


How to use blockchain technology in Java to implement decentralized applications?
How to use blockchain technology in Java to implement decentralized applications?
As an emerging technology, blockchain is being increasingly used in various fields. In traditional centralized applications, data and power are concentrated on a central node, which is vulnerable to attacks and tampering. Blockchain technology makes data storage and interaction more secure and reliable through a decentralized approach. This article will introduce how to use blockchain technology in Java to implement decentralized applications and give specific code examples.
- Create the blockchain structure
First, we need to define the structure of the blockchain. A basic blockchain consists of several blocks, each containing some data and the hash of the previous block. The following is a simple Java class to represent a block:
public class Block { private String hash; private String previousHash; private String data; // 构造函数 public Block(String data, String previousHash) { this.data = data; this.previousHash = previousHash; this.hash = calculateHash(); } // 计算区块的哈希值 public String calculateHash() { // 使用SHA-256算法计算哈希值 // 省略具体实现 return null; } // 获取区块的哈希值 public String getHash() { return hash; } // 获取前一个区块的哈希值 public String getPreviousHash() { return previousHash; } // 获取区块的数据 public String getData() { return data; } }
- Create Blockchain
Next, we need to create a blockchain and implement some Basic operations such as adding blocks, verifying the integrity of the blockchain, etc. Here is a simple Java class to represent a blockchain:
import java.util.ArrayList; import java.util.List; public class Blockchain { private List<Block> chain; // 构造函数 public Blockchain() { chain = new ArrayList<>(); // 创建初始区块 Block genesisBlock = new Block("Genesis Block", "0"); chain.add(genesisBlock); } // 添加一个新区块 public void addBlock(Block newBlock) { newBlock.previousHash = getLatestBlock().getHash(); newBlock.hash = newBlock.calculateHash(); chain.add(newBlock); } // 获取链中最后一个区块 public Block getLatestBlock() { return chain.get(chain.size() - 1); } // 验证区块链的完整性 public boolean isValid() { for (int i = 1; i < chain.size(); i++) { Block currentBlock = chain.get(i); Block previousBlock = chain.get(i - 1); // 比较当前区块的哈希值和计算的哈希值是否一致 if (!currentBlock.getHash().equals(currentBlock.calculateHash())) { return false; } // 比较当前区块的前一个区块的哈希值和保存的哈希值是否一致 if (!currentBlock.getPreviousHash().equals(previousBlock.getHash())) { return false; } } return true; } }
- Testing the Blockchain
Now, we can test the blockchain we just created. The following is a simple test class to demonstrate how to use blockchain technology in Java to implement decentralized applications:
public class BlockchainTest { public static void main(String[] args) { // 创建一个新的区块链 Blockchain blockchain = new Blockchain(); // 添加一些新的区块 blockchain.addBlock(new Block("Block 1", blockchain.getLatestBlock().getHash())); blockchain.addBlock(new Block("Block 2", blockchain.getLatestBlock().getHash())); blockchain.addBlock(new Block("Block 3", blockchain.getLatestBlock().getHash())); // 输出区块链的完整性 System.out.println("Blockchain is valid: " + blockchain.isValid()); } }
The above code demonstrates how to use blockchain technology in Java to create A simple decentralized application. By defining the blockchain structure, implementing the functions of adding blocks and verifying the integrity of the blockchain, we can easily build a safe and reliable decentralized application.
It should be noted that the above code example is only a simplified implementation, and more factors need to be considered in actual applications, such as network communication, consensus algorithm, etc. In addition, more functions need to be added to the blockchain, such as finding specific blocks, querying transaction records of blocks, etc. I hope this article can provide you with some reference for using blockchain technology in Java to implement decentralized applications.
