Optimize the error handling mechanism of PHP applications
Optimizing the error handling mechanism of PHP applications
When developing PHP applications, error handling is a very important issue. A good error handling mechanism can improve the robustness and maintainability of the program. This article will introduce how to optimize error handling in PHP applications, helping developers better handle errors and provide a better user experience.
- Turn on error reporting and logging
First, we should ensure that PHP’s error reporting and logging functions are turned on. In this way, when an error occurs, we can receive timely warnings and record the details of the error.
In the PHP configuration file (php.ini), find the following two configuration items and set them to the following values:
error_reporting = E_ALL log_errors = On
Set error_reporting
to E_ALL
means turning on all error reports, including E_NOTICE and E_WARNING level errors. Setting log_errors
to On
means logging error information to the log file.
- Custom error handling function
Through the custom error handling function, we can convert standard PHP errors into our custom format and do further deal with.
The following is an example of a custom error handling function:
function customErrorHandler($errno, $errstr, $errfile, $errline) { // 根据错误级别分类处理 switch ($errno) { case E_ERROR: case E_USER_ERROR: // 处理致命错误 exit("致命错误:{$errstr} 在 {$errfile} 第 {$errline} 行"); break; case E_WARNING: case E_USER_WARNING: // 处理警告 echo "警告:{$errstr} 在 {$errfile} 第 {$errline} 行"; break; case E_NOTICE: case E_USER_NOTICE: // 处理注意 echo "注意:{$errstr} 在 {$errfile} 第 {$errline} 行"; break; default: // 处理其他错误 echo "未知错误:{$errstr} 在 {$errfile} 第 {$errline} 行"; break; } } // 设置错误处理函数 set_error_handler("customErrorHandler");
Set through the set_error_handler()
function and pass the error handling function customErrorHandler
enter. When an error occurs, this function will be automatically called for processing.
- Exception handling
In addition to handling PHP's standard error, we can also use exception handling to capture and handle exceptions in the program. Exception handling can better manage error information and provide a more reliable error handling mechanism.
The following is an example of simple exception handling:
try { // 代码块 // 可能会抛出异常的代码 } catch (Exception $e) { // 异常处理代码 echo "捕获到异常:".$e->getMessage(); }
In the above example, we use the try-catch
structure to wrap the code block that may throw an exception . When an exception is thrown, the program will automatically jump to the catch
block for exception handling.
- Error logging and information display
It is very important to record error information in the log file, which helps us locate and solve the problem. We can do this by writing error information to a log file.
function customErrorHandler($errno, $errstr, $errfile, $errline) { // 将错误信息写入日志文件 $logMessage = "错误:{$errstr} 在 {$errfile} 第 {$errline} 行"; error_log($logMessage, 3, "/path/to/log/file.log"); // 根据错误级别分类处理 // ... }
In the above example, we use the error_log()
function to write error information to the specified log file. Among them, parameter 1 is the error message, parameter 2 is the way to write the log file (usually 3, which means appending to the end of the file), and parameter 3 is the log file path.
In addition, we can also display error messages through the user interface so that users can understand and report problems. In the development environment, we can display error messages directly; in the production environment, we can display customized error pages or friendly prompts.
Summary
By optimizing the error handling mechanism of PHP applications, we can improve the robustness and maintainability of the program and improve the user experience. By enabling error reporting and logging, custom error handling functions, exception handling, and error logging and information display, we can better handle errors and quickly locate and solve problems.
Good error handling mechanism is an important part of a good PHP application. I believe that through the methods introduced in this article, developers can handle errors more efficiently when developing and maintaining PHP applications.
The above is the detailed content of Optimize the error handling mechanism of PHP applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
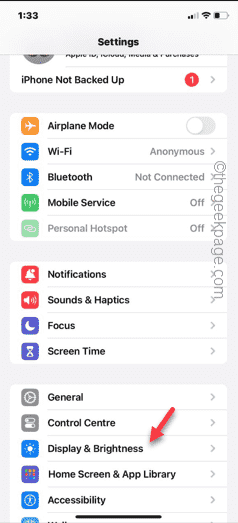
Having issues with the Shazam app on iPhone? Shazam helps you find songs by listening to them. However, if Shazam isn't working properly or doesn't recognize the song, you'll have to troubleshoot it manually. Repairing the Shazam app won't take long. So, without wasting any more time, follow the steps below to resolve issues with Shazam app. Fix 1 – Disable Bold Text Feature Bold text on iPhone may be the reason why Shazam is not working properly. Step 1 – You can only do this from your iPhone settings. So, open it. Step 2 – Next, open the “Display & Brightness” settings there. Step 3 – If you find that “Bold Text” is enabled
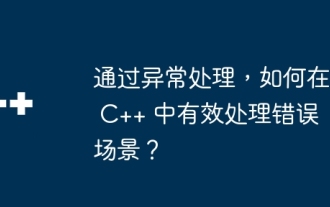
In C++, exception handling handles errors gracefully through try-catch blocks. Common exception types include runtime errors, logic errors, and out-of-bounds errors. Take file opening error handling as an example. When the program fails to open a file, it will throw an exception and print the error message and return the error code through the catch block, thereby handling the error without terminating the program. Exception handling provides advantages such as centralization of error handling, error propagation, and code robustness.
