


Comprehensive interpretation of PHP writing specifications: key elements of standardized development
Comprehensive interpretation of PHP writing specifications: key elements of standardized development
1. Introduction
In the software development process, good coding specifications can improve the reliability of the code. Readability, maintainability and scalability, reducing errors and debugging time. In PHP development, there is also a set of widely accepted writing specifications. This article will comprehensively interpret the PHP writing specifications to help developers standardize development and improve coding efficiency.
2. Naming specifications
- File name: Use lowercase letters and underscores (snake_case) to name, for example: user_model.php.
- Class name: Use big camel case naming method (PascalCase), with the first letter capitalized, for example: UserModel.
- Method name: Use camelCase naming method (camelCase), with the first letter lowercase, for example: getUserName().
- Function name: Use lowercase letters and underscores (snake_case) to name, for example: get_user_name().
- Variable name: Use lowercase letters and underscores (snake_case) to name, for example: user_name.
- Constant name: Use uppercase letters and underscores (SNAKE_CASE) to name, for example: MAX_LENGTH.
Sample code:
<?php class UserModel { public function getUserName() { $user_name = "John Doe"; return $user_name; } public function get_user_name() { $user_name = "John Doe"; return $user_name; } const MAX_LENGTH = 100; } ?>
3. Code style
- Indentation: Use 4 spaces for indentation and no tabs.
- Line break: Each line should not exceed 80 characters. If it exceeds, line break should be performed. When wrapping lines in function call arguments, use 4 spaces for indentation.
- Braces: The left brace starts on a new line, and the right brace goes with the code.
- Space: Use spaces on both sides of the operator and after the comma. Do not use spaces when calling functions. Do not use spaces between keywords and parentheses.
Sample code:
<?php class UserModel { public function getUserName() { $user_name = "John Doe"; return $user_name; } public function getUserByEmail($email) { if (strlen($email) > self::MAX_LENGTH) { return false; } return true; } public function saveUser($user_name, $email) { // 代码逻辑 } } ?>
4. Comment specifications
- Single-line comments: Use // for comments, with two spaces between the comments and the code.
- Multi-line comments: Use / comment /, and the start and end of the comment will occupy one line.
- Documentation comments: Use /* comments / to describe classes, methods, and properties in detail.
Sample code:
<?php class UserModel { /** * 获取用户姓名 * * @return string 用户姓名 */ public function getUserName() { $user_name = "John Doe"; return $user_name; } /** * 根据邮箱判断是否为有效用户 * * @param string $email 用户邮箱 * @return bool 是否为有效用户 */ public function getUserByEmail($email) { if (strlen($email) > self::MAX_LENGTH) { return false; } return true; } /** * 保存用户信息 * * @param string $user_name 用户姓名 * @param string $email 用户邮箱 * @return void */ public function saveUser($user_name, $email) { // 代码逻辑 } } ?>
5. Error handling
- Exception handling: Use try-catch blocks in the code for exception handling, which can be better Catch and handle exceptions effectively.
- Error reporting: Turn on error reporting in the development environment to display error and warning information, while turn off error reporting in the production environment and only record error logs.
- Error log: Use appropriate logging tools to record error information for subsequent analysis and processing.
Sample code:
<?php try { // 代码逻辑 } catch (Exception $e) { // 异常处理逻辑 } // 错误报告配置 ini_set('display_errors', 1); error_reporting(E_ALL); // 错误日志记录 error_log("Error message", 3, "/var/log/php_error.log"); ?>
6. Summary
Standard coding style and naming convention can improve the readability and maintainability of the code, and use comments to clearly explain the code Functions and usage methods make it easier for other developers to understand and use the code. Error handling is an important part of ensuring system stability. Reasonable error handling methods can improve system reliability. In PHP development, writing code according to specifications is a key element of standardized development, which facilitates teamwork and code maintenance. I hope this article will help you understand and comply with PHP writing standards.