The above is the detailed content of How to use blockchain technology in Java to implement decentralized applications?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
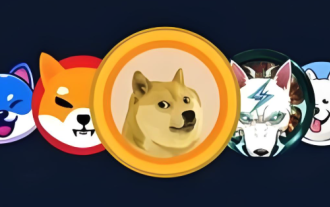
The most suitable platforms for trading Meme coins include: 1. Binance, the world's largest, with high liquidity and low handling fees; 2. OkX, an efficient trading engine, supporting a variety of Meme coins; 3. XBIT, decentralized, supporting cross-chain trading; 4. Redim (Solana DEX), low cost, combined with Serum order book; 5. PancakeSwap (BSC DEX), low transaction fees and fast speed; 6. Orca (Solana DEX), user experience optimization; 7. Coinbase, high security, suitable for beginners; 8. Huobi, well-known in Asia, rich trading pairs; 9. DEXRabbit, intelligent
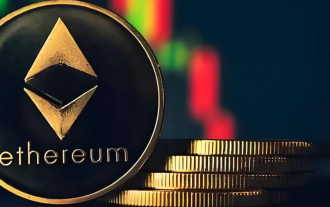
EU MiCA compliance certification, covering 50 fiat currency channels, cold storage ratio 95%, and zero security incident records. The US SEC licensed platform has convenient direct purchase of fiat currency, a ratio of 98% cold storage, institutional-level liquidity, supports large-scale OTC and custom orders, and multi-level clearing protection.
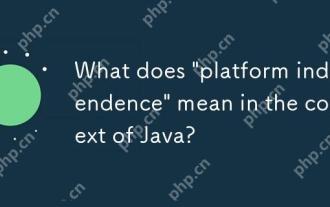
Java's platform independence means that the code written can run on any platform with JVM installed without modification. 1) Java source code is compiled into bytecode, 2) Bytecode is interpreted and executed by the JVM, 3) The JVM provides memory management and garbage collection functions to ensure that the program runs on different operating systems.

The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.
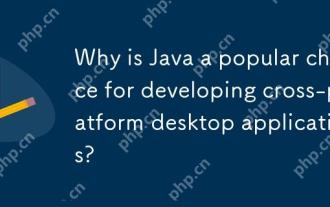
Javaispopularforcross-platformdesktopapplicationsduetoits"WriteOnce,RunAnywhere"philosophy.1)ItusesbytecodethatrunsonanyJVM-equippedplatform.2)LibrarieslikeSwingandJavaFXhelpcreatenative-lookingUIs.3)Itsextensivestandardlibrarysupportscompr
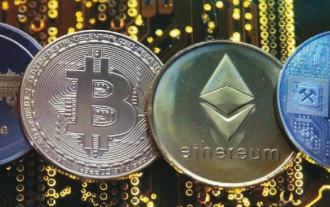
The world-renowned hybrid stablecoin trading platforms include: 1. Binance: the world's largest, supports a variety of stablecoins, comprehensive trading tools, and strong security measures. 2. OKX: It is a world-leading, efficient trading engine, and provides products such as multi-chain non-custodial wallets. 3. Bitget: Innovative order transactions, complete security agreements, and advantages in transaction handling fees. 4. Coinbase: The US platform, known for its compliance and security, is suitable for beginners and professional traders. 5. Huobi: well-known in Asia, strong technical strength, and compliant operations. 6. Kraken: Has a long history, good safety record, and strict compliance. 7. Bitfinex: a global platform, high liquidity, suitable for
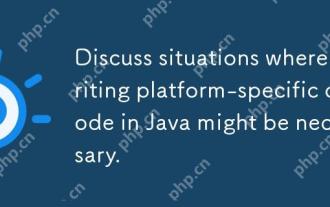
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
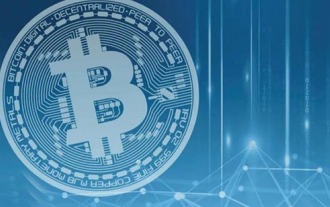
Virtual currency is a digital asset encrypted using cryptography technology, with its main features including decentralization, encryption security, global circulation and relative anonymity. Virtual currency has a wide range of application scenarios, including value storage, trading media, investment and financial management, DeFi, NFT, blockchain games, smart contracts, dApps and governance voting. Participating in virtual currency requires 1) Learn basic knowledge, 2) Select a trading platform, 3) Register and verify your identity, 4) Purchase virtual currency, 5) Safely store, 6) Participate in the community. Investments need to pay attention to risks such as price fluctuations, market, technology, supervision, fraud and information asymmetry.