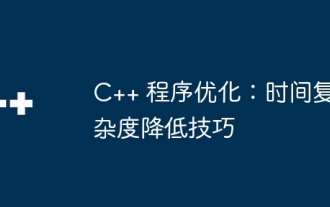
Time complexity measures the execution time of an algorithm relative to the size of the input. Tips for reducing the time complexity of C++ programs include: choosing appropriate containers (such as vector, list) to optimize data storage and management. Utilize efficient algorithms such as quick sort to reduce computation time. Eliminate multiple operations to reduce double counting. Use conditional branches to avoid unnecessary calculations. Optimize linear search by using faster algorithms such as binary search.
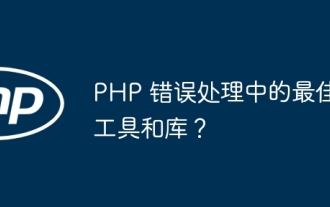
The best error handling tools and libraries in PHP include: Built-in methods: set_error_handler() and error_get_last() Third-party toolkits: Whoops (debugging and error formatting) Third-party services: Sentry (error reporting and monitoring) Third-party libraries: PHP-error-handler (custom error logging and stack traces) and Monolog (error logging handler)
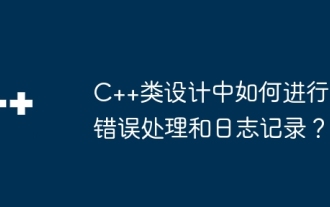
Error handling and logging in C++ class design include: Exception handling: catching and handling exceptions, using custom exception classes to provide specific error information. Error code: Use an integer or enumeration to represent the error condition and return it in the return value. Assertion: Verify pre- and post-conditions, and throw an exception if they are not met. C++ library logging: basic logging using std::cerr and std::clog. External logging libraries: Integrate third-party libraries for advanced features such as level filtering and log file rotation. Custom log class: Create your own log class, abstract the underlying mechanism, and provide a common interface to record different levels of information.
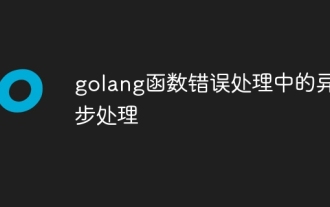
In Go functions, asynchronous error handling uses error channels to asynchronously pass errors from goroutines. The specific steps are as follows: Create an error channel. Start a goroutine to perform operations and send errors asynchronously. Use a select statement to receive errors from the channel. Handle errors asynchronously, such as printing or logging error messages. This approach improves the performance and scalability of concurrent code because error handling does not block the calling thread and execution can be canceled.
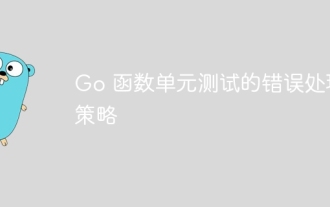
In Go function unit testing, there are two main strategies for error handling: 1. Represent the error as a specific value of the error type, which is used to assert the expected value; 2. Use channels to pass errors to the test function, which is suitable for testing concurrent code. In a practical case, the error value strategy is used to ensure that the function returns 0 for negative input.
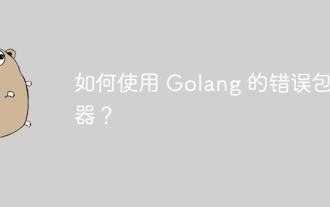
In Golang, error wrappers allow you to create new errors by appending contextual information to the original error. This can be used to unify the types of errors thrown by different libraries or components, simplifying debugging and error handling. The steps are as follows: Use the errors.Wrap function to wrap the original errors into new errors. The new error contains contextual information from the original error. Use fmt.Printf to output wrapped errors, providing more context and actionability. When handling different types of errors, use the errors.Wrap function to unify the error types.
