Using template technology in C++
Using template technology in C
C is a very popular programming language with powerful functions and flexibility. One of the most important features is template technology, which allows programmers to define common data types and functions to adapt to various needs and scenarios.
1. The basic concept of templates
Template is a mechanism for code expansion at compile time. We can use templates to parameterize types when writing code so that the code can be applied to different types. By using templates, we can avoid repeatedly writing multiple similar codes and improve the reusability and maintainability of the code.
In C, templates can be used to define two things: function templates and class templates. Their syntax is basically the same, but their uses are slightly different. For example, the following is the definition of a simple function template:
template<typename T> T Max(T x, T y) { return (x > y ? x : y); }
In this example, we define a function template Max, use the keyword template to indicate that this is a template, and in <> Specify the type parameters we want. typename T here indicates that T is a type parameter.
2. Usage of function template
When we want to use the Max function in a program, we can pass different types of parameters. For example, it can be used like this:
int a = 1, b = 2; double c = 1.2, d = 3.4; cout << Max(a, b) << endl; cout << Max(c, d) << endl;
In this example, we use the Max function to calculate the maximum value of two integers and the maximum value of two floating point numbers. The C compiler will automatically expand these calls into corresponding functions at compile time.
In addition to using template parameters to indicate the type, we can also use other parameters. For example, we can use an integer parameter to determine the number of digits to compare (if we want to compare the lower 4 bits of two integers, rather than the entire integer):
template<typename T> T MaxBits(T x, T y, int numbits) { T mask = (1 << numbits) - 1; x &= mask; y &= mask; return (x > y ? x : y); } int x = 0x1234, y = 0x9876; cout << hex << MaxBits(x, y, 4) << endl;
3. Usage of class templates
In addition to function templates, C also allows us to define class templates. A class template is also a type of class, which can use template parameters as member data types. For example, the following is the definition of a stack class template:
template<typename T> class Stack { public: void Push(const T& value) { data_.push_back(value); } void Pop() { data_.pop_back(); } T& Top() { return data_.back(); } const T& Top() const { return data_.back(); } bool Empty() const { return data_.empty(); } private: std::vector<T> data_; };
In this example, we define a template class Stack that uses the template parameter T as the element type. We can use the Stack class like this:
Stack<int> stack1; stack1.Push(1); stack1.Push(2); stack1.Push(3); cout << stack1.Top() << endl; stack1.Pop(); cout << stack1.Top() << endl; Stack<string> stack2; stack2.Push("Hello"); stack2.Push("World"); cout << stack2.Top() << endl; stack2.Pop(); cout << stack2.Top() << endl;
In this example, we create two Stack instances, one for storing integers and the other for storing strings. By using templates, we can easily create common data structures that work for many different types of data.
4. Things to note about templates
When using templates, there are several things to note:
- The code of the template must be in the header file. Due to the special nature of templates, the compiler needs to instantiate the template when using it. If we assign the template code into a .cpp file, it may cause multiple definition errors and other issues.
- The instantiation of templates comes at a cost. Because the compiler must compile for every template instance used, using too many templates can result in long compilation times. It is recommended to control the scope of template usage during development to avoid excessive use of templates, which may lead to longer compilation times.
- Template error messages can be difficult to understand. Because the compilation process of templates is much more complicated than ordinary code, you may encounter some difficult-to-understand error messages when using templates. It is recommended to debug with caution when using templates and to read error messages carefully.
In short, templates are a very powerful mechanism in C programming. Using templates can greatly improve the reusability and maintainability of code, allowing us to write code more efficiently. I hope this article can help readers better understand and use template technology in C.
The above is the detailed content of Using template technology in C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
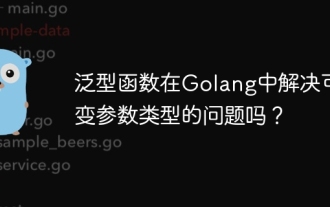
Generic functions in Go solve the problem of variadic types: generic functions allow type parameters to be specified at runtime. This makes it possible to write functions that can handle parameters of different types. For example, the Max function is a generic function that accepts two comparable parameters and returns the larger value. By using generic functions, we can write more flexible and general code that can handle different types of parameters.