The above is the detailed content of Comprehensive interpretation of PHP writing specifications: key elements of standardized development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










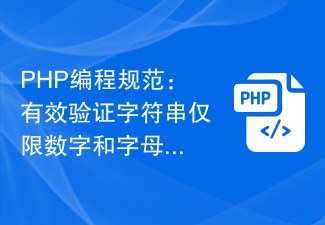
Programming disciplines are crucial to ensure code quality and maintainability, especially when developing PHP applications. One of the common requirements is efficient validation of input strings to ensure that they contain only numeric and alphabetic characters. This article will introduce how to write code in PHP to achieve this requirement while following programming conventions. Overview of Programming Standards In PHP programming, following certain programming standards can make the code easier to read and maintain, while helping to reduce errors and improve code performance. Here are some programming guideline recommendations: Use intentional
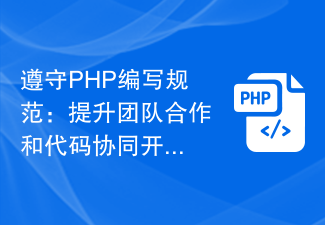
Comply with PHP writing specifications: Improve teamwork and code collaborative development capabilities Introduction: In software development, code quality and teamwork are crucial. Complying with programming standards is one of the effective means to improve code quality and teamwork. This article will focus on how to comply with PHP writing standards to improve teamwork and code collaborative development capabilities. 1. Naming conventions Good naming conventions can increase the readability and maintainability of code. In PHP programming, we recommend following the following naming convention: Use camelCase naming for variables and functions, such as
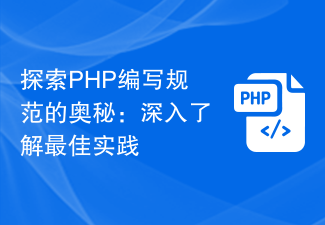
Explore the secrets of PHP writing specifications: In-depth understanding of best practices Introduction: PHP is a programming language widely used in web development. Its flexibility and convenience allow developers to use it widely in projects. However, due to the characteristics of the PHP language and the diversity of programming styles, the readability and maintainability of the code are inconsistent. In order to solve this problem, it is crucial to develop PHP writing standards. This article will delve into the mysteries of PHP writing disciplines and provide some best practice code examples. 1. Naming conventions compiled in PHP
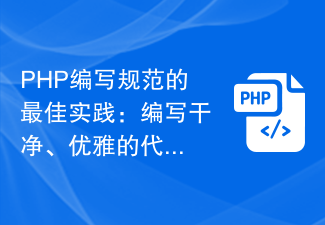
Best practices for PHP writing specifications: Write clean and elegant code Introduction: In PHP development, writing clean and elegant code is the key to improving code quality and maintainability. This article will explore several best practices to help developers write high-quality PHP code, thereby improving the maintainability and readability of the project. 1. Unified coding standards In a project, the coding styles of different developers may vary greatly, which is a huge challenge to the readability and maintainability of the code. Therefore, it is very important to develop and adhere to unified coding standards.
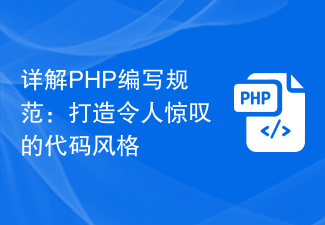
Detailed explanation of PHP writing specifications: Create amazing coding style Introduction: In the field of software development, excellent coding style is a programmer's advantage. PHP is a commonly used programming language. Good writing standards can improve the readability, maintainability and collaboration of the code. This article will introduce PHP writing specifications in detail to help you create an amazing coding style. 1. Naming specifications 1.1 Naming variables and functions Variables and functions should use meaningful and clear names, using a combination of lowercase letters and underscores. Variable names should use camelCase
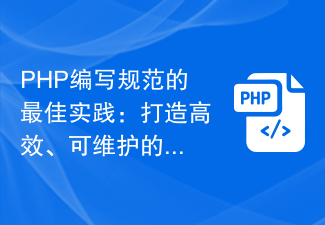
Best practices for writing specifications in PHP: Creating an efficient and maintainable code base Introduction: With the rapid development of Internet technology, PHP has become one of the most popular development languages. As a flexible scripting language, PHP has unparalleled advantages in building dynamic websites and web applications. However, if we don’t follow some PHP coding best practices, our codebase can become unmaintainable, unstable, and inefficient. This article will introduce some noteworthy PHP coding standards to help developers create efficient
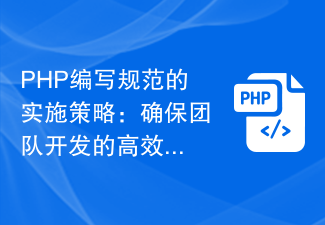
Implementation strategies for PHP writing specifications: ensuring high efficiency of team development In today's software development field, team collaboration has become the norm. In order to ensure high efficiency of team development, writing specifications has become an essential link. This article will introduce the implementation strategy of PHP writing specifications, with code examples to help the development team better understand and apply these specifications. Using consistent naming conventions is one of the important factors in code readability and maintainability. Team members should agree on consistent naming rules to ensure code consistency and readability
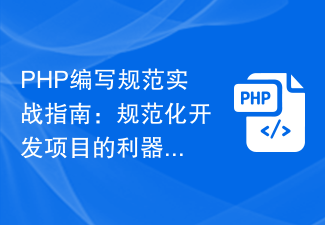
A Practical Guide to Writing Specifications in PHP: A Sharp Tool for Standardizing Development Projects Introduction: In a team collaboration development environment, writing specifications is very important. Not only does it improve code readability and maintainability, it also reduces the occurrence of errors and conflicts. This article will introduce some practical guidelines for writing specifications in PHP and demonstrate specific specifications through code examples. 1. Naming convention: Use camel case naming for class names, method names, and attribute names, with the first letter lowercase. Constant names are in all capital letters, and multiple words are separated by underscores. Use variable names that are meaningful and
