


A practical guide to writing specifications in PHP: a powerful tool for standardized development projects
Practical Guide to Writing Specifications in PHP: A Sharp Tool for Standardizing Development Projects
Introduction:
In a team collaboration development environment, writing specifications is very important. Not only does it improve code readability and maintainability, it also reduces the occurrence of errors and conflicts. This article will introduce some practical guidelines for writing specifications in PHP and demonstrate specific specifications through code examples.
1. Naming convention:
- Use camel case naming for class names, method names, and attribute names, with the first letter lowercase.
- Constant names are all in capital letters, and multiple words are separated by underscores.
- Use variable names that are meaningful and express their purpose.
Sample code:
class myClass { private $myVariable; public function myMethod($myParameter) { // code... } const MY_CONSTANT = 1; }
2. Indentation and spaces:
- Use four spaces for indentation and no tabs.
- Add spaces before and after operators, and add appropriate spaces to the code to improve readability.
Sample code:
function myFunction($var1, $var2) { $result = $var1 + $var2; if ($result > 0) { // code... } for ($i = 0; $i < $result; $i++) { // code... } }
3. Comment specifications:
- Use English to write comments.
- Longer comments are limited to 80 characters per line.
- Use comments to explain the function, principle, and variable meaning of the code.
Sample code:
/** * 计算两个数的和 * * @param int $var1 第一个数 * @param int $var2 第二个数 * @return int 两个数的和 */ function sum($var1, $var2) { return $var1 + $var2; }
4. Function and method specifications:
- Functions and methods should be kept as simple as possible, and each function and method only needs to be completed one thing.
- Avoid using global variables and try to use parameter passing and return values to interact with data.
Sample code:
// 不推荐的写法 function calculateSum() { global $var1, $var2; return $var1 + $var2; } // 推荐的写法 function calculateSum($var1, $var2) { return $var1 + $var2; }
5. Error handling specifications:
- Appropriately handle possible errors in the code and provide error messages .
- Use try-catch blocks to catch and handle exceptions.
Sample code:
try { // code... } catch (Exception $e) { echo '错误消息:' . $e->getMessage(); }
6. Other specifications:
- The code should be appropriately segmented and commented to increase the readability of the code.
- Functions and methods longer than 100 lines should be considered for splitting.
Conclusion:
Through the introduction of this article, we have learned some practical guidelines for PHP writing specifications, including naming specifications, indentation and spaces, comment specifications, function and method specifications, and error handling specifications. etc. Following these norms can improve team development efficiency and reduce the occurrence of errors and conflicts. Therefore, standardized development projects are a powerful tool that we should carefully abide by during the development process.
References:
[1] PHP Programming Specification, https://psr.ren/php
[2] PHP Programming Specification Guide, https://www.php-fig.org /psr/psr-12/
The above is the detailed content of A practical guide to writing specifications in PHP: a powerful tool for standardized development projects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










