


The average of the set bit counts in a given binary string after all possible K operations
In this problem, we need to find the average of the set bit count after performing all selected K operations on the given string.
Brute force methods can be used to solve the problem, but we will use probability principles to overcome the time complexity of brute force methods.
Problem Statement - We are given an integer N, an array arr[] containing K positive integers, and a binary string of length N containing only set bits. We need to find the average of the set bit count after performing all possible K operations. In the i-th operation, we can flip any arr[i] bit in the given string.
Example
Input– N = 2, arr[] = {1, 2}
Output– 1
Description – The initial binary string is 11.
In the first step, we can flip the first character and the string will be 01.
In the second operation, we need to flip any two bits. So the string will become 10.
The second selection can start by flipping the second character from the first step and the string will be 10.
In the second step of the current operation, we need to flip any 2 bits, and the string can be 01.
So, we have two choices, the final string can be 01 or 10.
Total selections = 2, total set bits in final string = 2, ans = 2/2 = 1.
Input– N = 3, arr[] = {2, 2}
Output– 1.6667
Explanation – We have an initial string which is 111.
In the first operation, we can flip any 2 characters. So the string could be 001, 100, 010.
In the second operation, we can flip 2 bits in the resulting string from the first operation.
When we flip any two bits of 001, we get 111, 010 and 100.
When we flip any 2 digits of 100, we can get 010, 111 and 001.
When we flip any two bits of 010, we can get 100, 001 and 111.
So, in the last operation, we got a total of 9 different strings.
The total number of setting digits in 9 strings=15, the total number of operations=9, the answer=15/9=1.6667
method one
Here, we will use the principle of probability to solve this problem. Suppose that after performing i-1 operations, the average value of the set bits is p and the average value of the unset bits is q. We need to calculate the average of the set bits and unset bits in the ith operation.
So, the updated value of p can be the average of the new set bits of p - the average of the new closed bits.
algorithm
Initialize P to N because we initially have N set bits, and initialize Q to 0 because we initially have 0 set bits.
Traverse the operation array.
Initialize prev_p and prev_q using P and Q values.
Update the P value using prev_p - prev_p * arr[i] / N prev_q * arr[i] / N, which will average the inverted bits to the set bits and average the set bits Invert to unset bits
Update Q value.
Return P value.
Example
is:Example
#include <bits/stdc++.h> using namespace std; double getAverageBits(int len, int K, int array[]) { // to store the average '1's in the binary string double P = len; // to store the average '0's in the binary string double Q = 0; // Traverse the array array[] for (int i = 0; i < K; i++) { // Initialize the prev_p and prev_q with P and Q, which we got from the previous iteration double prev_p = P, prev_q = Q; // Update the average '1's P = prev_p - prev_p * array[i] / len + prev_q * array[i] / len; // Update the average '0's Q = prev_q - prev_q * array[i] / len + prev_p * array[i] / len; } return P; } int main() { int N = 2; int array[] = {1}; int K = sizeof(array) / sizeof(array[0]); cout << "The average number of set bits after performing the operations is " << getAverageBits(N, K, array); return 0; }
Output
The average number of set bits after performing the operations is 1
Time complexity - O(K), where K is the length of the array.
Space Complexity - O(1) since we are not using any extra space.
In this tutorial we learned to find the average set bit after performing all possible choices of K operations. In single selection we need to perform all the operations given in the array.
The above is the detailed content of The average of the set bit counts in a given binary string after all possible K operations. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
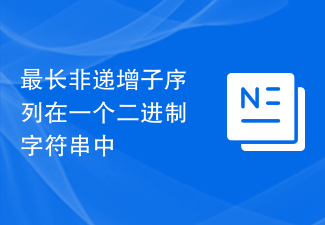
In this problem, we need to find the longest non-increasing subsequence of a given string. Non-increasing means that the characters are either the same or in descending order. Since binary strings only contain "0" and "1", the resulting string should either start with "1" and end with "0", or start and end with "0" or "1". To solve this problem, we will count the prefix "1" and suffix "0" at each position of the string and find the maximum sum of prefix "1" and suffix "0". Problem Statement - We are given a binary string str. We need to find the longest non-increasing subsequence from the given string. Example Input–str="010100"Output–4 illustrates the longest non-recursive
