, deletion, modification, search, sorting, filtering, etc. Below we will introduce some commonly used set operation functions and give specific code examples. Traversing a collection In Java, we can use the following method to traverse a collection: //Create a List collection List<
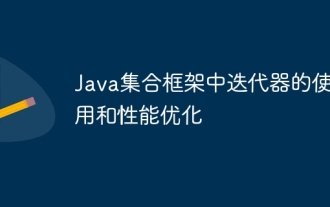
Use Fail-fast iterators and apply the following optimization techniques to improve the performance of iterators in the Java collection framework: Avoid iterating the same collection multiple times Minimize the number of times to create iterators Use parallel iterations to prefetch elements Avoid removing elements during iterations Consider Using cursors