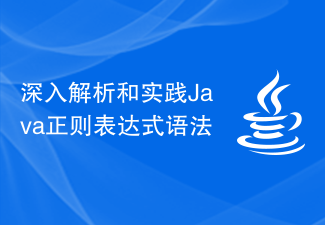
Java regular expression syntax detailed explanation and practical guide Introduction: Regular expression is a powerful text processing tool that can match, find and replace strings through a specific grammar rule. In the Java programming language, regular expressions can be used through the classes provided by the Java.util.regex package. This article will introduce the syntax of Java regular expressions in detail and provide practical code examples. 1. Basic syntax: 1. Single character matching: -Character class: expressed by square brackets [], indicating from the character sequence
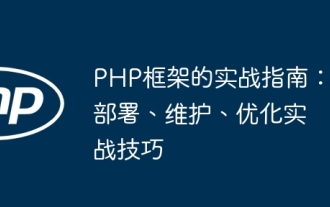
In order to fully utilize the potential of the PHP framework, this article provides practical tips, including: Deployment: choosing the appropriate environment, using version control, and automating deployment. Maintenance: Regularly check for updates, performance monitoring, and security patches. Optimization: Implement caching, code analysis, and optimize database queries. By following these best practices, you can ensure that your PHP applications run at peak performance and remain secure at all times.
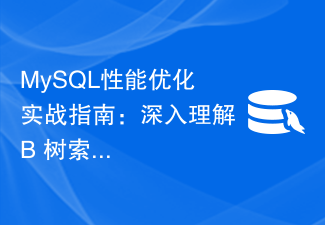
MySQL Performance Optimization Practical Guide: In-depth Understanding of B+ Tree Indexes Introduction: As an open source relational database management system, MySQL is widely used in various fields. However, as the amount of data continues to increase and query requirements become more complex, MySQL's performance problems are becoming more and more prominent. Among them, the design and use of indexes are one of the key factors affecting MySQL performance. This article will introduce the principle of B+ tree index and show how to optimize the performance of MySQL with actual code examples. 1. Principle of B+ tree index B+ tree is a
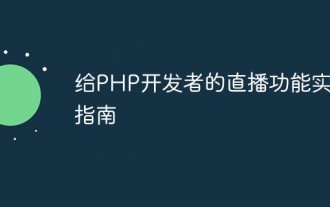
PHP is currently one of the most popular languages in website development. Its openness, flexibility and high customizability make it the development language of choice for many companies, organizations and individuals. In today's digital era, promoting products and services through live broadcast technology has become a very popular marketing method. This article will introduce live broadcast technology to PHP developers and provide some practical guidelines to help them quickly build an efficient live broadcast platform. First introduction to live broadcast technology Live broadcast technology refers to the transmission and playback of real-time audio and video data through the Internet
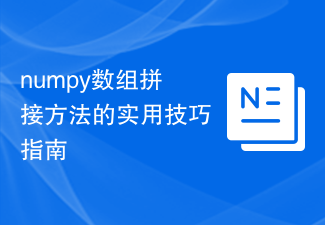
Practical guide: How to flexibly use the numpy array splicing method Introduction: In the process of data analysis and scientific calculation, we often need to splice arrays to achieve the combination and integration of data. Numpy is an important scientific computing library in Python. It provides a wealth of array operation functions, including a variety of array splicing methods. This article will introduce several commonly used Numpy array splicing methods and give specific code examples to help readers master their usage skills. 1. vstack and hstackv
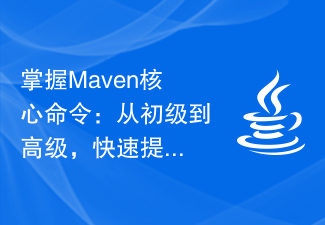
Maven Command Practical Guide: From entry to proficiency, master core commands and improve development efficiency. Introduction: As Java developers, we often use Maven, a powerful project construction tool. It can not only manage project dependencies, but also help us complete a series of tasks such as compilation, testing, and packaging. This article will introduce Maven's core commands and their usage examples to help readers better understand and apply Maven and improve development efficiency. 1. Maven’s core command mvncompil

Core principles of PHP writing specifications: ensuring code readability and maintainability Summary: In PHP development, writing standardized code is very important. Good coding style can improve the readability and maintainability of code and reduce the occurrence of errors. This article will introduce some core principles of PHP writing specifications and give corresponding code examples. Code indentation and line width: Code indentation can increase the readability of the code. It is recommended to use 4 spaces as the indentation standard. It is generally recommended that the line width should not exceed 80 characters. If it exceeds, line wrapping can be performed. Example
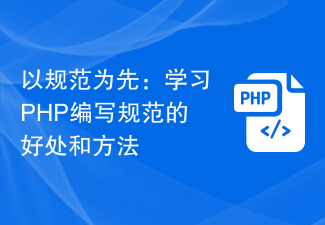
Put specifications first: The benefits and methods of learning PHP writing specifications 1. Introduction Programming specifications, as one of the basic qualities necessary for programmers, play an important role in ensuring code quality, readability, maintainability, etc. For PHP programmers, learning and complying with PHP writing specifications is an important step to improve their own abilities and improve the efficiency of team collaboration. This article will discuss the benefits of learning PHP writing standards and provide methods and examples. 2. The benefits of learning PHP writing standards to improve code quality: standardized coding habits can reduce errors and omissions