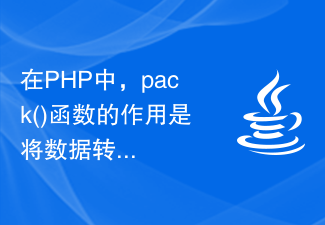
The pack() function packs data into a binary string. Syntax pack(format,args) Parameters format - the format to use. The following are possible values - a - NUL padded string A - space padded string h - hexadecimal string, low nibble first H - hexadecimal string, high nibble first c - signed char C - unsigned char s - signed short (always 16 bits, machine byte order) S - unsigned short (always 16 bits, machine byte order) n - unsigned short (always 16 bits, big endian byte order) v - unsigned short (always 16 bits, little endian byte order) i - signed integer (depends on machine size and byte order) I - None signed integer (depending on
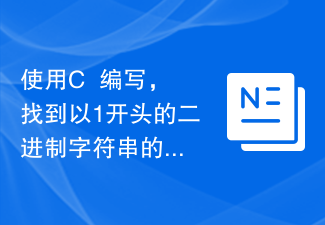
In the given problem, we are given a string consisting of 0 and 1; we need to find the total number of all permutations starting with 1. Since the answer may be a huge number, we take it modulo 1000000007 and output it. Input:str="10101001001"Output:210Input:str="101110011"Output:56 We will solve this problem by applying some combinatorial mathematics and setting up some formulas. Solution Method In this method we will count the number of 0's and 1's. Now suppose n is the number of 1's that appear in our string and m is the number of 0's that appear in our string
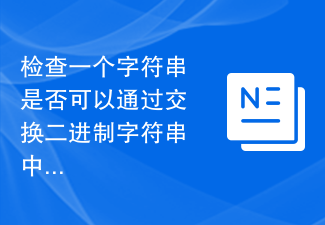
Problem Statement We have a string str and a binary string B. The length of both strings is equal to N. We need to check if we can make string str a palindrome string by swapping its characters multiple times on any pair of indexes containing unequal characters in string B. Example Example Input str=‘AAS’ B=‘101’ Output ‘YES’ The Chinese translation of Explanation is: Explanation We can exchange str[1] and str[2] because B[1] and B[2] are not equal. The final string can be 'ASA'. Input str=‘AASS’ B=‘1111’ and output ‘No’. The Chinese translation of Explanation is: Explanation that we cannot make the string palindrome,
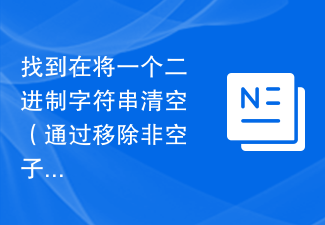
In this article, we will discuss an interesting problem related to the field of string manipulation and game theory: "Empty a binary string by removing non-empty substrings and find the player with the fewest remaining zeros". This question explores the concept of using binary strings for competitive gaming. Our goal is to find the player with the fewest 0 remaining at the end of the game. We will discuss this issue, provide a C++ code implementation, and explain the concept through an example. Understanding the problem statement Two players are given a binary string and they take turns playing the game. On each turn, the player removes non-empty substrings that contain at least one "1". The game ends when the string becomes empty or there is no "1" in the string. Players unable to take action lose the game. The task is to find the final 0
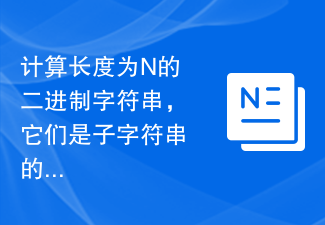
The purpose of this article is to implement a program that counts the number of binary strings of length N formed by repeated concatenation of a substring. The goal is to determine how many binary strings of length N can be created by repeatedly concatenating individual substrings of the given text, where N is a positive integer. Problem Statement Implement a program that counts the number of binary strings of length N that repeatedly concatenate substrings. Example Example 1 Letus take the Input, N = 3 Output: 2 The Chinese translation of Explanation is: Explanation The following lists feasible binary strings of length N = 3, in which a substring is repeatedly concatenated. "000":Thesubstr
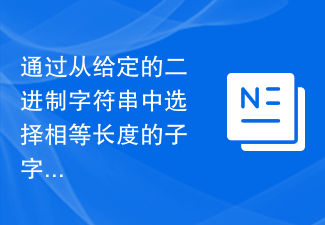
Given two binary strings str1 and str2 of the same length, we have to maximize the given function value by selecting substrings from the given strings of the same length. The given function is like this - fun(str1,str2)=(len(substring))/(2^xor(sub1,sub2)). Here, len(substring) is the length of the first substring and xor(sub1,sub2) is the XOR of the given substring, this is possible since they are binary strings. Example Input1:stringstr1=10110&stringstr2=11101Output:3 illustrates our
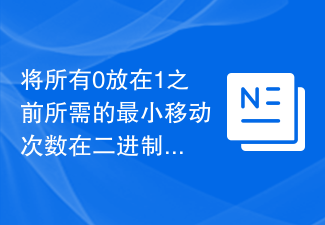
Problem Statement We are given a binary string str and we are required to remove minimum characters from the string so that we can place all zeros before ones. Example input str=‘00110100111’ Output 3 Description Here, we can achieve output 3 in two ways. We can remove arr[2], arr[3] and arr[5] or arr[4], arr[6] and arr[7] from the string. Input str=‘001101011’ and output 2 shows that we can delete arr[4] and arr[6] and put all zeros before 1. Input str=‘000111’ Output 0 means that in the given string, all zeros have been placed before 1, so we don’t need to start from
