


C++ user interface and interactive function practice in embedded system development
C User interface and interactive function practice in embedded system development
Embedded systems play an important role in modern life, from home appliances to cars, From smart watches to industrial controls, various embedded devices have requirements for user interface and interactive functions. As a widely used high-level programming language, C has flexibility, portability and performance advantages, and has become the preferred language in embedded system development. This article will introduce how to use C to implement user interface and interactive functions in embedded system development, and provide practical demonstrations through code examples.
1. User interface design
The user interface design of embedded systems should focus on user experience and usability, while taking into account the hardware resource limitations of the device. C provides a variety of interface design libraries and frameworks, such as Qt, wxWidgets, etc. Developers can choose the appropriate library for development according to project needs. The following takes Qt as an example to introduce how to use C to implement user interface design for embedded systems.
In Qt, the interface can be designed through the QML language. QML is a declarative language based on JavaScript that can easily describe the user interface and interaction logic. The following is a simple QML code example that implements a user interface containing text and buttons:
import QtQuick 2.0 Rectangle { width: 200 height: 200 Text { anchors.centerIn: parent text: "Hello World" font.pixelSize: 20 } Button { anchors.horizontalCenter: parent.horizontalCenter anchors.bottom: parent.bottom text: "Click Me" onClicked: { console.log("Button clicked") } } }
Through the above code, we define a rectangular control and place a text control in the center of the rectangle with text content For "Hello World", the font size is 20 pixels. At the same time, we place a button in the lower part of the rectangle, display "Click Me" on the button, and print log information when the button is clicked.
Through the above examples, we can see that using C language combined with QML can easily realize the design of user interface. You only need to describe the hierarchy, style, layout and interaction logic of the control in the QML file, and then Simply load this file into your application via C code.
2. Realization of interactive functions
In addition to user interface design, embedded systems also need to implement rich interactive functions. In C, various libraries and frameworks can be used to implement these functions, such as serial communication, network communication, sensor data collection, etc. Below, we will introduce how to use C to implement serial communication through a code example.
In embedded system development, serial communication is a common interaction method. C provides a variety of libraries for serial communication, such as Boost.Asio and Qt SerialPort. The following is a sample code that uses the Qt SerialPort library to implement serial port communication:
#include <QtSerialPort/QSerialPort> #include <QCoreApplication> #include <QDebug> int main(int argc, char *argv[]) { QCoreApplication a(argc, argv); QSerialPort serial; serial.setPortName("/dev/ttyUSB0"); serial.setBaudRate(QSerialPort::Baud9600); serial.setDataBits(QSerialPort::Data8); serial.setParity(QSerialPort::NoParity); serial.setStopBits(QSerialPort::OneStop); if (serial.open(QIODevice::ReadWrite)) { qDebug() << "Serial port opened successfully"; qDebug() << "Sending data..."; serial.write("Hello World"); qDebug() << "Data sent"; serial.close(); } else { qDebug() << "Failed to open serial port"; } return a.exec(); }
Through the above code, we create a QSerialPort object and set the parameters of the serial port, such as device name, baud rate, data bits, calibration check position and stop position. Then we try to open the serial port. If the opening is successful, we write a piece of data "Hello World" to the serial port, and then close the serial port. If the opening fails, an error message is printed.
Through the above examples, we can see that the serial port communication function can be easily implemented using C language combined with the Qt SerialPort library. You only need to set the serial port parameters and then call the corresponding function. Developers can implement other interactive functions in a similar manner based on specific project needs.
Summary:
This article introduces how to use C to implement user interface and interactive functions in embedded system development. By choosing appropriate interface design libraries and frameworks, developers can easily implement user interface design. At the same time, by leveraging various libraries and frameworks, such as the serial communication library, developers can implement rich interactive functions. As a flexible, portable and high-performance programming language, C has broad application prospects in embedded system development.
However, it should be noted that in actual development, developers need to carefully consider the hardware resource limitations and performance requirements of the embedded system, select appropriate libraries and frameworks, and conduct sufficient testing and optimization to Ensure system stability and reliability. I hope this article will be helpful to readers in using C to implement user interface and interactive functions in embedded system development.
The above is the detailed content of C++ user interface and interactive function practice in embedded system development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
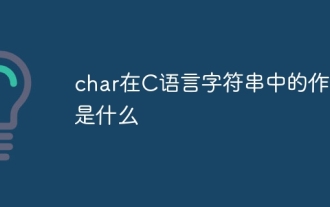
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
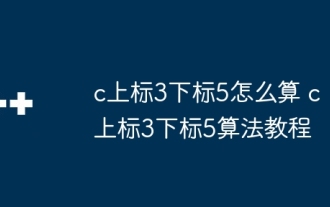
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
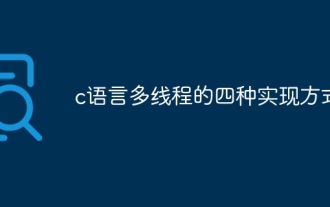
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
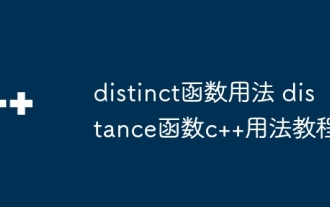
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
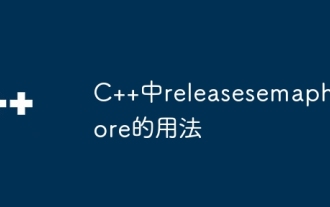
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
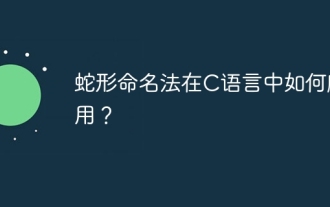
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
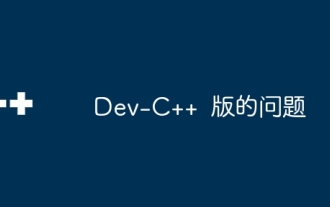
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
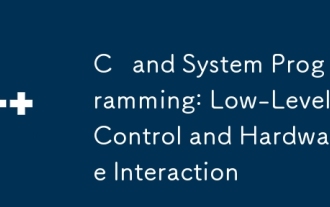
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
