


How to display statistical charts on a web page using PHP and Vue.js
How to use PHP and Vue.js to display statistical charts on a web page
Overview:
In modern web development, data visualization has become very important a part of. Statistical charts can visually display the trends and relationships of data, helping users better understand the data. This article mainly introduces how to use PHP and Vue.js to display statistical charts on web pages. Using PHP to process data and Vue.js to render charts, you can quickly and easily implement data visualization functions.
- Preparation
Before we start, we need to install PHP and Vue.js, and make sure you are familiar with basic PHP and Vue.js syntax. - Create database and table
First, we need to create a database and create a data table in it to store the data that needs to be displayed. You can use MySQL or other database management systems to accomplish this step.
CREATE DATABASE data_visualization; USE data_visualization; CREATE TABLE statistics ( id INT PRIMARY KEY AUTO_INCREMENT, date DATE, value INT );
The above is a simple data table structure, which contains an auto-incrementing id field, date field and numerical field.
- Connect to the database and get data
Next, we need to connect to the database and get data from the data table. We can accomplish this step using the MySQLi extension for PHP.
<?php $host = "localhost"; $username = "root"; $password = "password"; $dbname = "data_visualization"; $conn = new mysqli($host, $username, $password, $dbname); if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } $query = "SELECT * FROM statistics"; $result = $conn->query($query); $data = []; if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { $data[] = [ 'date' => $row['date'], 'value' => $row['value'] ]; } } $conn->close(); echo json_encode($data); ?>
The above code connects to the database, gets all the data from the data table, and returns it in JSON format.
- Use Vue.js to render the chart
Next, we need to use Vue.js to render the chart. We can use a third-party charting library to accomplish this step. Here we use Echarts to display a histogram.
First, introduce the library files of Vue.js and Echarts into HTML.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Data Visualization</title> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> <script src="https://cdn.jsdelivr.net/npm/echarts/dist/echarts.min.js"></script> </head> <body> <div id="app"> <div id="chart" style="width: 600px;height:400px;"></div> </div> <script> new Vue({ el: '#app', mounted() { this.loadData(); }, methods: { loadData() { // 发送Ajax请求获取数据 axios.get('data.php') .then(response => { this.renderChart(response.data); }) .catch(error => { console.log(error); }); }, renderChart(data) { // 使用Echarts绘制柱状图 var chart = echarts.init(document.getElementById('chart')); var dates = data.map(item => item.date); var values = data.map(item => item.value); var option = { xAxis: { data: dates }, yAxis: {}, series: [{ name: 'Value', type: 'bar', data: values }] }; chart.setOption(option); } } }); </script> </body> </html>
In Vue's mounted hook function, we call the loadData method to send an Ajax request, obtain data, and call the renderChart method to render the chart. In the renderChart method, we use Echarts to draw the histogram. The chart is rendered by extracting the date and value separately and then passing them to Echarts' drawing function.
- Run and display
Finally, the above code needs to be deployed to a PHP server and accessed through a browser. When the web page is accessed, data will be automatically obtained from the database and a histogram will be displayed.
Summary:
By using PHP and Vue.js, we can easily display statistical charts on the web page. The data is processed through PHP and returned to the front end in JSON format, and then Vue.js is used to render charts, which can quickly implement data visualization functions. The following is an example of using PHP and Vue.js to display statistical charts. I hope it will be helpful to you.
The above is the detailed content of How to display statistical charts on a web page using PHP and Vue.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
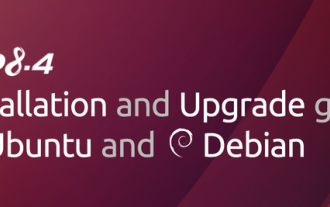
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
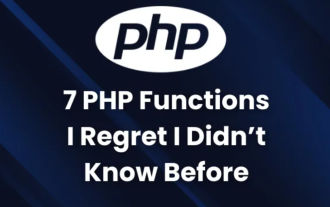
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
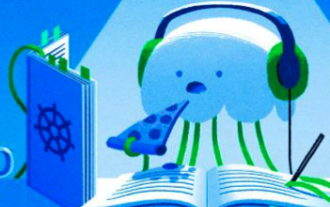
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
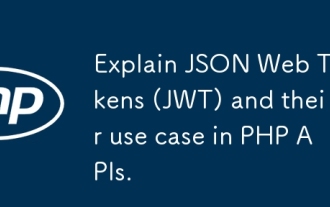
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
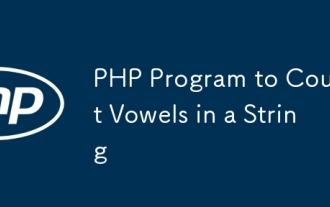
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
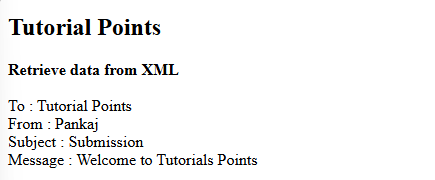
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
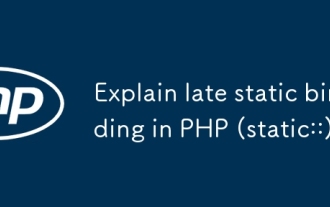
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