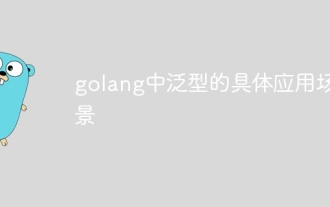
Application scenarios of generics in Go: Collection operations: Create collection operations suitable for any type, such as filtering. Data Structures: Write general-purpose data structures such as queues, stacks, and maps to store and manipulate various types of data. Algorithms: Write general-purpose algorithms such as sorting, search, and reduction that can handle different types of data.
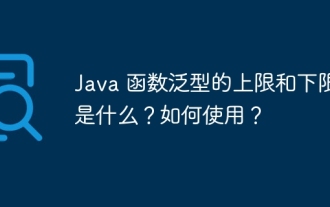
Java function generics allow setting upper and lower bounds. Extends specifies that the data type accepted or returned by a function must be a subtype of the specified type, e.g. The lower bound (super) specifies that the data type accepted or returned by a function must be a supertype of the specified type, e.g. The use of generics improves code reusability and security.
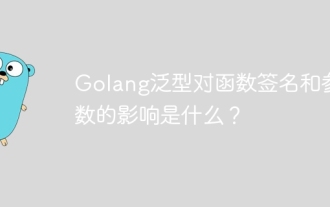
The impact of generics on Go function signatures and parameters includes: Type parameters: Function signatures can contain type parameters, specifying the types that the function can use. Type constraints: Type parameters can have constraints that specify conditions that they must satisfy. Parameter type inference: The compiler can infer the type of unspecified type parameters. Specifying types: Parameter types can be explicitly specified to call generic functions. This increases code reusability and flexibility, allowing you to write functions and types that can be used with multiple types.
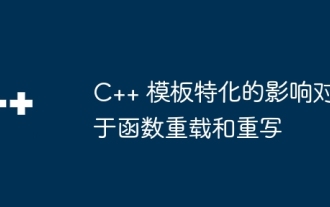
C++ template specializations affect function overloading and rewriting: Function overloading: Specialized versions can provide different implementations of a specific type, thus affecting the functions the compiler chooses to call. Function overriding: The specialized version in the derived class will override the template function in the base class, affecting the behavior of the derived class object when calling the function.
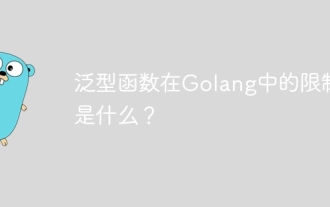
Limitations of Go generic functions: only type parameters are supported, value parameters are not supported. Function recursion is not supported. Type parameters cannot be specified explicitly, they are inferred by the compiler.
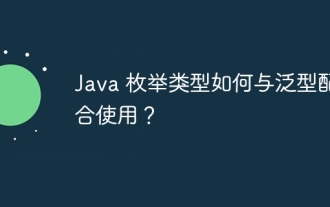
The combination of enumeration types and generics in Java: When declaring an enumeration with generics, you need to add angle brackets, and T is the type parameter. When creating a generic class, you also need to add angle brackets, T is a type parameter that can store any type. This combination improves code flexibility, type safety, and simplifies code.
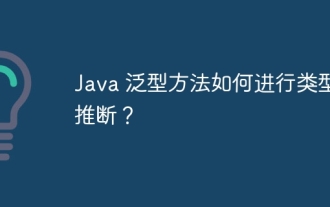
Java generic methods automatically infer type parameters without explicit declaration. Rules include: 1. Use explicit type declarations; 2. Infer a single type; 3. Infer wildcard types; 4. Infer constructor return value types. This simplifies the code, making it easier to write and use generic methods.
